What Are the Best Practices for Using Tailwind CSS in a React Project?
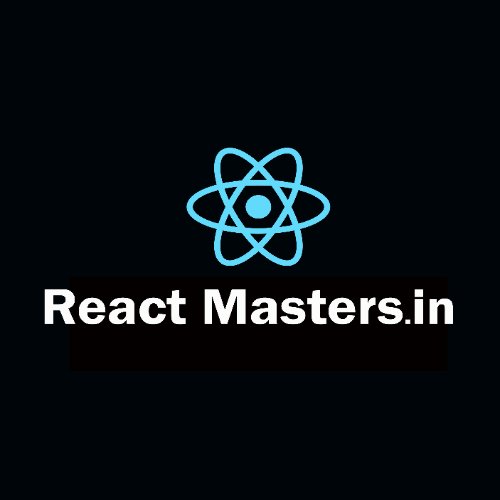

Introduction
Tailwind CSS has revolutionized the way developers build UI with utility-first CSS classes. When combined with React, a powerful JavaScript library for building user interfaces, Tailwind CSS enables developers to create fast, responsive, and maintainable applications.
However, to truly harness its potential in a React project, it's crucial to follow best practices that ensure clean code, scalability, and improved developer experience.
In this article, we’ll dive deep into the best practices for using Tailwind CSS in React projects, covering setup, structure, componentization, performance, and tooling.
1. Proper Project Setup
Use PostCSS and Tailwind CLI
Set up Tailwind using its official CLI with PostCSS integration for full customization:
bashCopyEditnpx create-react-app my-app
cd my-app
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
Configure Tailwind in tailwind.config.js
Enable JIT mode (Just-In-Time compilation) and set up purge paths to eliminate unused styles:
jsCopyEditmodule.exports = {
content: ['./src/**/*.{js,jsx,ts,tsx}'],
theme: {
extend: {},
},
plugins: [],
};
2. Use Semantic Class Naming Through Components
Convert Repetitive Classes into Reusable Components
Instead of repeating long Tailwind class strings, extract them into reusable React components:
jsxCopyEditconst PrimaryButton = ({ children }) => (
<button className="bg-blue-500 hover:bg-blue-600 text-white font-bold py-2 px-4 rounded">
{children}
</button>
);
Benefits:
Improved readability
Easier maintenance
Consistent styling
3. Use Utility Classes with Conditional Styling
Leverage libraries like clsx
or classnames
to apply Tailwind classes conditionally:
bashCopyEditnpm install clsx
jsxCopyEditimport clsx from 'clsx';
const Button = ({ isActive }) => (
<button className={clsx('py-2 px-4 font-semibold', isActive ? 'bg-green-500' : 'bg-gray-300')}>
Click Me
</button>
);
4. Organize Styles with Tailwind Plugins
Tailwind supports official and community plugins. Use them to extend functionality:
@tailwindcss/forms
for form inputs@tailwindcss/aspect-ratio
for media@tailwindcss/typography
for rich text styling
Install and include in your config:
bashCopyEditnpm install @tailwindcss/forms
jsCopyEditplugins: [require('@tailwindcss/forms')],
5. Adopt Consistent Design Tokens
Use the Tailwind config to define a consistent design system:
jsCopyEdittheme: {
extend: {
colors: {
brand: '#1DA1F2',
},
fontFamily: {
sans: ['Inter', 'sans-serif'],
},
},
}
This avoids scattered design choices and improves collaboration across teams.
6. Integrate Dark Mode Thoughtfully
Tailwind supports dark mode out-of-the-box. Use media
or class
strategies:
jsCopyEditdarkMode: 'class',
Then toggle with:
jsxCopyEdit<div className="bg-white dark:bg-gray-900 text-black dark:text-white">Hello</div>
7. Keep Class Names Manageable
Split Long Classes into Multiple Lines
For better readability in JSX:
jsxCopyEdit<div
className="
bg-white
p-6
rounded-lg
shadow-md
hover:shadow-xl
transition-all
duration-300"
>
Card Content
</div>
8. Linting and Prettier Configuration
Use Prettier plugins to format Tailwind classes alphabetically or based on groups:
bashCopyEditnpm install -D prettier prettier-plugin-tailwindcss
Then add to .prettierrc
:
jsonCopyEdit{
"plugins": ["prettier-plugin-tailwindcss"]
}
9. Avoid Inline Styles Unless Necessary
Tailwind offers nearly all CSS features through utility classes. Use inline styles only for dynamic values that Tailwind can't express (e.g., dynamic heights or inline SVGs).
10. Use Responsive and Mobile-First Utilities
Tailwind follows mobile-first principles. Use breakpoints smartly:
jsxCopyEdit<div className="text-sm md:text-base lg:text-lg">Responsive Text</div>
This ensures your app looks great on all screen sizes.
Conclusion
Tailwind CSS with React can dramatically enhance your development workflow, performance, and UI consistency. Following these best practices ensures that your code remains scalable, clean, and professional, making collaboration and maintenance far more efficient.
Embrace the utility-first mindset, and let Tailwind + React become your powerhouse duo for frontend development.
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
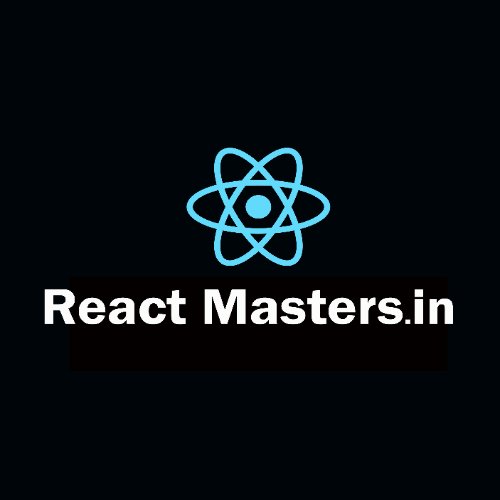
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.