Introduction to MongoDB for Web Development
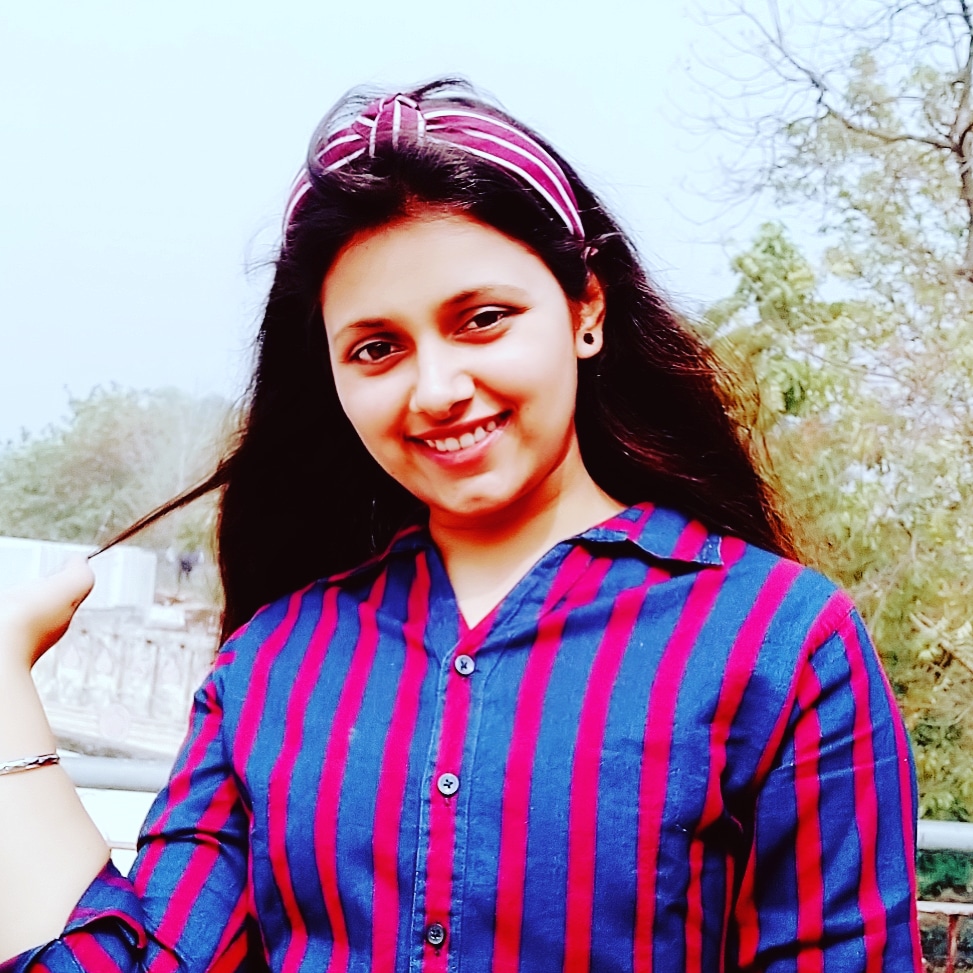

Introduction
MongoDB is a NoSQL database system that stores data in a flexible, JSON-like format called BSON (Binary JSON). Unlike SQL databases, which store data in tables with rows and columns, MongoDB organizes data in documents that are grouped in collections. This schema-less structure makes MongoDB particularly useful for web applications where data structures may evolve over time. In this lesson, we’ll cover MongoDB basics and practice creating and managing a simple movie application database.
Key Concepts and Definitions
Database: A container for collections in MongoDB. Each database holds multiple collections, similar to how a relational database holds tables.
Collection: A group of MongoDB documents, somewhat analogous to a table in an SQL database. Collections store documents with potentially diverse structures.
Document: The basic unit of data in MongoDB, stored as a BSON object. It typically represents a record with fields and values.
Example: For a movie app, we could have:
A database named
movieApp
.Collections such as
users
andmovies
.Documents within these collections representing individual users or movies.
Step-by-Step Explanation
1. Setting Up MongoDB and Creating a Database
Connect to MongoDB: Open MongoDB Compass (GUI) or use the MongoDB shell/CLI.
Create a Database:
use movieApp; // This creates a database named movieApp or switches to it if it already exists
2. Creating Collections in MongoDB
You can create a collection by simply inserting a document. MongoDB will create the collection automatically.
db.createCollection("users");
db.createCollection("movies");
3. Basic CRUD Operations on Documents
Create (Insert)
Adding documents to a collection in MongoDB:
Insert One Document:
db.users.insertOne({ username: "john_doe", email: "john@example.com" });
Insert Multiple Documents:
db.movies.insertMany([ { title: "Inception", genre: "Sci-Fi", year: 2010 }, { title: "The Matrix", genre: "Action", year: 1999 } ]);
Read (Query)
Retrieving data from a collection:
Find One Document:
db.movies.findOne({ title: "Inception" });
Find All Documents:
db.movies.find({});
Update
Modifying documents in a collection:
Update One Document:
db.users.updateOne( { username: "john_doe" }, { $set: { email: "john.new@example.com" } } );
Update Multiple Documents:
db.movies.updateMany( { genre: "Action" }, { $set: { genre: "Action/Adventure" } } );
Delete
Removing documents from a collection:
Delete One Document:
db.users.deleteOne({ username: "john_doe" });
Delete Multiple Documents:
db.movies.deleteMany({ year: { $lt: 2000 } });
4. Building a Movie App Database
In a movie application, we’ll define two collections: users
and movies
. Each collection will hold relevant documents, and we’ll set up basic CRUD operations.
