Event Driven Programming and Its Core Concepts
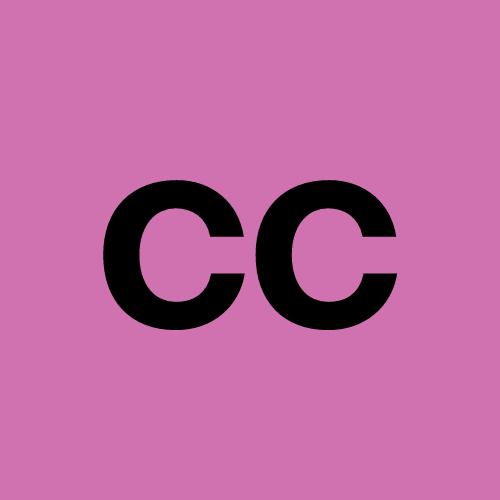

Event-driven programming is a software development paradigm centered around responding to events or actions initiated by users, sensors, or other systems. In contrast to traditional programming, event-driven programming emphasizes asynchronous behavior, enabling applications to manage multiple tasks effectively.
This programming approach has transformed modern software development by facilitating the creation of highly interactive and responsive applications. Event-driven programming is the backbone of technologies such as graphical user interfaces, real-time systems, and IoT devices, making it essential for delivering seamless user experiences.
Key Takeaways
Event-driven programming helps apps react to user actions or events.
It uses event sources, listeners, and handlers to manage tasks.
The event loop handles many tasks at once, keeping apps fast.
This method works well for big apps with changing workloads.
Examples include websites, smart devices, and games, showing its usefulness.
What Is Event Driven Programming?
Definition and Core Principles
Event-driven programming is a paradigm where the flow of a program is determined by events. These events can originate from user actions, system changes, or external inputs. Unlike traditional programming, which follows a sequential flow, event-driven programming reacts to specific triggers, making it highly dynamic and responsive.
At its core, event-driven programming relies on several key principles:
Event Sources: These are the origins of events, such as user interactions (e.g., button clicks) or system-generated signals.
Event Listeners: These components monitor event sources and wait for specific triggers.
Event Handlers: These are functions or methods that execute when an event occurs.
The Event Loop: This mechanism continuously checks for new events and ensures the program responds appropriately.
For example, in a graphical user interface (GUI), clicking a button generates an event. The event listener detects this action and triggers the corresponding event handler, which executes the desired functionality. This seamless interaction is a hallmark of event-driven programming.
Real-world applications of event-driven programming include logging systems, real-time notifications, and network request handling. For instance, a logging system processes logs and triggers alerts when specific conditions are met. Similarly, real-time notification services send alerts based on events in project management tools. These examples illustrate the versatility and practicality of event-driven programming in modern software development.
How It Differs from Traditional Programming Paradigms
Traditional programming paradigms, such as procedural or object-oriented programming, follow a linear and predefined sequence of instructions. In contrast, event-driven programming operates asynchronously, responding to events as they occur. This fundamental difference makes event-driven programming more suitable for applications requiring high interactivity and responsiveness.
Consider a network server as an example. In a traditional paradigm, the server processes requests sequentially, which can lead to delays if one request takes longer to complete. Event-driven programming, however, allows the server to handle multiple requests simultaneously by reacting to events. This approach minimizes latency and optimizes performance.
A well-documented technical paper highlights the benefits of event-driven architectures (EDAs) in multi-cloud and hybrid environments. It demonstrates how EDAs improve operational efficiency and system resilience by addressing challenges like data silos and latency. These findings underscore the advantages of event-driven programming in ensuring data consistency and optimizing performance.
Key Concepts of Event Driven Programming
Event Sources and Their Role
Event sources are the origins of events in event driven programming. These sources can include user actions, such as mouse clicks or keyboard inputs, as well as system-generated events like file changes or network requests. They act as the starting point for the entire event-driven process. Without event sources, there would be no triggers to initiate the flow of the program.
For example, in a web application, a button serves as an event source. When a user clicks the button, it generates an event that the program can respond to. Similarly, in IoT systems, sensors act as event sources by detecting changes in the environment, such as temperature or motion. These sources ensure that the program remains dynamic and responsive to external stimuli.
