CSS Media Query in Javascript
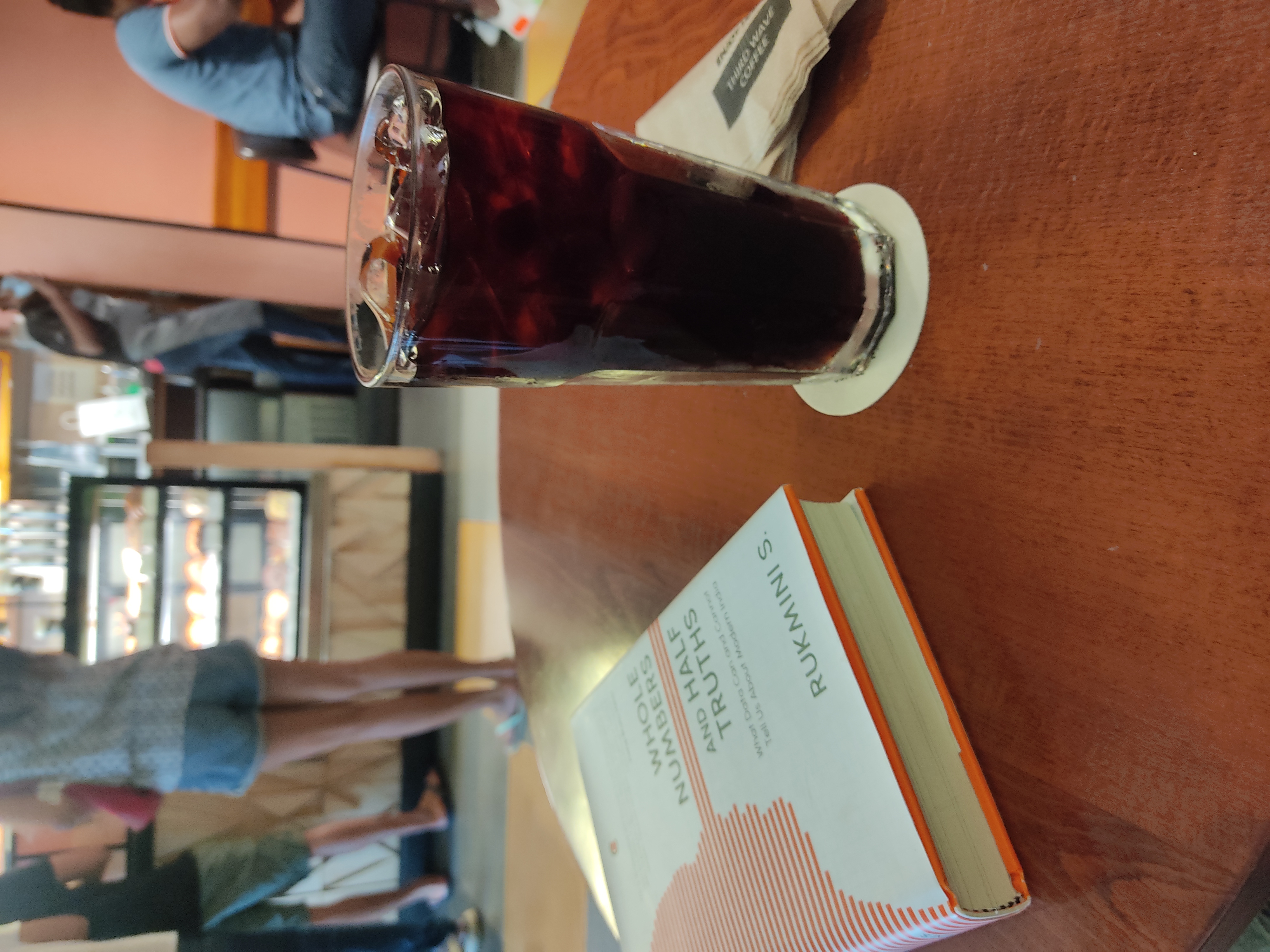
Table of contents

Quick Summary
CSS media queries are very effective and optimal for applying styles based on device properties and settings. We’re going to look at a feature in javascript that let’s us use CSS media queries in our JS.
What’s matchMedia?
matchMedia
is a method available on the window
object that can be used to match or track a CSS media query on the document
. Read more about it on MDN.
Syntax
Taking a CSS media query for example
@media (max-width: 480px) {
body {
font-size: 16px;
background-color: lightgray;
}
}
We would need what’s written after @media
as it is
const matchMobileQuery = window.matchMedia("(max-width: 480px)");
Testing it on the browser console, here’s what the matchMedia returns
So we can take the matches
variable to check whether the query matched or not.
To Track a media query, we can add a change
event listener on the same MediaQueryList
object given by the matchMedia
.
