Chintu’s Magical Shopping List and the Power of Python Lists
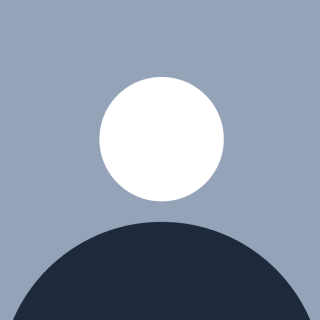

Previously on Episode 4...
We saw Chintu and Pintu explore D-Mart and experience the magic of structure and organization. Chintu had a lightbulb moment realizing how order makes life (and code) so much easier. From chaos to clarity—that’s the power of Data Structures.
But what happens when Chintu actually starts shopping?
Inside D-Mart – The Real Adventure Begins
chintu standing with Pintu in the snacks aisle.
Pintu: “Bro, let’s make a list. We always forget something and regret later.”
Chintu: “Good idea.”
He takes out his phone and opens the notes app.
Enter: The Python List
Just like how Chintu writes down a shopping list, Python programmers use lists to store items in one place.
A list in Python is like your digital trolley –
It holds multiple items, you can add more, remove some, check what's inside, and even rearrange them.
Example:
Chintu looks at the shopping list
shopping_list = ["Toothpaste", "Shampoo", "Chips", "Soap"]
He reads the list and starts picking items.
🧺 “ Toothpaste✅... Shampoo ✅...”
List Indexing - Like Aisle Numbers in D-Mart!
Every item had a position. just like how every section in D-Mart has an aisle number. In python that numbers or item position called index
In python two indexes are there:
Positive Indexes:
Negative Indexes:
Chintu’s Shopping List:
shopping_list = ["Toothpaste", "Soap", "Chips", "Maggie", "Bag","WaterBottle"]
Accessing Items Using Index
Let’s say Chintu wants to grab Chips from his list:
print(shopping_list[2]) # Output: Chips
What if Chintu Wants the Last Item?
No worries, Python’s got you!
print(shopping_list[-1]) # Negative indexing # Output: WaterBottle
Index Out of Range:
print(shopping_list[10]) # Output: IndexError: list index out of range
Just like you can't reach for aisle 50 if the store only has 10, Python won’t let you access indexes that don’t exist!
Basic List Operations — Let’s Learn With Chintu’s Shopping Journey
- Adding Items – Like grabbing new deals:
Chintu sees Maggie on sale and thought “Maggie is not in the list…but don’t worry just add it!
shopping_list = ["Toothpaste", "Shampoo", "Chips", "Soap"]
shopping_list.append("Maggie") # output: ["Toothpaste", "Shampoo", "Chips", "Soap","Maggie"]
.append()
adds a new item to the end of your list.
- Insert Items - Like insert new object: Chintu suddenly remembers Face Wash.
Oh! i forgot Face Wash. just add before the Maggie!
shopping_list = ["Toothpaste", "Shampoo", "Chips", "Soap","Maggie"]
shopping_cart.insert(4, "Face Wash") # output: ["Toothpaste", "Shampoo", "Chips", "Soap","Face Wash", "Maggie"]
.insert(position, item)
lets you place an item exactly where you want.
- Removing Items – If Chintu changes his mind:
shopping_list = ["Toothpaste", "Shampoo", "Chips", "Soap","Face Wash", "Maggie"]
shopping_list.remove("Chips") # output: ["Toothpaste", "Shampoo", "Soap","Face Wash", "Maggie"]
.remove(item)
deletes the first matching item.
- Checking Items – What’s at position 2?
shopping_list = ["Toothpaste", "Shampoo", "Soap","Face Wash", "Maggie"]
print(shopping_list[2]) # Soap
Access items by their position using []
brackets. notice one thing in python index start with 0.
