Solving LeetCode 2444: Count Subarrays With Fixed Bounds Using Sliding Window π
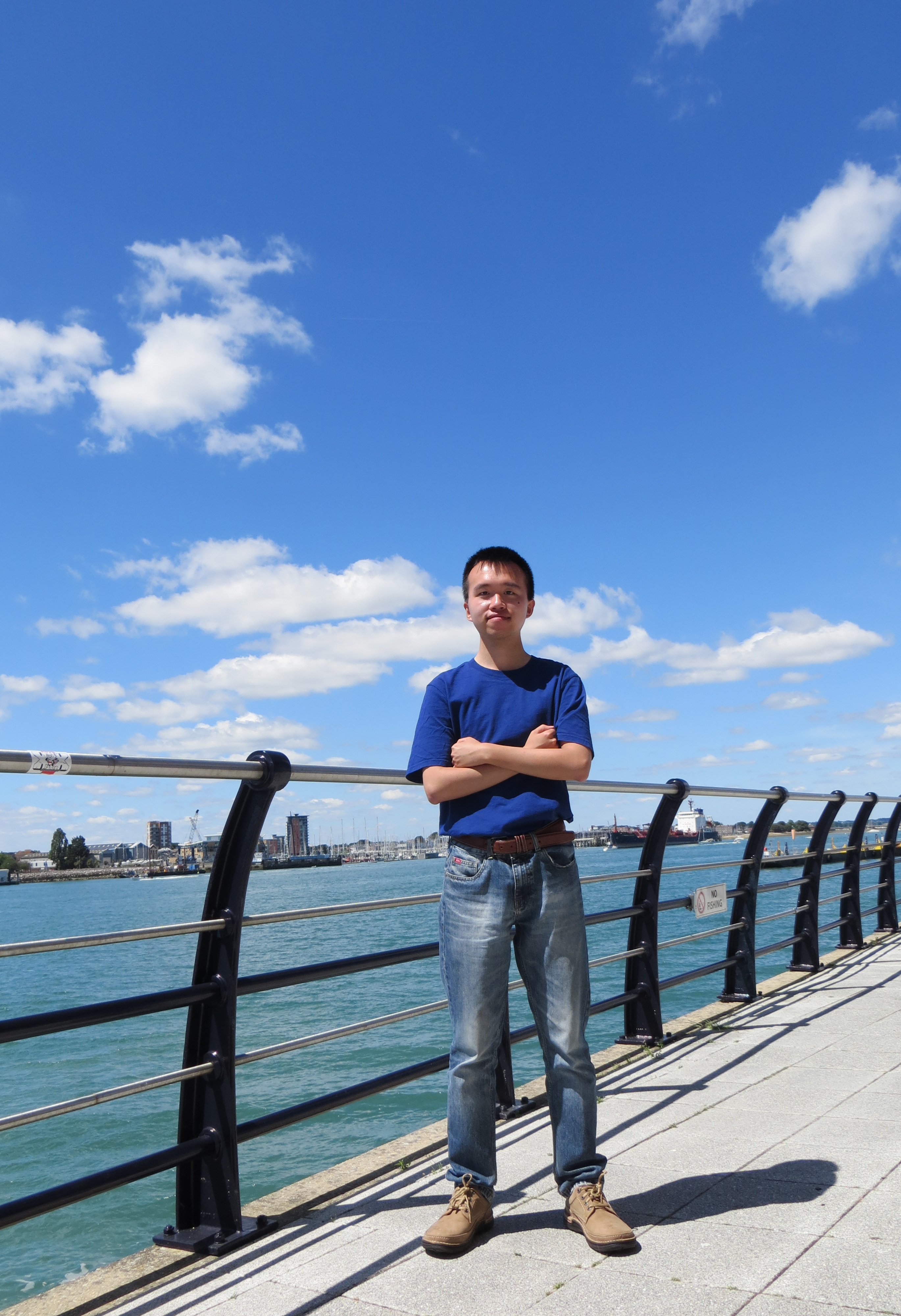

Introduction π
π’ Have you counted patterns from a list of numbers?
π‘οΈ Imagine you are counting the stretches of days with freezing temperatures or heat waves, thatβs the problem I solved recently from LeetCode - 2444: Count Subarrays with Fixed Bounds.
πͺ In this blog, I will apply a sliding window algorithm to solve this problem with a real-world example to highlight how you can demonstrate critical thinking.
π§© Problem Breakdown
Given:
An array of numbers,
nums
.Two integers:
minK
andmaxK
.
Determine how many subarrays contain both:
A minimum value equal to
minK
.A maximum value equal to
maxK
.
For example,
Input: nums = [1,3,5,2,7,5], minK = 1, maxK = 5
Output: 2
Explanation: The fixed-bound subarrays are [1,3,5] and [1,3,5,2].
π Sliding Window Algorithm
A sliding window explores each subarray through the following steps:
Iterate through
nums
to track the last positions ofminK
,maxK
, and the invalid numbers.For each index
i
, calculate how many valid subarrays there are.Accumulate this index for every element in the list,
nums
.
