Springboot vs Node JS
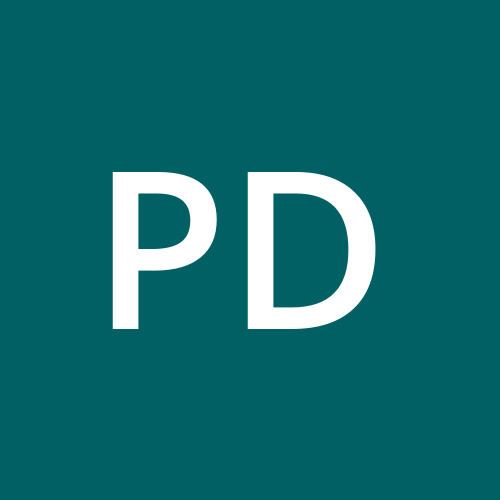

When building simple APIs or prototypes, both Node.js and Spring Boot can get the job done. But, when you scale up to complex backend systems that involve domain-heavy logic, security and performance, Spring Boot offers more structure and safety by default. In this post, we'll explore concrete reasons why that's the case, with side-by-side comparisons.
1. Type Safety and Domain Modeling
Java (Spring Boot)
public class Payment {
private BigDecimal amount;
private Currency currency;
private PaymentStatus status;
public void markAsRefunded() {
if (status == PaymentStatus.COMPLETED) {
this.status = PaymentStatus.REFUNDED;
} else {
throw new IllegalStateException("Cannot refund incomplete payment");
}
}
}
With Java, the compiler enforces types and access rules (private, protected, public). This protects business logic from accidental misuse and keeps domain models consistent. For example, it is impossible to have a random value in an enum field.
Node.js (especially with TypeScript) can use classes to replicate much of the structure we see in Java. In fact, we could write a nearly equivalent version of our Payment
class in TypeScript:
✅ TypeScript Equivalent
enum PaymentStatus {
COMPLETED = 'COMPLETED',
REFUNDED = 'REFUNDED',
PENDING = 'PENDING'
}
class Payment {
private amount: number;
private currency: string;
private status: PaymentStatus;
constructor(amount: number, currency: string, status: PaymentStatus) {
this.amount = amount;
this.currency = currency;
this.status = status;
}
public markAsRefunded() {
if (this.status === PaymentStatus.COMPLETED) {
this.status = PaymentStatus.REFUNDED;
} else {
throw new Error('Cannot refund incomplete payment');
}
}
public getStatus(): PaymentStatus {
return this.status;
}
}
Lets see an example here. Although, typescript enforces at compile time that the value for status
field should be one of those values defined within enum: PaymentStatus
. But, this does not work at runtime.
Example: TypeScript’s private
Is Not Really Private
const payment = new Payment(100);
// This compiles with an error in TypeScript.
// But works fine at runtime if you bypass the compiler!
console.log((payment as any).amount);
Problem: With
private
, anyone can cast toany
and mutate the “private” property. The compiler helps, but the runtime doesn't stop you.
How to overcome the limitation?
class SecurePayment {
#amount: number;
constructor(amount: number) {
this.#amount = amount;
}
getAmount() {
return this.#amount;
}
}
const secure = new SecurePayment(200);
console.log(secure.getAmount()); // ✅ 200
console.log((secure as any).#amount); // ❌ Syntax error — doesn't compile or run
console.log((secure as any)['#amount']); // ❌ undefined — cannot access it by name
Benefit: This is enforced at runtime — no reflection or hack can break into #amount
.
Still, invalid values could sip in at runtime. For example, invalid value could be set in an enum type if we construct the object using json.
const json = '{"amount": 100, "currency": "USD", "status": "FAKE_STATUS"}';
const payment = JSON.parse(json);
const pm = new Payment(payment.amount, payment.currency, payment.status);
To prevent that, we can add validation logic inside the constructor. It is quite a work, agreed?
These types of compile and runtime safety are provided by java inherently. So, java gives us stronger immutability and encapsulation.
2. Framework-Level Validation
Spring Boot: Auto-validates DTOs with annotations like
@Min
,@Email
, and triggers errors without writing manual checks.public class UserDTO { @NotBlank @Email private String email; @Min(18) private int age; }
Validation is automatic and enforced by annotations when using
@Valid
in controller methods.Node.js: You must integrate manual schema validation (e.g., with Joi/Zod), and it’s easy to skip or misconfigure.
const schema = Joi.object({ email: Joi.string().email().required(), age: Joi.number().min(18).required() });
In Node.js, you must remember to validate and handle errors yourself — a missed step could lead to bugs.
3. Concurrency and Multithreading
Spring Boot
ExecutorService executor = Executors.newFixedThreadPool(10);
executor.submit(() -> processLargeFile());
Java apps naturally leverage multiple CPU cores, and concurrency is built-in.
Node.js
Node is single-threaded. For heavy processing, you need to manually set up Worker Threads or cluster mode, increasing complexity.
4. Exception Handling
Spring Boot
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<?> handleNotFound(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
You can define global exception handlers declaratively, safer, centralized, and reusable.
Node.js
app.use((err, req, res, next) => {
res.status(500).send({ error: err.message });
});
It's manual. You must wire everything up explicitly and defensively.
5. Transactions
Spring Boot
@Transactional
public void transferMoney(Long fromId, Long toId, BigDecimal amount) {
// Automatic rollback on failure
}
With @Transactional
, Spring Boot manages database commits and rollbacks seamlessly.
Node.js
await db.transaction(async (trx) => {
await trx('accounts').decrement('balance', amount).where({ id: fromId });
await trx('accounts').increment('balance', amount).where({ id: toId });
});
You need to manage every step and handle rollback logic manually.
6. Spring boot has built in support for microservices:
Spring Boot offers built-in support for developing microservices, making it a strong choice for enterprise-level applications. It provides out-of-the-box features like service discovery with Spring Cloud, centralized configuration, circuit breakers, and seamless integration with tools like Eureka, Zuul, and Hystrix. In contrast, Node.js does not provide dedicated, built-in microservice support. While it is highly flexible and lightweight, developers must rely on third-party libraries and frameworks to manually implement features like service discovery, load balancing, and fault tolerance. This often results in more configuration effort and architectural decisions being placed on the developer.
Final Thoughts
Node.js is fantastic for lightweight APIs, real-time apps, and rapid prototyping. But when your system grows in complexity and scale, Spring Boot shines with its built-in structure:
Strong typing and modeling
Built-in validation and transactions
Native multithreading
Declarative exception handling
This doesn’t mean Java is always better, just that Spring Boot provides a more structured foundation out of the box, especially for complex business logic.
Subscribe to my newsletter
Read articles from pramithas dhakal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
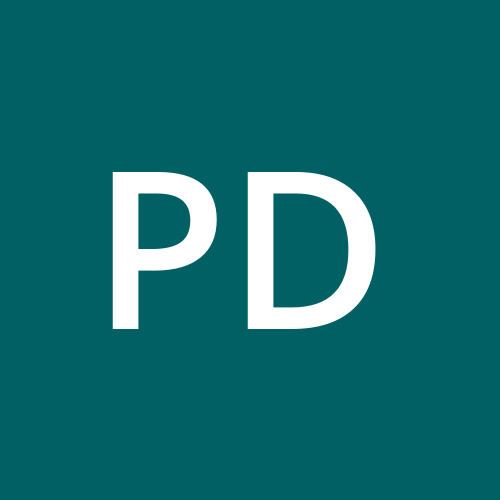
pramithas dhakal
pramithas dhakal
Lifelong learner