Flyweight Design Pattern in Java for Effective Memory Optimization
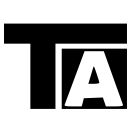
5 min read
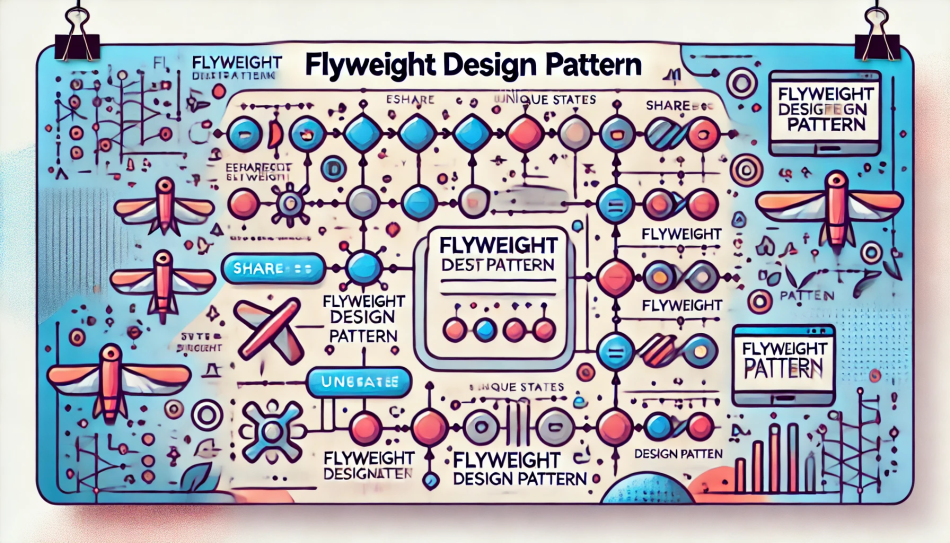
1. Understanding the Flyweight Design Pattern
The Flyweight Pattern is a structural design pattern that focuses on minimizing memory usage by sharing common parts of objects. Instead of holding multiple instances of the same data across many objects, the Flyweight Pattern allows you to centralize these shared aspects.
1.1 Key Components of the Flyweight Pattern
- Intrinsic State: The data that is shared across objects. It remains constant and does not vary.
- Extrinsic State: The data that is unique to each object instance. It is passed to the object whenever it’s needed.
- Flyweight Factory: This class ensures that only one instance of each intrinsic state is created and shared.
1.2 When to Use the Flyweight Pattern
Consider using the Flyweight Pattern when:
- There is a large number of objects that consume a significant amount of memory.
- Most of these objects share identical data, and only a few attributes vary between them.
1.3 Example Use Case: A Text Editor
In a text editor, each character on the screen could be represented by a separate object. Since documents can contain thousands of characters, creating a unique object for each character could quickly consume a large amount of memory. By using the Flyweight Pattern, we can share objects representing characters with the same font style, size, and color, storing only the character's position and extrinsic state separately.
2. Implementing the Flyweight Pattern in Java
Below, we’ll implement a basic Flyweight Pattern to demonstrate memory optimization.
2.1 Step 1: Define the Flyweight Interface
The Flyweight interface declares methods that will operate on both intrinsic and extrinsic states.
public interface Character {
void display(int x, int y); // x and y are extrinsic state
}
2.2 Step 2: Create Concrete Flyweight Classes
The ConcreteCharacter class implements the Character interface. It holds the intrinsic state, in this case, the char value and font information. Extrinsic data, such as x and y positions, are provided as parameters.
public class ConcreteCharacter implements Character {
private final char symbol;
private final String font;
public ConcreteCharacter(char symbol, String font) {
this.symbol = symbol;
this.font = font;
}
@Override
public void display(int x, int y) {
System.out.println("Displaying character " + symbol + " at (" + x + ", " + y + ") with font " + font);
}
}
2.3 Step 3: Create a Flyweight Factory
The Flyweight Factory is responsible for creating and managing Flyweight objects. It stores the shared instances in a map, and if an instance with a specific intrinsic state already exists, it returns that instance instead of creating a new one.
import java.util.HashMap;
import java.util.Map;
public class CharacterFactory {
private Map<String, Character> characters = new HashMap<>();
public Character getCharacter(char symbol, String font) {
String key = symbol + "-" + font;
if (!characters.containsKey(key)) {
characters.put(key, new ConcreteCharacter(symbol, font));
}
return characters.get(key);
}
}
2.4 Step 4: Use the Flyweight in the Client Code
In the main method, we utilize the Flyweight Pattern by creating multiple character instances but sharing the same intrinsic data for characters with the same appearance.
public class FlyweightDemo {
public static void main(String[] args) {
CharacterFactory factory = new CharacterFactory();
Character c1 = factory.getCharacter('A', "Arial");
Character c2 = factory.getCharacter('A', "Arial");
Character c3 = factory.getCharacter('B', "Arial");
// Display characters at different positions
c1.display(10, 20);
c2.display(15, 25);
c3.display(30, 40);
System.out.println("Are c1 and c2 the same object? " + (c1 == c2)); // Should print true
}
}
3. Best Practices for Using the Flyweight Pattern
3.1 Keep Extrinsic Data External
One of the best practices for using the Flyweight Pattern effectively is to ensure that extrinsic data is not stored within the Flyweight objects. This helps to maintain the memory savings by keeping only the essential shared data within the objects.
3.2 Use the Flyweight Pattern with Caution
Not all applications benefit from the Flyweight Pattern. The pattern is best suited for scenarios where there is significant data repetition. If the objects have minimal or no shared data, this pattern could lead to unnecessary complexity.
3.3 Monitor and Measure Memory Consumption
Always monitor memory usage to confirm that the Flyweight Pattern is indeed reducing your application’s memory footprint. Java profiling tools, like VisualVM, can help you to see whether memory usage has improved after implementing this pattern.
4. Potential Issues with the Flyweight Pattern
4.1 Increased Complexity
The Flyweight Pattern adds a layer of complexity, especially in managing extrinsic data. In cases where the extrinsic state is extensive, managing it can become cumbersome.
4.2 Limited Flexibility
Once implemented, the intrinsic state within Flyweight objects cannot be easily modified. This can be limiting if you need to support frequent updates to shared data.
4.3 Trade-off Between Memory and Performance
While the Flyweight Pattern reduces memory usage, it can also increase the time complexity due to the additional process of looking up and managing shared instances.
5. Conclusion
The Flyweight Design Pattern is an effective solution for optimizing memory in Java applications. When used appropriately, it can significantly reduce the memory footprint by sharing data across similar objects. However, it’s important to consider the added complexity and potential limitations of this pattern. If you have any questions or would like further clarification, please feel free to leave a comment below!
Read more at : Flyweight Design Pattern in Java for Effective Memory Optimization
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
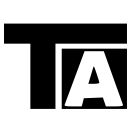
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.