Event Listeners and Handlers
Event listeners and event handlers are critical components of event driven programming. Event listeners monitor event sources and wait for specific triggers. Once an event occurs, the corresponding event handler executes the required action. This mechanism ensures that the program responds appropriately to each event.
To optimize performance, developers often follow best practices when implementing event listeners and handlers:
Gather user feedback by using event listeners to capture ratings and comments.
Ensure scalability by optimizing event handling as user interactions increase.
Prioritize critical events to reduce lag and errors.
Streamline event scripts to minimize resource-intensive operations.
Keep data passed through events concise to improve processing time.
Regularly test and profile scripts to identify and address performance bottlenecks.
For instance, in a chat application, an event listener might detect when a user sends a message. The event handler then processes the message and displays it in the chat window. This seamless interaction highlights the importance of well-designed event listeners and handlers.
The Event Loop and Its Functionality
The event loop is the backbone of event driven programming. It continuously monitors the program for new events and ensures that they are processed in the correct order. This mechanism enables asynchronous operations, allowing the program to handle multiple tasks simultaneously without blocking the main thread.
In JavaScript, the event loop interacts with components like the Call Stack, Web APIs, Callback Queue, and Microtask Queue. It manages asynchronous tasks efficiently, ensuring that the program remains responsive. The following table provides an overview of the event loop's key functions:
Topic | Description |
Event Loop | Core mechanism enabling JavaScript to handle asynchronous operations efficiently. |
Interaction | Works with Call Stack, Web APIs, Callback Queue, and Microtask Queue. |
Optimization | Helps in writing non-blocking JavaScript code. |
For example, in a Node.js application, the event loop schedules and executes asynchronous tasks, such as reading files or handling HTTP requests. By avoiding blocking operations, the event loop ensures that the application remains responsive, even under heavy workloads.
How Event Driven Programming Works
Image Source: pexels
Step-by-Step Process
Event-driven programming operates through a structured process that ensures responsiveness and efficiency. The following steps outline how this paradigm works:
Event Source Generation: An event source, such as a user action or system signal, generates an event. For instance, a mouse click or a sensor detecting motion can act as triggers.
Event Detection: Event listeners monitor the event sources and detect when an event occurs. These listeners remain active throughout the program's lifecycle.
Event Dispatching: Once detected, the event is dispatched to the appropriate event handler. This step ensures that the correct function or method processes the event.
Event Handling: The event handler executes the required action. For example, it might update the user interface or send a notification.
Event Loop Management: The event loop continuously checks for new events and ensures they are processed in the correct order. This mechanism enables asynchronous operations, allowing the program to handle multiple tasks simultaneously.
The Event-driven process chain (EPC) provides a useful framework for understanding this flow. The table below summarizes its key aspects:
Aspect | Description |
Definition | An Event-driven process chain (EPC) is an ordered graph of events and functions. |
Purpose | Used for modeling business processes, particularly in event-driven programming mechanisms. |
Characteristics | Provides connectors for alternative and parallel execution of processes, specified by logical operators (OR, AND, XOR). |
Strengths | Simplicity and easy-to-understand notation, making it widely acceptable for denoting business processes. |
Execution Semantics | Requires non-local semantics, where the execution behavior of a node may depend on the state of other parts of the EPC, potentially far away. |
This structured approach ensures that event-driven programming remains efficient and adaptable to various use cases.
Example: Button Click in a GUI Application
A button click in a graphical user interface (GUI) application provides a simple yet effective example of event-driven programming.
Event Source: The button acts as the event source. When a user clicks the button, it generates an event.
Event Listener: The program includes an event listener that monitors the button for click events.
Event Dispatching: Upon detecting the click, the event listener dispatches the event to the corresponding handler.
Event Handling: The event handler executes the desired action, such as displaying a message or updating the interface.
For example, in JavaScript, the following code demonstrates this process:
// Event Listener for Button Click
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
In this example, the button with the ID "myButton" serves as the event source. The addEventListener
method attaches an event listener to the button. When the user clicks the button, the event handler displays an alert message. This simple interaction highlights the power of event-driven programming in creating responsive applications.
Benefits of Event Driven Programming
Enhanced Responsiveness and User Experience
Event-driven programming significantly enhances application responsiveness by enabling systems to react to events in real time. This approach ensures that applications remain interactive and efficient, even during high user activity. For instance, in a messaging app, users can send and receive messages instantly because the system processes events, such as message delivery, without delay.