Create
users
Collection and Add Documents:db.users.insertOne({ username: "jane_doe", email: "jane@example.com", favoriteMovies: ["Inception", "The Matrix"] });
Create
movies
Collection and Add Documents:db.movies.insertMany([ { title: "Avatar", genre: "Sci-Fi", year: 2009 }, { title: "Titanic", genre: "Drama", year: 1997 } ]);
Examples of CRUD Operations for the Movie App
Adding a New Movie:
db.movies.insertOne({ title: "Interstellar", genre: "Sci-Fi", year: 2014 });
Finding a User's Favorite Movies:
db.users.findOne({ username: "jane_doe" });
Updating a Movie's Information:
db.movies.updateOne( { title: "Avatar" }, { $set: { genre: "Science Fiction" } } );
Deleting a Movie Released Before a Certain Year:
db.movies.deleteMany({ year: { $lt: 2000 } });
Try it yourself
Create User Documents: Add three user documents with different favorite movies.
Query Movies: Find all movies with the genre “Sci-Fi.”
Update User Email: Update a user’s email address.
Delete a Movie: Delete a movie by title from the
movies
collection.
Subscribe to my newsletter
Read articles from Anjali Saini directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
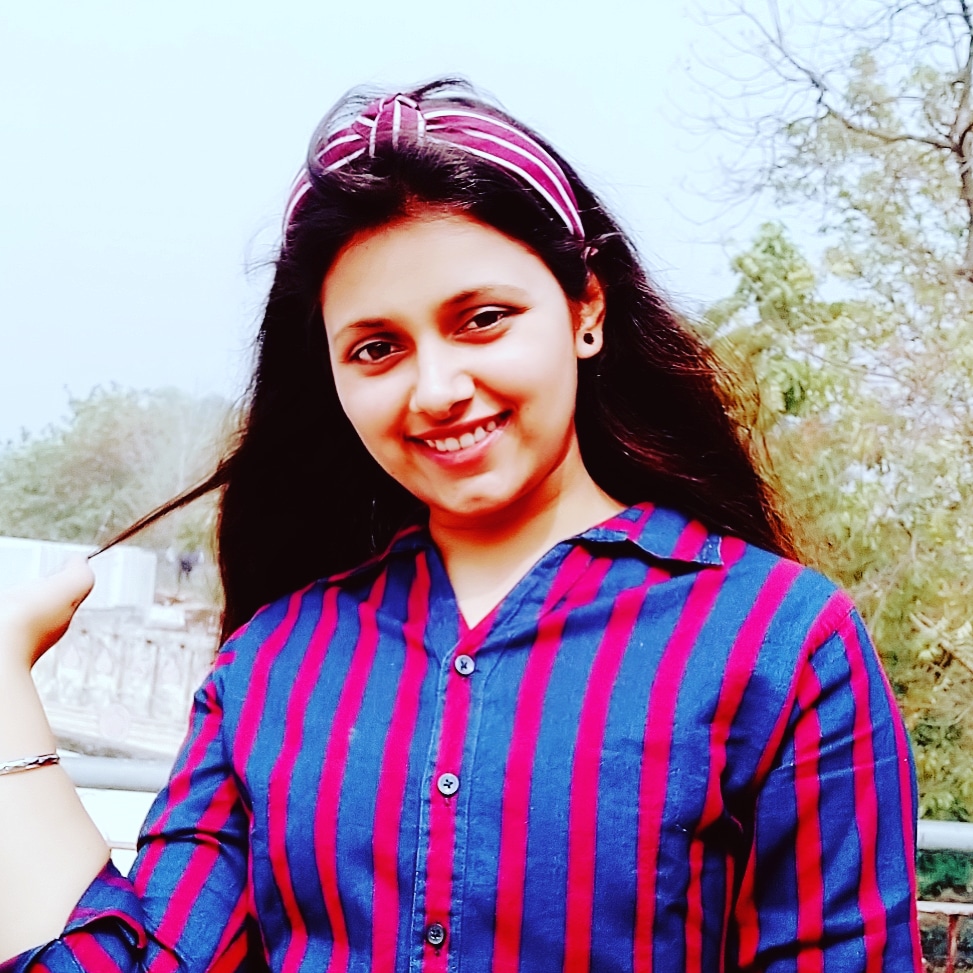
Anjali Saini
Anjali Saini
I am an Enthusiastic and self-motivated web-Developer . Currently i am learning to build end-to-end web-apps.