Shell & Scripting
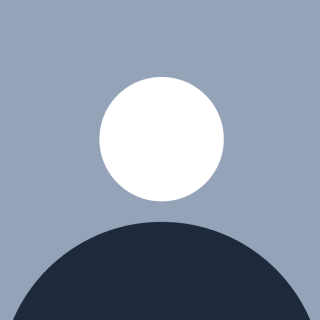

A program that takes commands from the user and sends them to the OS (e.g., Bash, Zsh)
✅ Key Concepts:
What is a Shell?
- A program that takes commands from the user and sends them to the OS (e.g., Bash, Zsh).
Popular Shell:
bash
(Bourne Again SHell)
✅ Basic Script Example:
#!/bin/bash
echo "Hello, DevOps!"
✅ How to Run a Script:
chmod +x script.sh # Make it executable
./script.sh # Run the script
Variables and User Input
✅ Define and Use Variables:
name="DevOpsUser"
echo "Welcome, $name"
✅ Read Input:
echo "Enter your name:"
read username
echo "Hello, $username"
✅ Quoting:
Double quotes
" "
allow variable expansionSingle quotes
' '
treat everything literally
Conditional Statements
✅ If-Else Syntax:
if [ "$name" == "admin" ]; then
echo "Welcome, Admin"
else
echo "Access Denied"
fi
✅ File Test Examples:
if [ -f /etc/passwd ]; then
echo "File exists"
fi
Loops
✅ For Loop:
for i in 1 2 3; do
echo "Loop #$i"
done
✅ While Loop:
count=1
while [ $count -le 5 ]; do
echo "Count: $count"
((count++))
done
Functions
✅ Define and Call a Function:
say_hello() {
echo "Hello from a function"
}
say_hello
✅ Return Values:
sum() {
return $(($1 + $2))
}
sum 3 4
echo $? # Outputs 7
After learning the basic concept of Bash script. we can do the practice for writing any script which may help us to do our work. Like just an example of Update your system, cleans temp files & checks disk space etc.
#!/bin/bash
echo "Updating Linux based system..."
sudo apt update && sudo apt upgrade -y
echo "Cleaning /tmp..."
rm -rf /tmp/*
echo "Disk usage:"
df -h
save this file as “maintenance.sh” and execute it. it will return output like given below.
$ ./maintenance.sh
Updating system...
Hit:1 http://security.ubuntu.com/ubuntu focal-security InRelease
Hit:2 http://archive.ubuntu.com/ubuntu focal InRelease
Reading package lists... Done
Building dependency tree
Reading state information... Done
Calculating upgrade... Done
The following packages will be upgraded:
openssl libssl1.1 ...
N upgraded, 0 newly installed, 0 to remove and N not upgraded.
Need to get 3,000 kB of archives.
After this operation, 1,024 kB of additional disk space will be used.
Do you want to continue? [Y/n] y
...
Cleaning /tmp...
Disk usage:
Filesystem Size Used Avail Use% Mounted on
/dev/sda1 50G 15G 32G 32% /
tmpfs 1.9G 2.1M 1.9G 1% /tmp
🔥 Next Steps: Once you're comfortable, dive into Git, Bash scripting, Ansible, Docker, and Kubernetes — all of which build on these Linux foundations.
Thanks for reading, Happy Learning ~Prem G
#Linux #Bash #Scripting #DevOps #SysAdmin #CloudComputing #DevOpsJourney #TechLearning #LinuxForDevOps #LearningInPublic #TechJourney #30DaysOfDevOps #shubhamLondhe #TrainWithShubham #KorboLorboJeetbo
Subscribe to my newsletter
Read articles from Prem Kr Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Prem Kr Gupta
Prem Kr Gupta
A software developer aiming to become a DevOps engineer, exploring and sharing insights on DevOps methodology.