By prioritizing responsiveness, event-driven architecture improves user experience. Applications feel more intuitive and dynamic, as they respond immediately to user actions like button clicks or form submissions. This responsiveness fosters user satisfaction and engagement, making event-driven programming a cornerstone for modern, user-centric software.
Scalability in Event-Driven Architecture
Event-driven architecture excels in building scalable applications. Its ability to handle fluctuating workloads makes it ideal for systems with unpredictable user interactions. For example, a streaming platform can process millions of concurrent requests by distributing events across multiple servers. This design ensures that the system remains operational and responsive, even during peak usage.
Scalability is achieved through loose coupling, where components operate independently. This independence allows developers to scale individual modules based on demand. For instance, a payment processing module in an e-commerce platform can scale separately from the product catalog. This modularity ensures efficient resource utilization and cost-effectiveness, making event-driven architecture a preferred choice for scalable applications.
Modularity and Flexibility in System Design
Event-driven architecture promotes loose coupling, enabling modular and flexible system design. Each module functions independently, allowing developers to add, remove, or update components without disrupting the entire system. This flexibility reduces maintenance costs and minimizes the risk of system-wide failures.
Real-world examples highlight the benefits of modularity. Uber's event-driven architecture processes real-time data and triggers actions across distributed services. This design ensures responsiveness and scalability, even under varying loads. Additionally, modular systems support innovation by allowing teams to develop and integrate new features independently. This adaptability provides a strategic advantage in rapidly evolving industries, ensuring that applications meet changing user expectations.
Practical Applications of Event-Driven Architecture
Image Source: unsplash
Types of Event-Driven Architectures
Event-driven architectures (EDAs) come in various forms, each tailored to specific use cases. Two primary types include simple event processing and complex event processing.
Simple Event Processing: This type focuses on responding to individual events as they occur. For example, a notification system sends alerts when a user receives a message.
Complex Event Processing (CEP): CEP analyzes patterns across multiple events to derive insights or trigger actions. For instance, fraud detection systems in financial services use CEP to identify suspicious transactions by analyzing real-time data streams.
Industries have adopted these architectures to address unique challenges. The table below highlights their applications:
Industry | Application Description |
Financial Services | Use of ML-based fraud algorithms in payment processing workflows to reduce losses. |
Retail and CPG | Implementation to create flexible supply chains during COVID-19 lockdowns. |
Transportation and Logistics | Adoption for effective track-and-trace systems to ensure employee safety. |
Government, Technology, Telecom, and Media | Utilization to address operational challenges during the pandemic. |
Use Cases in Web Development, IoT, and Gaming
Event-driven applications thrive in industries requiring responsiveness and scalability. In web development, they power real-time features like chat systems and live notifications. For example, microservices in a social media platform handle events such as likes, comments, and shares independently, ensuring seamless user interactions.
In IoT, event-driven architectures process real-time data from sensors. Smart home systems, for instance, react to events like motion detection or temperature changes to adjust lighting or HVAC systems. Market research confirms the growing adoption of IoT, with data collected from directories, databases, and interviews validating its expanding role in modern technology.
Gaming also benefits significantly from event-driven programming. Multiplayer games rely on microservices to handle events like player actions, matchmaking, and in-game purchases. This approach ensures low latency and a smooth gaming experience, even with millions of concurrent users.
Comparison with Reactive Programming
Event-driven programming and reactive programming share similarities but differ in performance, complexity, and suitability. The table below outlines these distinctions:
Aspect | Event-Driven Programming | Reactive Programming |
Performance | Handles fewer concurrent connections due to synchronous nature | Designed for high-performance, non-blocking operations, scales well across multiple cores |
Complexity | Easier to use for straightforward web server needs | More complex toolkit for reactive applications |
Suitability | Best for traditional web applications | Ideal for asynchronous, reactive applications on the JVM |
Reactive programming, particularly with tools like Vert.x, excels in building high-performance applications. Features such as the Multi-Reactor Pattern and Event Bus enhance responsiveness and resilience. These capabilities make reactive programming a preferred choice for applications requiring extensive real-time data processing.
