Pattern Matching with instanceof in Java 17
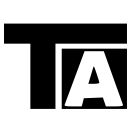
5 min read
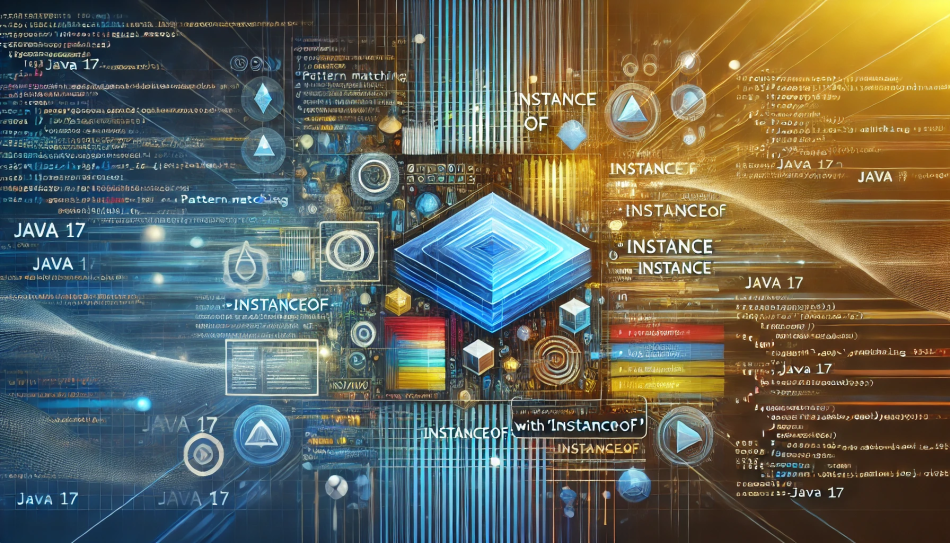
1. What is Pattern Matching for instanceof?
This feature allows us to perform type casts and assignments in a single step. In previous versions, you’d typically follow an instanceof check with a cast. Java 17 streamlines this by combining these actions, reducing boilerplate code and potential for errors.
1.1 Traditional instanceof Check and Casting
In versions prior to Java 17, you might see code like this:
Object obj = "Hello, World!";
if (obj instanceof String) {
String str = (String) obj;
System.out.println("String length: " + str.length());
}
While this is functional, it requires both the instanceof check and a cast, introducing redundancy.
.2 Pattern Matching Syntax in Java 17
Java 17 lets you reduce this redundancy:
Object obj = "Hello, World!";
if (obj instanceof String str) {
System.out.println("String length: " + str.length());
}
Here, if obj is an instance of String, it is simultaneously cast and assigned to str.
1.3 Why Use Pattern Matching?
This feature isn’t just for brevity; it improves readability and eliminates potential errors by reducing unnecessary casting. You’re now less likely to accidentally use a type mismatch, enhancing code safety.
1.4 Pattern Matching and Null Safety
Java’s instanceof has always been null-safe, meaning that null instanceof Type always returns false. Pattern matching is consistent with this behavior:
Object obj = null;
if (obj instanceof String str) {
// This block will not execute since obj is null.
System.out.println(str);
}
Null-safe instanceof checks combined with pattern matching add a layer of robustness, particularly when dealing with potentially null values.
2. Best Practices for Pattern Matching with instanceof
While pattern matching is straightforward, some techniques can make your code even more effective.
2.1 Using Pattern Matching in Switch Expressions
Java 17’s pattern matching works seamlessly with switch expressions, allowing for even cleaner, more expressive code:
Object obj = "Hello, Java!";
switch (obj) {
case String s -> System.out.println("String of length: " + s.length());
case Integer i -> System.out.println("Integer value: " + i);
default -> System.out.println("Unknown type");
}
Combining instanceof pattern matching with switch expressions in this way can simplify complex conditionals, making your code more expressive and readable.
2.2 Avoiding Ambiguities in Complex Conditions
When using pattern matching, it’s essential to keep the conditions clear. Nesting conditions can lead to confusion:
if (obj instanceof String str && str.length() > 10) {
System.out.println("Long string: " + str);
}
If conditions become too complex, it’s better to split them for readability:
if (obj instanceof String str) {
if (str.length() > 10) {
System.out.println("Long string: " + str);
}
}
2.3 Performance Considerations
Pattern matching doesn’t change the runtime behavior of instanceof. In terms of performance, Java handles it in the same way as traditional instanceof checks with casting. However, it does save a little on memory and processing by reducing redundant casts.
2.4 Error Handling with Pattern Matching
Pattern matching for instanceof can make error handling more precise. Instead of catching general exceptions and casting objects, you can use pattern matching to filter and handle specific types directly:
try {
// Code that may throw different types of exceptions
} catch (Exception e) {
if (e instanceof NullPointerException npe) {
System.out.println("Null pointer issue: " + npe.getMessage());
} else if (e instanceof IllegalArgumentException iae) {
System.out.println("Illegal argument: " + iae.getMessage());
} else {
System.out.println("Other exception: " + e.getMessage());
}
}
3. Common Issues with Pattern Matching for instanceof
Pattern matching is a powerful tool, but there are a few considerations to keep in mind to avoid pitfalls.
3.1 Compatibility Issues
Using pattern matching means your code is not backward-compatible with versions before Java 17. It’s crucial to consider the Java version if you’re working on projects with older environments or teams that have yet to migrate.
3.2 Accidental Use with Non-Final Variables
Remember that pattern variables are effectively final. Attempting to reassign them will cause compilation errors:
Object obj = "Text";
if (obj instanceof String str) {
str = "New Text"; // Compilation error: cannot reassign str
}
This immutability feature prevents accidental changes to pattern-matched variables.
3.3 Pattern Matching in Streams
While pattern matching can be convenient, using it within streams is limited, as streams currently do not directly support instanceof pattern matching.
4. Leveraging Pattern Matching in Real-World Applications
To bring it all together, let’s look at how to implement pattern matching within a simple application that processes different data types:
public class DataProcessor {
public static void processData(Object obj) {
if (obj instanceof String s) {
System.out.println("String data: " + s.toUpperCase());
} else if (obj instanceof Integer i) {
System.out.println("Integer data: " + (i * 2));
} else {
System.out.println("Unrecognized data type");
}
}
public static void main(String[] args) {
processData("Pattern Matching");
processData(42);
processData(3.14);
}
}
Output:
String data: PATTERN MATCHING
Integer data: 84
Unrecognized data type
This example illustrates how pattern matching allows us to handle different data types dynamically and with minimal code.
5. Conclusion
Pattern matching for instanceof in Java 17 simplifies code, making it more readable and maintainable. While it’s a helpful feature, it’s essential to be mindful of backward compatibility and avoid over-complicating conditions. Are there questions about pattern matching for instanceof? Feel free to leave a comment below!
Read more at : Pattern Matching with instanceof in Java 17
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
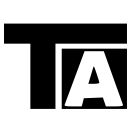
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.