Static Site Generation (SSG) vs. Server-Side Rendering (SSR) in Next.js
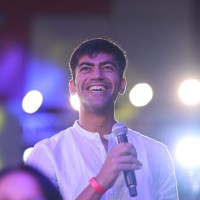
What is Next js ?
Next.js is a React framework for building full-stack web applications.
Rendering is one of the features of Next.js that is very useful for developer. In this blog post, we'll explore the key differences between SSG and SSR, their advantages and when to choose one over the other.
Two main rendering strategy of Next.js.
Static Site Generation (SSG)
Static site generation is a technique in web development where it pre-renders pages at build time. That means in production, the page HTML generated when you run next build. SSG results in excellent performance because this HTML will then be reused on each request. This pre-render pages are ready to serve directly to user on each request. This approach is ideal for websites with content that doesn’t change frequently, like blogs or documentation sites.
In Next js, you can statically generates pages with or without data. Lets take a look on example below.
Static Site Generation without data
Using Static Generation, it re-render without fetching data. Here's an example.
function About() {
return <div>About</div>
}
export default About
Note that this page does not need to fetch any external data to be pre-rendered. In cases like this, Next.js generates a single HTML file per page during build time.
Static generation with data
Next js provides two important function for fetching data during static generation.
getStaticProps: Fetches data for a specific page.
getStaticPaths: Defines dynamic routes for a page.
- getStaticProps.
To fetch this data on pre-render, Next.js allows you to export an async function called getStaticProps from the same file. This function gets called at build time and lets you pass fetched data to the page's props on pre-render.
export default function Blog({ posts }) {
// Render posts...
}
// This function gets called at build time
export async function getStaticProps() {
// Call an external API endpoint to get posts
const res = await fetch('https://.../posts')
const posts = await res.json()
// By returning { props: { posts } }, the Blog component
// will receive `posts` as a prop at build time
return {
props: {
posts,
},
}
}
- getStaticPath
Next.js allows you to create pages with dynamic routes. Next.js lets you export an async function called getStaticPaths from a dynamic page . This function gets called at build time and lets you specify which paths you want to pre-render.
// This function gets called at build time
export async function getStaticPaths() {
// Call an external API endpoint to get posts
const res = await fetch('https://.../posts')
const posts = await res.json()
// Get the paths we want to pre-render based on posts
const paths = posts.map((post) => ({
params: { id: post.id },
}))
// We'll pre-render only these paths at build time.
// { fallback: false } means other routes should 404.
return { paths, fallback: false }
}
Advantages of SSG
Speed: Pre-rendered HTML files are served directly, resulting in faster load times.
Scalability: Static files can be easily served by Content Delivery Networks (CDNs), improving your application’s ability to handle more global traffic around the world.
You can use Static Generation for many types of pages, including:
Marketing pages
Blog posts and portfolios
E-commerce product listings
Help and documentation
When should you use Static Generation?
If you want to re-render page ahead of a user's request, and if your content don't change often, then you must choose Static Generation for better performance and scalability.
Server-side Rendering (SSR)
Server-Side Rendering involves generating the HTML for each page on the server when a user requests it. It is also referred as a "Dynamic Rendering". With SSR, there is a server that pre-renders the page – it’s like a template that you plug variables into and the server handles all of the rendering. This happens at request time, so when the user requests the page, they get the server side rendered page. This rendering happens all on the server side and never runs in the browser.
If your page needs to pre-render frequently updated data (fetched from an external API). You can write getServerSideProps which fetches this data and passes it to Page like below.
export default function Page({ data }) {
// Render data...
}
// This gets called on every request
export async function getServerSideProps() {
// Fetch data from external API
const res = await fetch(`https://.../data`)
const data = await res.json()
// Pass data to the page via props
return { props: { data } }
}
SSR is ideal for websites with dynamic or personalised content that changes frequently. You can use Static Generation for many types of pages, including
E-commerce websites.
Media platforms.
Advantages of SSR
Consistent user experience: Users see the latest content as it’s generated on-the-fly, ensuring they always have up-to-date information.
Personalisation: SSR allows you to serve unique content based on user preferences or other dynamic data.
When you should use Server-Side Rendering.
Consider whether your users need real-time data or personalised content. If so, SSR is the better option. SSR pages will always display the latest data because they are generated on the server with every request from the user. With every request, you can always be sure that you have the most recent/up-to-date information.
Conclusion
In this article, we have discussed the two rendering approaches in Next.js: SSG and SSR. I have also provided an example codebase that will help you determine the best rendering approach for your application.
Resources
https://nextjs.org/docs/pages/building-your-application/rendering
Subscribe to my newsletter
Read articles from Piyush Nanwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
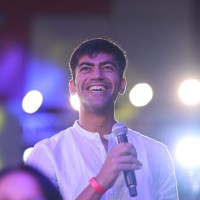
Piyush Nanwani
Piyush Nanwani
Experienced full-stack developer with a passion for tackling complex challenges. Dedicated to helping you become a top 1% mobile app developer. Passionate about mentoring, teaching, and continuous learning in new technologies.