π» Java Implementation
class Solution {
public long countSubarrays(int[] nums, int minK, int maxK) {
long count = 0;
int start = -1, mini = -1, maxi = -1;
for (int i = 0; i < nums.length; i++) {
if (nums[i] < minK || nums[i] > maxK) {
start = i;
}
if (nums[i] == minK) {
mini = i;
}
if (nums[i] == maxK) {
maxi = i;
}
int valid = Math.max(0, Math.min(mini, maxi) - start);
count += valid;
}
return count;
}
}
β Time Complexity: O(n)
, a single pass through the array.
π Space Complexity: O(1)
, constant extra spaces.
πReal-life Example
π Scenario: Tracking health vitals
π« Imagine that you are monitoring your heart rate daily. Some days, your heart rate dips too low or spikes too high. You want to count how many continuous days your heart rate remains within the minimum acceptable rate (e.g., 90 bpm) and the maximum acceptable rate (e.g., 170 bpm). A sliding window approach can track your heart rate over the month.
π Why does this approach work?
This allows examining multiple conditions while preventing backtracking to recheck the same subarrays. Smart tracking monitors each index and enhances algorithm optimisation, making it superior to its brute force counterparts.
π Conclusion
Sliding window simplifies complex problems, whether you are working on your interview preparations or analysing real-world data. They can save both time and effort.
Subscribe to my newsletter
Read articles from Haocheng Lin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
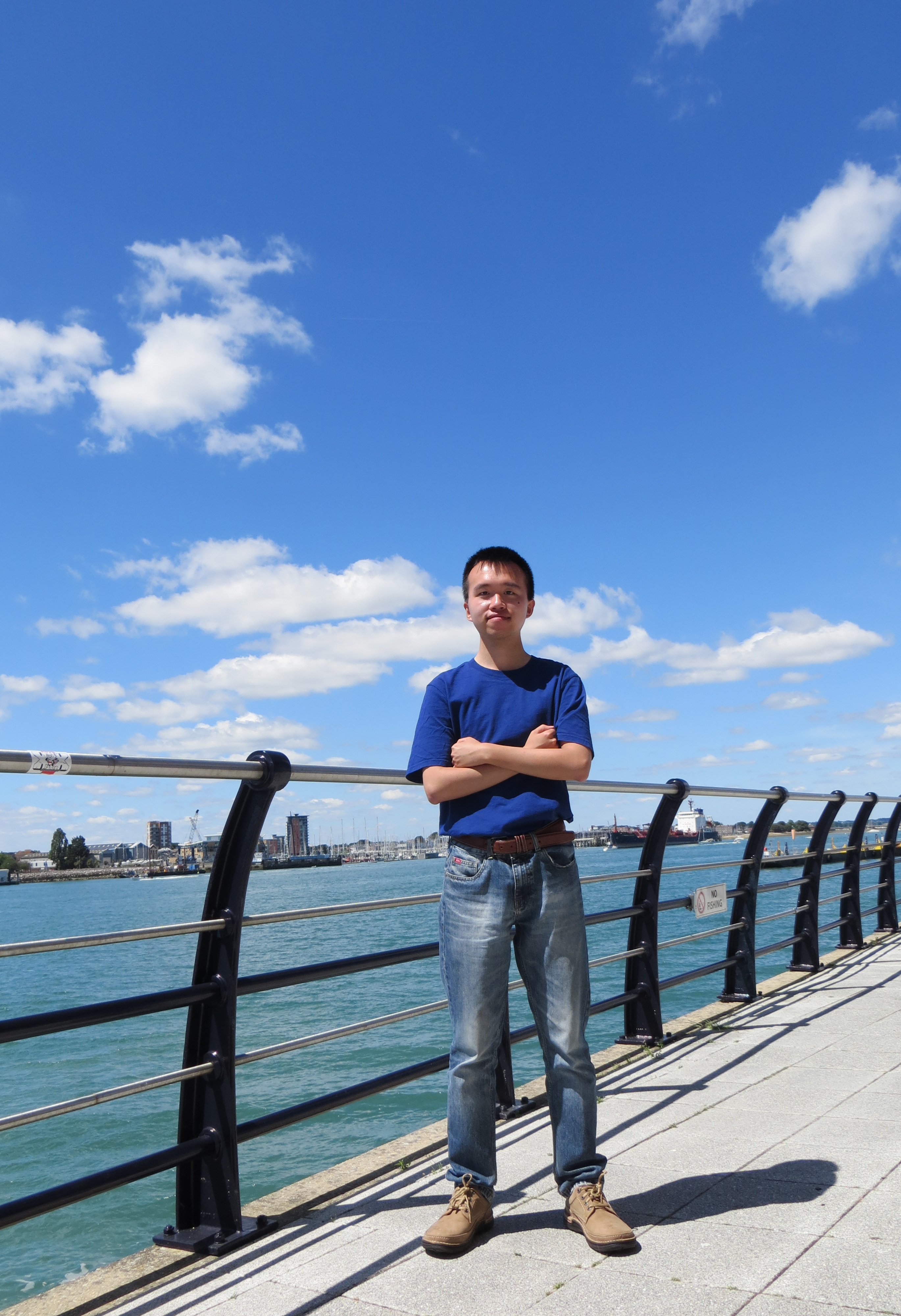
Haocheng Lin
Haocheng Lin
π» AI Developer & Researcher @ Geologix ποΈ UCL MEng Computer Science (2019 - 23). ποΈ UCL MSc AI for Sustainable Development (2023 - 24) π₯ Microsoft Golden Global Ambassador (2023 - 24)π