Day 1 - 1Z0-829
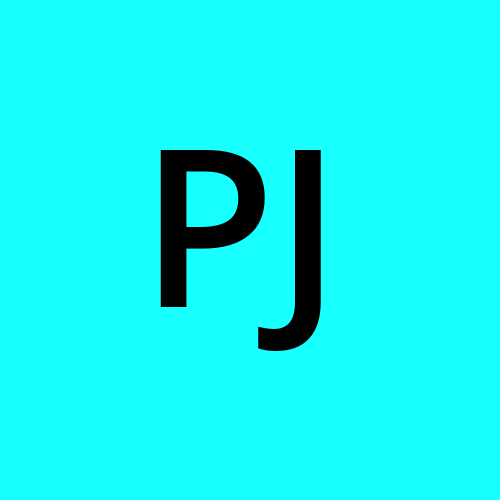

sudo apt install openjdk-17-jdk -y
The above command installs java 17 in Ubuntu
Naming Conflicts
java.util.Date
and java.sql.Date
Explicit mention always gets the precedence over any wildcard
If you want to use both, then use a fully qualified name like this may be:
public class Conflicts { java.util.Date date; java.sql.Date date; }
Argument Options
Compiling with Jar file
on Win:
java -cp ".;C:\temp\someOtherLocation;c:\temp\myJar.jar" myPackage.MyClass
on Unix based:
java -cp ".:/tmp/someOtherLocation:/tmp/myJar.jar" myPackage.MyClass
In the above commands,
.
- means to look for class files in the current dir:
- used in Unix based to separate paths;
- used by Win to separate paths
Order of Initialization
Fields → Initializer Block → Constructor
Initializer block cannot initialize a field before its declaration → ❌Doesn’t Compile
Data Types
Primitive
Without
l/L
all non-decimals areint
Without
f/F
all the decimals aredouble
Bit size of
boolean
depends on the JVM
Literals:
double notAtStart = _100.00; // Doesn't Compile double notAtEnd = 10000.00_; // Doesn't Compile double notByDecimal = 10_.5; // Doesn't Compile double rufrbro = 1_0_0.0__0; // Doesn't Compile double idgafnow = 6_______9; // Doesn't Compile
Wrapper Class:
WRAPPER_CLASS.valueOf($PRIMITIVE_VALUE)
→ Create the Wrapper Objectint primitive = Integer.parseInt("123");
→ Conv String to int primitiveInteger wrapper = Integer.valueOf("123");
→ Conv String to Integer (Wrapper of int)
Text Blocks
- Example:
String block = """
"doe\"\"\"
\"deer\"""
""";
System.out.print("*" + block + "*");
// The Output is:
* "doe"""
"deer"""
*
- Note: Text blocks require a line break after the opening
"""
P. S.
Any variable used inside a text block is a string and not a variable
var
is not a reserved wordAll Wrapper Classes defaults to null
boolean defaults to false
There is no default value for method params
Subscribe to my newsletter
Read articles from Priyan J directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
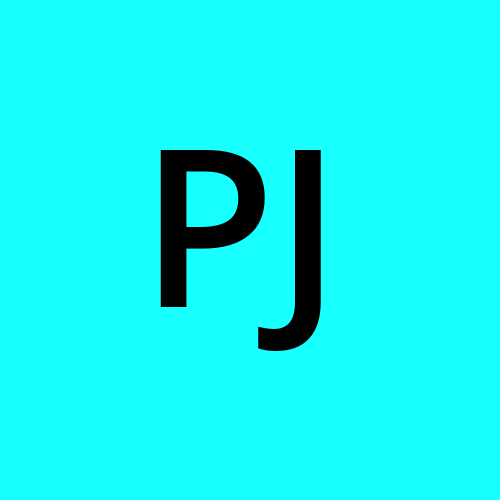