Managing Concurrency with the StampedLock Class in Java
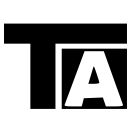
4 min read
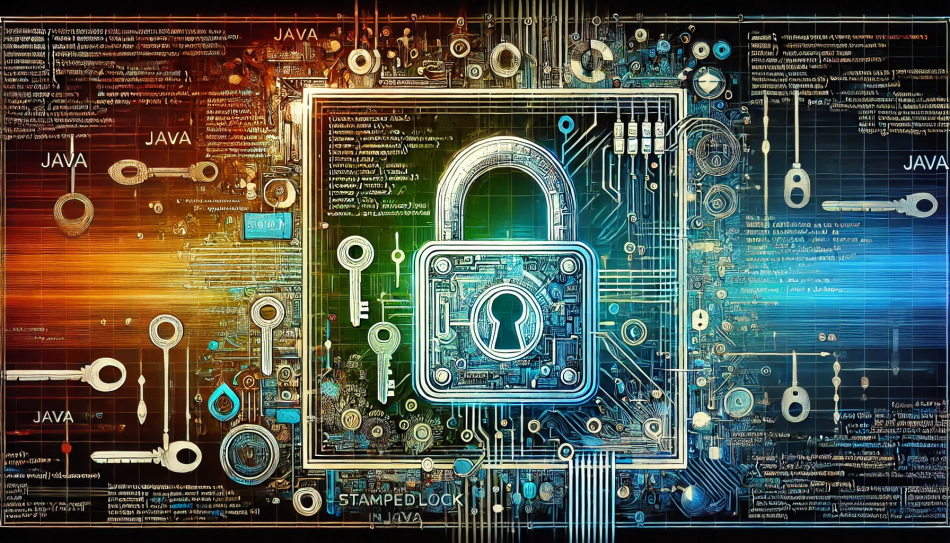
1. Understanding StampedLock in Java
1.1 What is StampedLock?
The StampedLock class was introduced in Java 8 to enhance performance in concurrent applications. Unlike traditional lock mechanisms, such as ReentrantLock, StampedLock provides three distinct locking modes:
- Write Lock: For exclusive access, similar to other locks.
- Read Lock: For shared access across multiple readers.
- Optimistic Read: A lightweight lock for scenarios with minimal risk of data changes.
1.2 How Does StampedLock Work?
At the core, StampedLock leverages stamps, or tokens, to manage locks. When a thread acquires a lock, it receives a unique stamp. The stamp can be used to release the lock or upgrade the lock, depending on the needs of the application.
1.3 Why Use StampedLock?
With StampedLock, Java developers can minimize blocking in read-heavy scenarios, as optimistic reads allow multiple threads to read without any locking overhead, provided that data is not modified by other threads. This capability makes StampedLock a powerful alternative for applications with high read-to-write ratios.
2. Using StampedLock in Java
2.1 Example Code for StampedLock
Let’s look at a basic example of StampedLock in action. Here’s how to implement it in a thread-safe counter:
import java.util.concurrent.locks.StampedLock;
public class ConcurrentCounter {
private int count = 0;
private final StampedLock lock = new StampedLock();
public void increment() {
long stamp = lock.writeLock();
try {
count++;
} finally {
lock.unlockWrite(stamp);
}
}
public int readCount() {
long stamp = lock.tryOptimisticRead();
int currentCount = count;
if (!lock.validate(stamp)) {
stamp = lock.readLock();
try {
currentCount = count;
} finally {
lock.unlockRead(stamp);
}
}
return currentCount;
}
}
2.2 Explanation of the Code
- Write Lock: The increment method uses a write lock to ensure exclusive access to the count variable.
- Optimistic Read: In readCount, we first attempt an optimistic read. This type of lock does not block other threads, making it highly efficient for read-heavy applications. If another thread writes to count while we are reading, lock.validate(stamp) will return false, and we fall back to acquiring a shared read lock.
3. Best Practices for StampedLock
3.1 Avoid Unnecessary Blocking
Whenever possible, use optimistic reads for read-heavy operations. This approach minimizes locking overhead and improves the performance of your application, particularly in cases where data modifications are infrequent.
3.2 Combine StampedLock with Other Concurrency Utilities
To build even more robust systems, consider pairing StampedLock with CompletableFuture or other Java concurrency utilities. For example, using StampedLock for fine-grained data access and CompletableFuture for async task management can help optimize both data safety and task efficiency.
3.3 Handle InterruptedException Carefully
When using StampedLock in a concurrent environment, handle InterruptedException properly to prevent potential resource leaks or incomplete tasks. Although StampedLock does not directly throw InterruptedException, it can arise when using other concurrent utilities together with StampedLock.
4. Common Issues with StampedLock
4.1 Starvation
StampedLock does not support fairness policies, meaning that threads might be starved if other threads continuously acquire locks. Avoid using StampedLock in scenarios where fairness is a critical requirement.
4.2 Performance Trade-offs
Although StampedLock can improve performance in read-heavy applications, it may not be as beneficial in write-heavy scenarios. In such cases, the overhead of acquiring and releasing stamps might outweigh the concurrency benefits. To achieve optimal results, use StampedLock in applications where reads are significantly more common than writes.
5. Conclusion
Using StampedLock can enhance the performance of Java applications that require efficient concurrency control. By leveraging optimistic reads and adopting best practices, you can reduce contention and improve throughput in read-heavy scenarios. If you have questions or would like to share your experiences with StampedLock, feel free to leave a comment below.
Read more at : Managing Concurrency with the StampedLock Class in Java
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
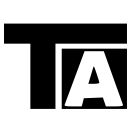
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.