10 Most Essential JavaScript Array Methods (With Code Examples)
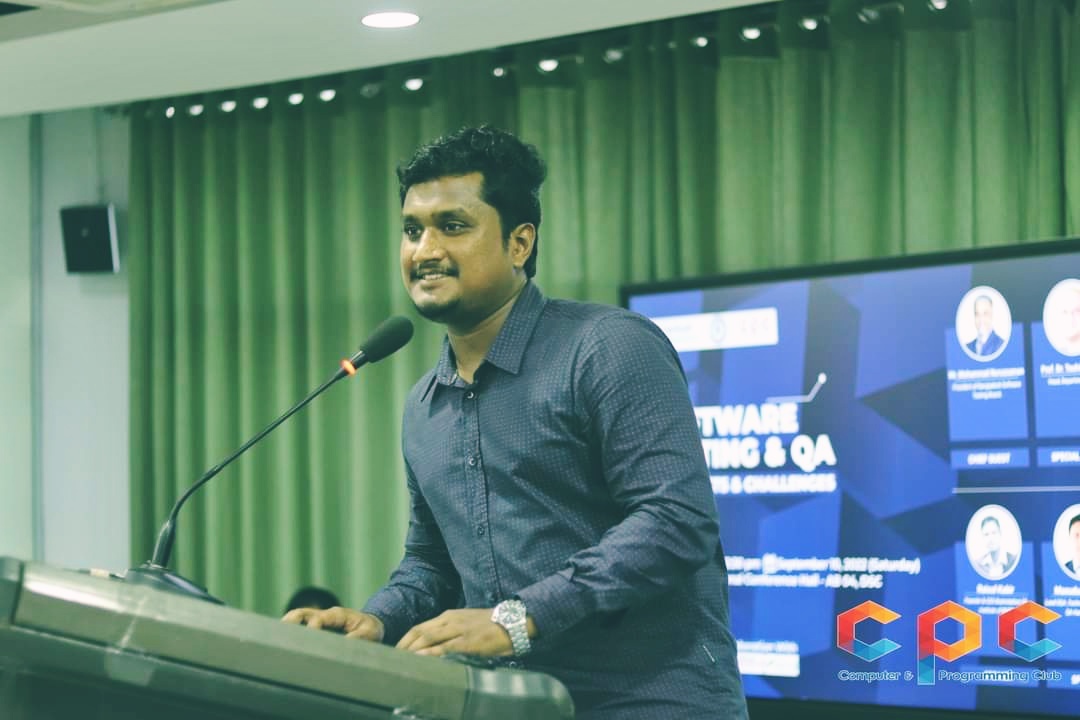

JavaScript arrays are incredibly versatile and come with many built-in methods that make manipulation a breeze. In this post, we’ll walk through 10 of the most important array functions every developer should know — complete with code examples and easy-to-understand explanations.
1. push()
— Add Elements to the End
The push()
method adds one or more elements to the end of an array. It also returns the new length of the array.
let lang = ["Java", "JS", "Python"];
lang.push("Ruby");
console.log(lang); // Output: ["Java", "JS", "Python", "Ruby"]
2. pop()
— Remove the Last Element
The pop()
method removes the last element from an array and returns it. It modifies the original array.
let lang1 = ["Java", "JS", "Python"];
lang1.pop();
console.log(lang1); // Output: ["Java", "JS"]
3. shift()
— Remove the First Element
The shift()
method removes the first element from an array and returns that removed element. It also updates the array’s length.
let fruits = ["apple", "banana", "orange", "mango"];
let firstFruit = fruits.shift();
console.log(firstFruit); // Output: "apple"
console.log(fruits); // Output: ["banana", "orange", "mango"]
4. unshift()
— Add Elements at the Beginning
The unshift()
method adds one or more elements to the beginning of an array. It returns the new length of the array.
let color = ['red', 'green'];
color.unshift('blue');
console.log(color); // Output: ['blue', 'red', 'green']
console.log(color.length); // Output: 3
5. splice()
— Add or Remove Elements at a Specific Index
The splice()
method can add, remove, or replace elements in an array. It modifies the original array.
let animals = ['bird', 'cow', 'cat', 'mouse'];
animals.splice(0, 1, 'bear'); // Remove 1 element at index 0 and add 'bear'
console.log(animals); // Output: ['bear', 'cow', 'cat', 'mouse']
6. slice()
— Extract a Portion of the Array
The slice()
method returns a shallow copy of a portion of an array without modifying the original array. The end index is not included.
