Mutable vs Immutable in Python: Explained with Examples and Memory Diagrams
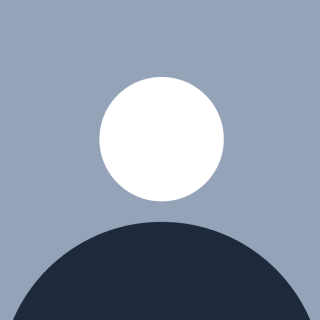
Ever changed a list and wondered why your original variable changed too? Or assigned a string and somehow it didn’t? That’s Python being clever with mutable and immutable types.
Don’t worry if it sounds confusing — let’s break it down like we’re chatting over chai ☕.
🔁 So, what’s "mutable" anyway?
In Python:
Mutable = can change after it's created
Immutable = can’t change once made
Think of it like writing on a whiteboard (mutable) vs carving something in stone (immutable).
🧱 Immutable Types (Stone Mode)
Examples:
int
,float
,str
,tuple
,bool
These stay the same no matter what. If you think you're changing them, Python is secretly making a new object in the background.
Example:
pythonCopyEdita = 10
b = a
b = b + 5
print(a) # Still 10
print(b) # 15
Memory behind the scenes:
Notice how b
points to a new place when we do b + 5
.
🔄 Mutable Types (Whiteboard Mode)
Examples:
list
,dict
,set
You can update them right there in memory. No copying or creating a new object.
Example:
pythonCopyEditx = [1, 2, 3]
y = x
y.append(4)
print(x) # [1, 2, 3, 4]
print(y) # [1, 2, 3, 4]
What’s going on?
Both x
and y
point to the same list — so changing one changes both!
🧠 Quick Hack: Use id()
to Peek at Memory
pythonCopyEdita = "hi"
b = a
print(id(a), id(b)) # Same memory address
b = b + " there"
print(id(a), id(b)) # Now different!
💡 TL;DR
Type | Mutable? | Examples |
Immutable | ❌ No | int, str, tuple |
Mutable | ✅ Yes | list, dict, set |
🎯 Final Thoughts
Understanding mutable and immutable objects helps you write smarter, cleaner code — and avoid bugs that’ll have you scratching your head.
So next time your variables start acting funny, remember:
🪨 Stone or 🧼 Whiteboard?
Subscribe to my newsletter
Read articles from abhishek Gaud directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by