🔥 Mastering DevOps: Overcoming Linux Challenges with Practical Scripting Tasks
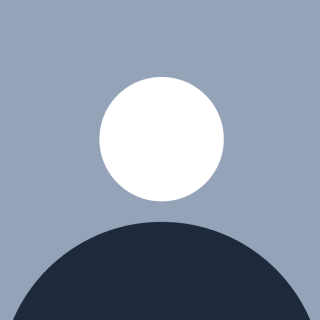
Mastering DevOps requires overcoming various challenges, especially when working with Linux systems. This article presents practical scripting tasks designed to help you tackle these challenges effectively. Here are some tasks to get you started:
🔹 Challenge 1: Write a simple Bash script that prints “Welcome to World of DevOps!” along with the current date and time.
🔹 Challenge 2: Create a script that checks if a website (e.g., https://www.google.com) is reachable using curl or ping. Print a success or failure message.
🔹 Challenge 3: Write a script that takes a filename as an argument, checks if it exists, and prints the content of the file accordingly.
🔹 Challenge 4: Create a script that lists all running processes and writes the output to a file named process_list.txt.
🔹 Challenge 5: Write a script that installs multiple packages at once (e.g., git, vim, curl). The script should check if each package is already installed before attempting installation.
Solution:
✅ Challenge 1: Write a simple Bash script that prints “Welcome to World of DevOps!” along with the current date and time.
Answer : Create Hello_Dev.sh
and write code by using Nano command to make changes in file. write #!/bin/bash echo "Welcome to World of DevOps! Today's date and time is : $(date)"
make this file executable by chmod +x Hello_dev.sh
command and run this file. you will see the whole terminal commands and output given below
Explanation:
#!/bin/bash
→ Shebang to specify Bash as the interpreter.echo "Welcome to World of DevOps! Today's date and time is : $(date)"
echo
prints the message.$(date)
fetches the current date and time.
[ec2-user@ip-172-31-80-217 ~]$ mkdir BashScripts
[ec2-user@ip-172-31-80-217 ~]$ cd BashScripts/
[ec2-user@ip-172-31-80-217 BashScripts]$ ll
total 0
[ec2-user@ip-172-31-80-217 BashScripts]$ nano Hello_dev.sh
[ec2-user@ip-172-31-80-217 BashScripts]$ cat Hello_dev.sh
#!/bin/bash
echo "Welcome to World of DevOps! Today's date and time is : $(date)"
[ec2-user@ip-172-31-80-217 BashScripts]$ ls -l
total 4
-rw-r--r--. 1 ec2-user ec2-user 83 Apr 24 07:34 Hello_dev.sh
[ec2-user@ip-172-31-80-217 BashScripts]$ chmod +x Hello_dev.sh
[ec2-user@ip-172-31-80-217 BashScripts]$ ls -l
total 4
-rwxr-xr-x. 1 ec2-user ec2-user 83 Apr 24 07:34 Hello_dev.sh
[ec2-user@ip-172-31-80-217 BashScripts]$ ./Hello_dev.sh
Welcome to World of DevOps! Today's date and time is : Thu Apr 24 07:34:47 UTC 2025
✅ Challenge 2: Create a script that checks if a website (e.g., https://www.google.com) is reachable using curl or ping. Print a success or failure message.
Explanation:
#!/bin/bash
→ Shebang (#!
): This tells the system to run the script usingbash
. This line is required to know which interpreter to use when executing the script.You define two variables:
website
is the full URL to check usingcurl
(which checks if the web server is up and responding to HTTP requests).domain
is just the domain part, used withping
to check network-level connectivity.
# Define the website address to check
website="https://google.com"
domain="google.com"
# Check website availability using curl
http_code=$(curl -o /dev/null -s -w "%{http_code}" --max-time 5 "$website")
This line uses
curl
to silently make a request to the URL and fetch only the HTTP status code:-o /dev/null
: Discards the response body.-s
: Runs silently (no progress output).-w "%{http_code}"
: Outputs only the HTTP status code (e.g., 200, 301, 404).--max-time 5
: Limits the request to 5 seconds to avoid long waits.
The result is saved into the variable
http_code
.
if echo "$http_code" | grep -qE "200|301|302"; then
This checks if the status code returned by
curl
indicates a successful or redirect response:200
means OK (successful request).301
and302
are redirects (still means the server is up).-qE
withgrep
: quiet mode with extended regex.
If one of those is matched, it proceeds with the success message:
if ping -c 2 -W 2 "$domain" > /dev/null 2>&1; then
This runs a
ping
to check basic network connectivity:-c 2
: Send 2 packets.-W 2
: Wait up to 2 seconds for a response.> /dev/null 2>&1
: Discards both stdout and stderr (silent).
If
ping
succeeds, it prints another success message , If bothcurl
andping
fail, it prints a final failure message.
[ec2-user@ip-172-31-80-217 BashScripts]$ nano Website_check.sh
[ec2-user@ip-172-31-80-217 BashScripts]$ ls -l
total 8
-rwxr-xr-x. 1 ec2-user ec2-user 83 Apr 24 07:34 Hello_dev.sh
-rwxr-xr-x. 1 ec2-user ec2-user 619 Apr 24 08:12 Website_check.sh
[ec2-user@ip-172-31-80-217 BashScripts]$ ./Website_check.sh
Success: https://google.com is reachable via curl!
[ec2-user@ip-172-31-80-217 BashScripts]$ cat Website_check.sh
#!/bin/bash
# Define the website address to check
website="https://google.com"
domain="google.com"
# Check website availability using curl
http_code=$(curl -o /dev/null -s -w "%{http_code}" --max-time 5 "$website")
if echo "$http_code" | grep -qE "200|301|302"; then
echo "Success: $website is reachable via curl!"
else
echo "Curl check failed (HTTP $http_code), trying ping..."
# Website check using Ping
if ping -c 2 -W 2 "$domain" > /dev/null 2>&1; then
echo "Success: $domain is reachable via ping!"
else
echo "Failure: Website is not reachable via curl or ping."
fi
fi
Subscribe to my newsletter
Read articles from Prem Kr Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Prem Kr Gupta
Prem Kr Gupta
A software developer aiming to become a DevOps engineer, exploring and sharing insights on DevOps methodology.