Leverage Sealed Classes in Java: Use Cases, Best Practices, and Common Pitfalls
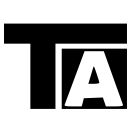
4 min read
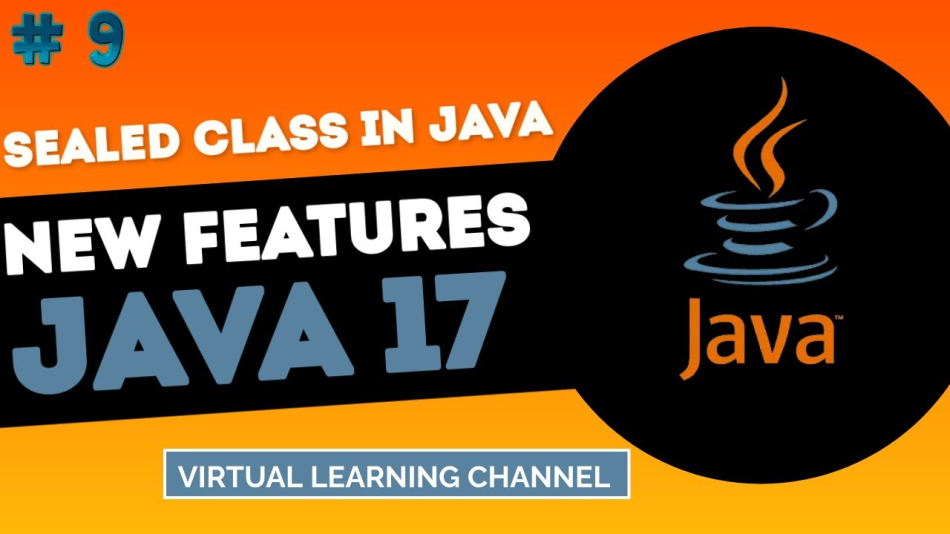
1. Understanding Sealed Classes in Java
Explain what Sealed Classes are and why they were introduced in Java 15 as a preview feature and then stabilized in Java 17. Mention how Sealed Classes allow you to define a restricted class hierarchy, specifying exactly which classes can extend or implement them.
1.1 What Are Sealed Classes?
Dive into the concept. Describe how Sealed Classes in Java enforce restrictions on which classes or interfaces can extend them. Here, introduce permits keyword and explain the final, non-sealed, and sealed modifiers that can be used by the subclasses.
1.2 Basic Example of Sealed Classes
Provide a simple code example to illustrate how a Sealed Class works:
public abstract sealed class Shape permits Circle, Rectangle, Triangle {
// Common properties and methods for shapes
}
public final class Circle extends Shape {
// Circle specific properties and methods
}
public final class Rectangle extends Shape {
// Rectangle specific properties and methods
}
public final class Triangle extends Shape {
// Triangle specific properties and methods
}
Explain each part of the code in detail, particularly the use of permits to restrict which classes can extend Shape.
2. Advantages of Using Sealed Classes
Discuss the main advantages that Sealed Classes offer, such as stronger encapsulation, more maintainable codebases, and enabling the compiler to make assumptions about class hierarchies.
Better Control Over Inheritance
Explain how Sealed Classes help you control inheritance, making it explicit which classes can extend a specific class. This control can be valuable in frameworks and APIs where you want to restrict the possible implementations to a defined set.
Enhanced Code Readability and Maintenance
Go into detail on how restricting inheritance pathways can make it easier for developers to understand class hierarchies and ensure consistent implementations of base class methods. Share some examples of where this can be beneficial in real-world applications, such as modeling finite states or specific types of domain objects.
3. Use Cases for Sealed Classes
Describe some practical scenarios where Sealed Classes can be particularly useful. Discuss areas such as modeling specific business domain models or enforcing certain types of access patterns in APIs.
Modeling Domain-Specific Types
Use an example related to a domain-specific scenario, such as a role-based access control system, where certain roles can inherit from a base role class, but others are restricted.
Implementing State Machines
Illustrate how Sealed Classes can model states with a finite set of transitions, where each transition state can only be represented by an explicitly permitted subclass. Provide a code example where states like Active, Inactive, and Suspended inherit from a UserState Sealed Class.
4. Best Practices for Using Sealed Classes
List and describe best practices, emphasizing how to structure Sealed Classes for optimal readability, testability, and performance.
Align Sealed Class Structure with Your Domain Model
Explain how Sealed Classes can align with your application’s domain model. Share some patterns for structuring Sealed Classes and common pitfalls, such as creating overly complex hierarchies that can reduce the readability of your code.
Use Sealed Classes to Optimize Compiler Checks
Discuss how Java’s compiler can make assumptions and optimizations when Sealed Classes are used. Explain how this can improve performance in some cases, while also ensuring that unanticipated subclasses don’t break your application logic.
5. Common Pitfalls and Challenges
Identify some potential issues, such as complexity and readability challenges, when implementing Sealed Classes.
Avoid Over-Restricting Class Hierarchies
Mention that Sealed Classes should be used judiciously and discuss potential drawbacks when used to over-restrict class hierarchies. Highlight the importance of keeping your class hierarchy as simple as possible and using Sealed Classes only when you have a clear set of subclasses that need to extend your base class.
Transitioning from Sealed to Non-Sealed or Final Classes
Explain what happens when you decide to change a Sealed Class to a non-sealed or final class and how that impacts the existing codebase. Discuss compatibility and maintenance considerations, especially when working in collaborative or open-source projects.
6. Conclusion
Summarize the advantages of Sealed Classes and encourage readers to experiment with them for specific use cases, mentioning how these can improve the clarity and maintainability of their Java code. Invite readers to comment on any questions they have about using Sealed Classes or how they might fit into their current projects.
This structure should help guide the content while ensuring it adheres to your preferences for clarity, depth, and engagement. Let me know if you’d like more details on any specific section!
Read more at : Leverage Sealed Classes in Java: Use Cases, Best Practices, and Common Pitfalls
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
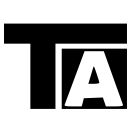
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.