Handling OutOfMemoryError: Requested Array Size Issues
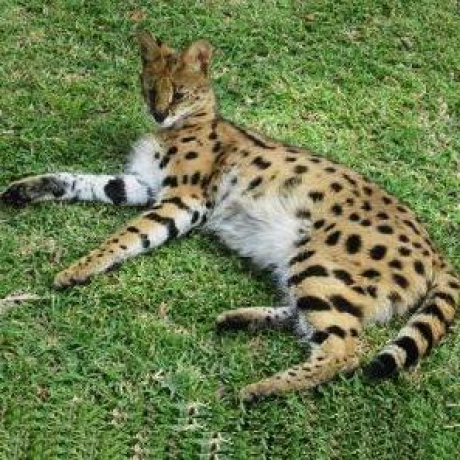

In Java, there are nine types of Out of Memory errors, each caused by a different issue. When it comes to debugging and solving these errors, we need to tailor our strategy to the particular type of error.
In this article, we’ll be concentrating on the error message: java.lang.OutOfMemoryError: Requested array size exceeds VM limit
. This error is rarely seen, and fortunately it’s one of the easiest to debug. Let’s look at what causes array size issues, and how we can solve them.
The JVM Memory Model
Java runtime memory can be represented like this:
Fig: JVM Memory Model
The heap, highlighted in red, is the area affected by array size issues. This area is used to store all objects created by the application. It’s split into the young generation and the old generation to optimize garbage collection. Arrays are always created in the heap.
The non-heap areas are created in native memory, and include stack spaces for each thread, metadata for each class, and other areas.
For more information on JVM memory and how it relates to java.lang.OutOfMemoryError
-- requested array size issues, you may like to read: Out of Memory Error: Requested Array Size.
What Causes java.lang.OutOfMemoryError: Requested Array Size Issues?
The JVM has certain limits as to the maximum size of an array. This is, to some extent, platform-dependent. In general, it relates to the maximum value that can be stored in an integer. This is because arrays are always indexed by integers, so it makes sense that we can’t have an array that’s too large to index.
The maximum value that can be stored in an integer is represented in Java by the constant Integer.MAX_VALUE. This is, in fact, 231, or 2 147 483 648 -- roughly 2 billion. Most platforms allow array sizes slightly less than this number to allow for array overheads. In Oracle Java, it’s said to be safe to create arrays up to ( 231 – 8) in size.
So, in a practical sense, what’s likely to cause this error? How often are we likely to want to create an array bigger than this maximum? Here are a few cases where the error may occur:
An application creates an array to hold all rows in a database table. This works fine, until the database grows, and has more rows than the JVM’s maximum array size.
An application uses an algorithm to calculate the size of an array. However, there’s a bug in the algorithm, and it inadvertently tries to create an array that’s too large.
Troubleshooting Array Size Issues
Troubleshooting this issue is very simple. When the error is thrown, the JVM will print a stack trace that looks like this:
Fig: Error Message with Stack Trace***
The first line after the error message states the exact line number within the source code that the program was executing when the error occurred. We have only to go to the source and fix the line that caused the problem.
Solutions
The solution to this error is simple in theory, but may involve some rethinking of the program in practice. We have to make the array smaller, so it doesn’t exceed the JVM limits. This may involve:
Checking the code that calculates the array size is working correctly;
If we’re loading rows from a database into an array, we can consider using filters so only relevant rows are loaded;
Consider processing the array in smaller batches;
Change program strategy.
Also bear in mind that the maximum array size is very large, and even if we bring it below the allowed maximum, we could find ourselves seeing this error in its place: java.lang.OutOfMemoryError: Java heap space
. This happens whenever there’s not enough heap space to create an object. We can increase the heap space using the JVM switch -Xmx
.
Conclusion
Errors relating to java.lang.outofmemoryerror: requested array size issues
are fairly rare. They are simple to debug, since the accompanying stack trace provides the exact program line that caused the error.
They are most likely to occur when the program calculates the size of an array incorrectly, but in some cases we need to rethink program strategy.
Thank you for reading this article. Your comments would be appreciated.
Subscribe to my newsletter
Read articles from Jill Thornhill directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
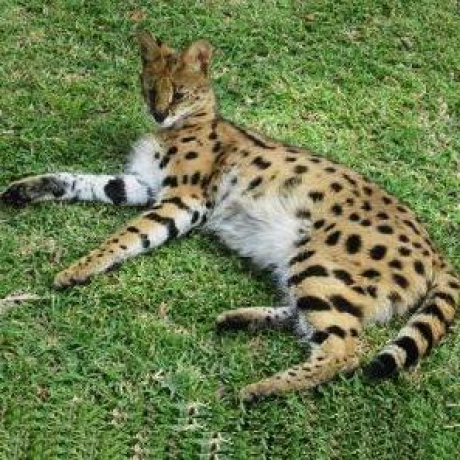