Let’s test this with an example of prefers-color-scheme
media query
const mediaQuery = window.matchMedia('(prefers-color-scheme: dark)');
function handleChange(e) {
if (e.matches) {
console.log('Dark mode');
} else {
console.log('Light mode');
}
}
mediaQuery.addEventListener('change', handleChange);
We should see a console log whenever the color scheme changes
When to use?
To replace
window.innerWidth
to find out what device we’re onReplace any element size tracking with
resizeObserver
, if it can be solved with a media queryIt’s not just for screen size—use it to react to dark mode, device orientation, and more
Thanks for reading!
If you made it till here, let’s hear your thoughts on this in the comments!
Subscribe to my newsletter
Read articles from Gulshan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
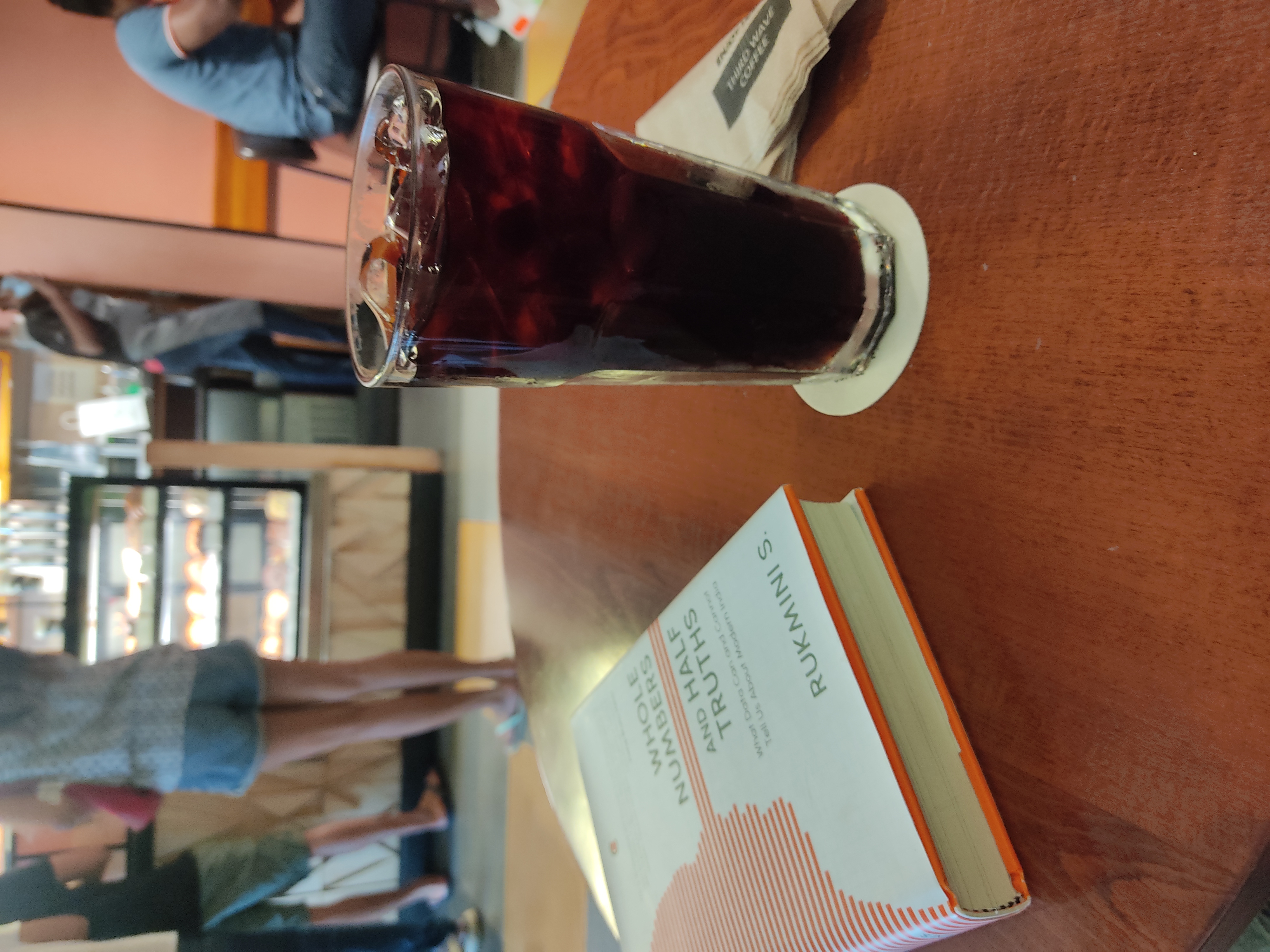
Gulshan
Gulshan
I'm a frontend engineer with 4+ years of experience in building web-apps. I like to share my experience through writing, hoping to help other devs find out something interesting or useful.