Dynamic Props in React.js
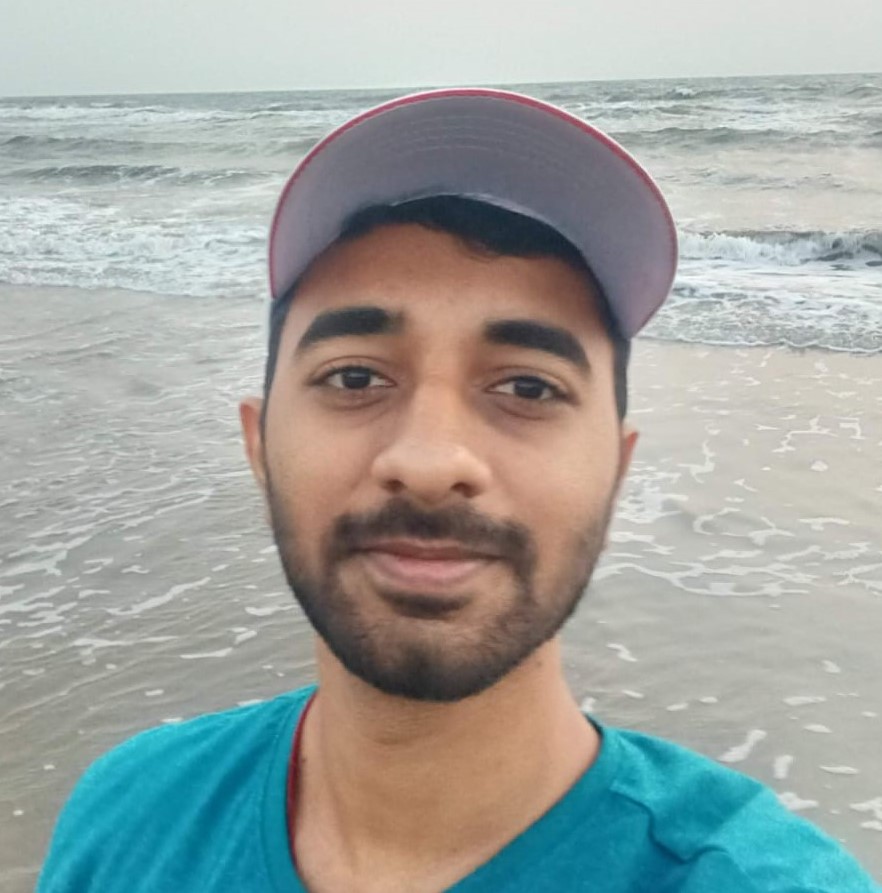

Using &&
inside a prop ❌ (can send false
)
type MyComponentProps = {
desc?: string;
};
const MyComponent = ({ desc }: MyComponentProps) => {
return <div>Desc is: {desc}</div>;
};
export default function App() {
const format = 'email'; // not 'phone'
const item = { code: '+91' };
return (
<div>
<MyComponent desc={format === 'phone' && item.code} />
</div>
);
}
Output:
Desc is: false
This happens because format === 'phone'
is false
so desc={false}
Using ternary ✅ (proper behavior)
<MyComponent desc={format === 'phone' ? item.code : undefined} />
Output:
Desc is:
Clean, nothing rendered — no "false"
weirdness!
When dealing with multiple props
const props = {
desc: format === 'phone' ? item.code : undefined,
image: format === 'phone' ? item.flag : undefined,
title: item.name,
disabled: isDisabled ? true : undefined,
};
<MyComponent {...props} />
If we want to avoid even undefined
keys, we can build props conditionally:
const props = {
...(format === 'phone' && { desc: item.code }),
...(format === 'phone' && { image: item.flag }),
title: item.name,
...(isDisabled && { disabled: true }),
};
This way, if a condition fails, that prop is not even created
Utility for all dynamic props
We can even have a generic dynamic object builder using Object.entries
:
function buildProps<T extends object>(source: T, keys: (keyof T)[]): Partial<T> {
const result: Partial<T> = {};
keys.forEach((key) => {
if (source[key] !== undefined && source[key] !== null) {
result[key] = source[key];
}
});
return result;
}
Usage:
const personalInfoProps = buildProps(user, ['firstName', 'lastName', 'dateOfBirth']);
Subscribe to my newsletter
Read articles from Yogesh Kanwade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
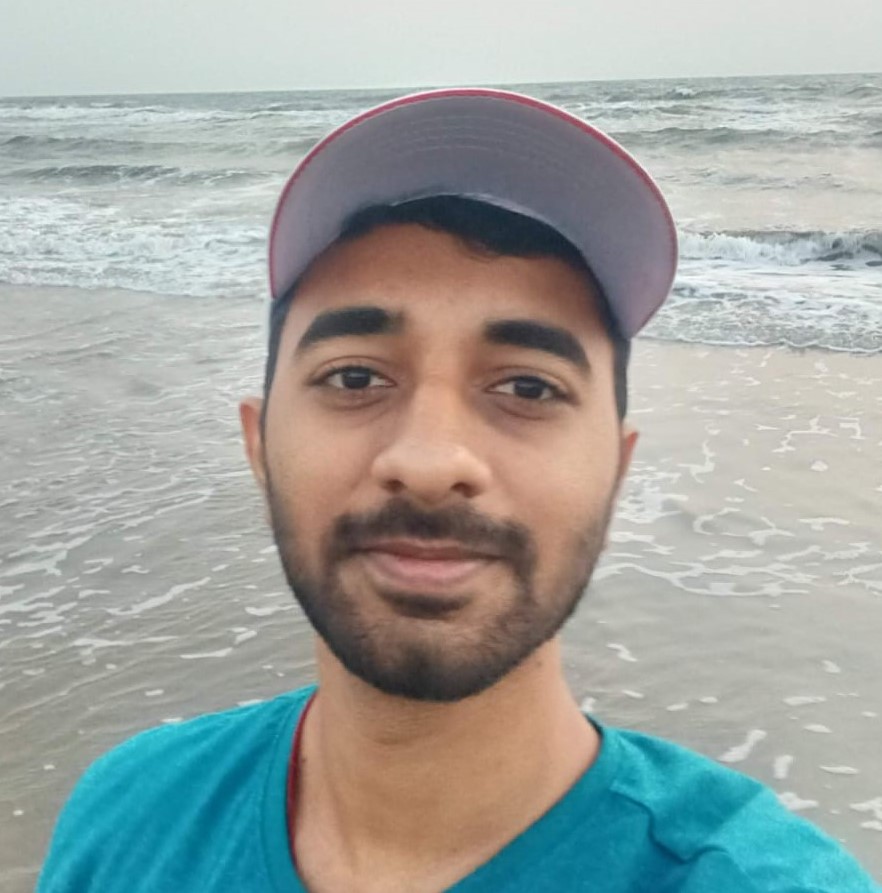
Yogesh Kanwade
Yogesh Kanwade
I'm Yogesh Kanwade, a final year Computer Engineering student with a deep passion for software development. I am a continuous learner, with hardworking and goal-driven mindset and strong leadership capabilities. I am actively exploring the vast possibilities of Web Development along with AWS and DevOps, fascinated by their impact on scalable and efficient web solutions.