- Updating Items – Replacing Soap with Body Wash:
shopping_list = ["Toothpaste", "Shampoo", "Soap","Face Wash", "Maggie"]
shopping_list[2] = "Body Wash" # ["Toothpaste", "Shampoo", "Body Wash","Face Wash", "Maggie"]
- Slicing the List – Only the first two items:
shopping_list = ["Toothpaste", "Shampoo", "Body Wash","Face Wash", "Maggie"]
print(shopping_list[:2]) # ["Toothpaste", "Shampoo"]
- Pop out - chintu thought remove shampoo at a position
shopping_list = ["Toothpaste", "Shampoo", "Body Wash","Face Wash", "Maggie"]
shopping_cart.pop(1) # output: ["Toothpaste", "Body Wash","Face Wash", "Maggie"]
.pop(index)
removes item at a position. If no index is given, it removes the last one.
- Cart Length Check - Chintu count how many items in trolly
shopping_list = ["Toothpaste", "Body Wash","Face Wash", "Maggie"]
len(shopping_cart) # output: 4
len()
tells you how many items are in your list.
- Sorting the List – Alphabetical shopping!
shopping_list = ["Toothpaste", "Body Wash","Face Wash", "Maggie"]
shopping_list.sort() # output: ["Body Wash","Face Wash", "Maggie", "Toothpaste"]
- Reverse the list - Chintu reverse list for fun
shopping_list = ["Body Wash","Face Wash", "Maggie", "Toothpaste"]
shopping_cart.reverse() # output: ["Toothpaste", "Maggie", "Face Wash", "Body Wash" ]
- Count - Chintu used this to count how many times an Maggie appears in his list
shopping_list = ["Maggie", "Body Wash","Face Wash", "Maggie", "Toothpaste", "Maggie"]
shopping_list.count("Maggie") # output: 3
Index - This helped Chintu find out at which position an Toothpaste is placed:
shopping_list = ["Body Wash","Face Wash", "Maggie", "Toothpaste"] shopping_list.index("Toothpaste")# output:3
Extend - His friend Mintu sent his shopping list too!
So, Instead of creating a whole new list, Chintu did something smart. he just merged both shopping lists using Python’s
extend()
method.# Chintu’s list shopping_list = ["Body Wash","Face Wash", "Maggie", "Toothpaste"] # Mintu’s list mintu_list = ["Shoes", "Chips", "Cheese"] # Merging Mintu’s items into Cintu’s list shopping_list.extend(mintu_list) print("Final Shopping List:", shopping_list) # Final Shopping List: ["Body Wash","Face Wash", "Maggie", "Toothpaste", "Shoes", "Chips", "Cheese"]
extend()
adds each element of another list individually to the end of the original list.
It's like putting all of Mintu’s items one by one into Chintu’s trolley.
Some Useful Methods:
max()
and min()
Used to find the largest or smallest item in a list.
numbers = [5, 2, 9, 1]
print(max(numbers)) # 9
print(min(numbers)) # 1
With key
argument:
words = ["apple", "banana", "kiwi"]
print(max(words, key=len)) # banana (longest word)
Nested Lists
Lists inside lists.
fruits = [
["Mango", "Apple"],
[3, 4]
]
print(matrix[0][0]) #Output: Mango
So far, we explored some of the most common and important list methods and operators in Python using Cintu's shopping adventure.
There are many more methods in the list world But hey! These you can explore like Cintu explores aisles in D-Mart
Real-World Coding Wisdom:
Chintu’s shopping list isn't just a list. it’s a live, editable, flexible tool to keep track of things.
That’s exactly what Python lists do in programming.
They are:
Ordered
Mutable (you can change them)
Can hold any type of data (even mix types!)
And just like that, our virtual trolley is filling up powered by Python lists!
We’ve seen how a real-world tool like a shopping list teaches us the power of storing, organizing, and modifying data in code.
Stay tuned for next blog,
Until then,
Stay curious. Keep learning. Keep Exploring
Subscribe to my newsletter
Read articles from Neha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by