let abc = [1, 2, 3, 4, 5, 6, 7];
let newArray = abc.slice(1, 4);
console.log(newArray); // Output: [2, 3, 4]
7. concat()
— Combine Two or More Arrays
The concat()
method merges two or more arrays and returns a new array.
let fr = ["apple", "banana", "orange", "mango"];
let nmbr = [1, 2, 3, 4];
let newConcatArray = fr.concat(nmbr);
console.log(newConcatArray);
// Output: ["apple", "banana", "orange", "mango", 1, 2, 3, 4]
8. indexOf()
— Find the Index of an Element
The indexOf()
method returns the first index at which a given element can be found. If the element is not found, it returns -1.
let anmls = ['bird', 'cow', 'cat', 'mouse', 'bird'];
let indx = anmls.indexOf("bird");
console.log(indx); // Output: 0
// Find second occurrence of 'bird'
let xyz = anmls.indexOf('bird', 1); // Start search from index 1
console.log(xyz); // Output: 4
9. includes()
— Check if an Element Exists
The includes()
method checks whether an array contains a certain element. It returns a boolean (true
or false
).
let test = ["admin", "super admin", "owner", "viewer"];
let flag = test.includes("admin");
console.log(flag); // Output: true
10. forEach()
— Loop Through the Array
The forEach()
method executes a provided function once for each array element.
let n = [1, 2, 3, 4, 5, 6, 7];
n.forEach((e) => {
console.log(e * 2); // Output: each element multiplied by 2
});
Conclusion
Mastering these basic yet powerful array methods is key to becoming proficient in JavaScript. They cover most of the array manipulation tasks you'll need in real-world coding scenarios.
Subscribe to my newsletter
Read articles from Md. Niaz Morshed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
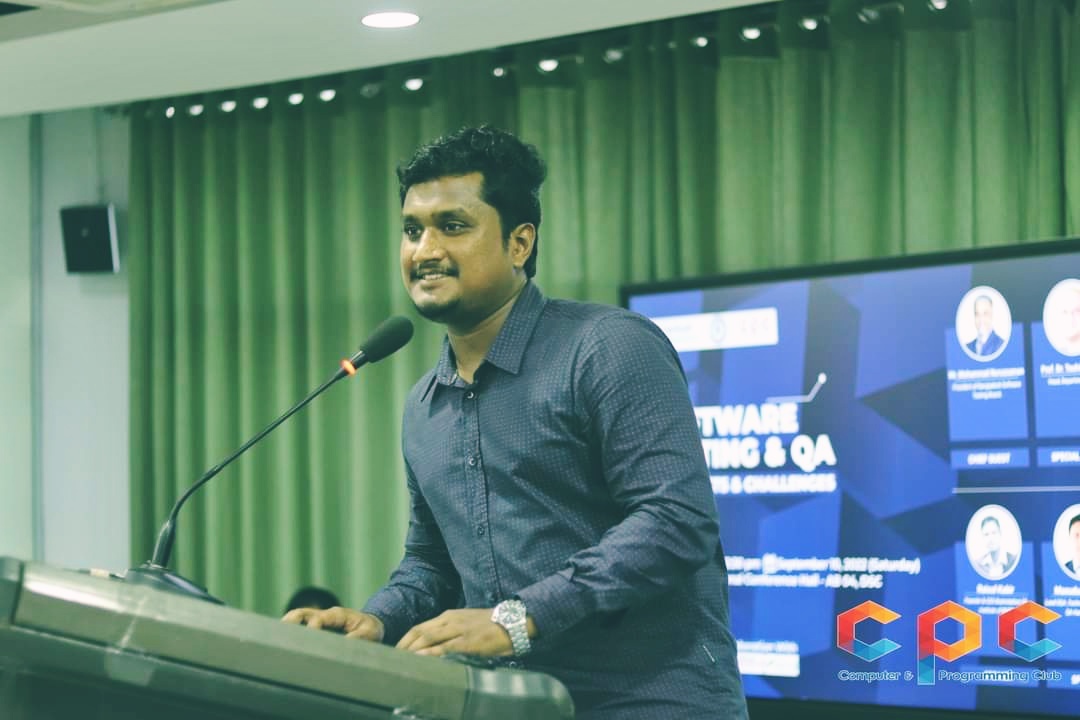
Md. Niaz Morshed
Md. Niaz Morshed
Software QA professional with the enthusiasm to learn more and spread knowledge as well as skills among others