Challenges and Solutions in Event Driven Programming
Common Debugging Challenges
Debugging event-driven systems presents unique challenges due to their asynchronous nature. Developers often struggle to trace the flow of events across multiple components, especially in distributed architectures. Observability issues frequently arise, making it difficult to pinpoint where an event gets delayed or lost. For example, developers may encounter situations where messages fail to propagate through the system, leaving no trace in logs or monitoring tools.
Observing the event flow appears significantly in observability challenges (89.47%). This highlights the difficulties developers face in understanding the progress of events as they flow across microservices.
Another common issue involves tracking data, such as JSON payloads, as they move through various microservices. Developers often ask how to visualize these flows or troubleshoot when events fail to trigger the expected actions. Without proper tools, debugging becomes time-consuming and error-prone.
Strategies for Managing Complexity
Managing asynchronous events in event-driven systems requires careful planning and design. One effective strategy is to implement centralized logging and monitoring tools. These tools provide a comprehensive view of event flows, helping developers identify bottlenecks and errors. Another approach involves using correlation IDs to track events across different services. This technique simplifies debugging by linking related events, even in complex workflows.
Developers can also reduce complexity by adopting modular designs. Breaking down systems into smaller, independent components makes it easier to manage and scale. Additionally, defining clear event schemas ensures consistency and reduces the likelihood of errors during event processing.
Tools and Frameworks for Event-Driven Systems
Several tools and frameworks simplify the development and debugging of event-driven systems. For instance, Apache Kafka and RabbitMQ are popular message brokers that facilitate reliable event communication. These tools support features like message persistence and fault tolerance, ensuring smooth event processing.
For observability, tools like Jaeger and Zipkin enable distributed tracing, allowing developers to visualize event flows across microservices. These tools help identify performance bottlenecks and ensure that events are processed efficiently. Frameworks like Node.js and Spring Boot also provide built-in support for managing asynchronous events, making them ideal for building scalable applications.
Event driven programming has revolutionized how modern applications respond to user actions and system events. Its core concepts, such as event sources, listeners, and the event loop, enable developers to create highly responsive and scalable systems. This paradigm supports diverse applications, from web development to IoT and gaming, showcasing its versatility.
Exploring event driven programming further can open doors to innovative solutions in software design. By mastering its principles, developers can build systems that adapt seamlessly to real-world demands, ensuring efficiency and user satisfaction.
FAQ
What is the main purpose of event-driven programming?
Event-driven programming focuses on responding to events, such as user actions or system signals. Its primary purpose is to create responsive and interactive applications by executing specific actions when events occur.
How does the event loop improve application performance?
The event loop enables asynchronous operations by continuously monitoring and processing events. This mechanism prevents blocking, allowing applications to handle multiple tasks simultaneously and maintain responsiveness.
Can event-driven programming be used in non-GUI applications?
Yes, event-driven programming applies to various domains beyond GUIs. It is widely used in server-side applications, IoT systems, and real-time data processing, where responsiveness and scalability are critical.
What are some common tools for event-driven programming?
Popular tools include Node.js for JavaScript-based applications, Apache Kafka for event streaming, and RabbitMQ for message brokering. These tools simplify event handling and improve system efficiency.
How does event-driven programming differ from reactive programming?
Event-driven programming reacts to discrete events, while reactive programming focuses on data streams and propagating changes. Reactive programming is ideal for high-performance, non-blocking applications, whereas event-driven programming suits simpler, event-based workflows.
Subscribe to my newsletter
Read articles from Community Contribution directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
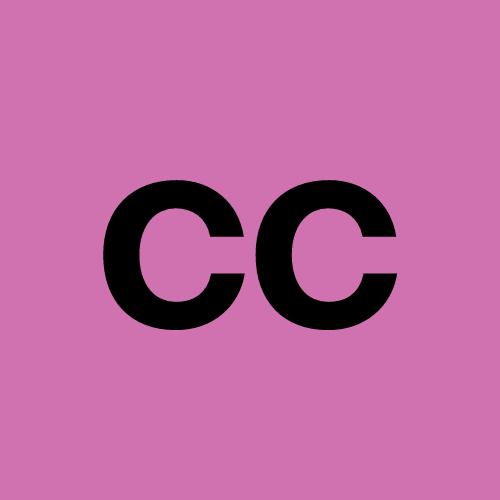