Exploring Spring ORM with Hibernate: A Beginner's Perspective
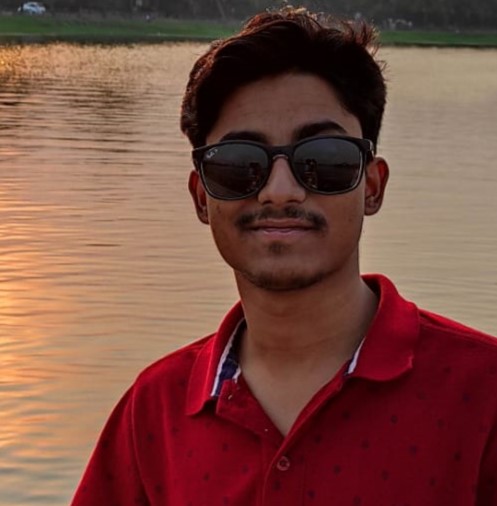

Hey everyone! π
This week was all about diving deep into Spring ORM (Object-Relational Mapping) using Hibernate β and honestly, it felt like unlocking another big puzzle piece in my Spring Framework learning journey. π
Coming from handling basic JDBC operations last week, moving to ORM felt both exciting and a little overwhelming at first β but once I understood the why behind it, everything started falling into place!
Hereβs a complete breakdown of my learning experience β straight from a student/learner's perspective. Let's go! π¨βπ»
π What is Spring ORM (and Why Hibernate)?
Before getting into Spring ORM, I first had to understand what ORM actually is.
In simple terms:
ORM (Object-Relational Mapping) is a technique that allows us to map Java objects (classes) to database tables β without writing messy SQL all the time.
Hibernate is one of the most popular ORM frameworks β and Spring integrates it beautifully through Spring ORM.
The biggest advantage?
β
I can now work with Java classes instead of worrying about manual SQL queries, result sets, and error-prone JDBC code.
β
Less boilerplate, more business logic. π―
π₯ What I Learned This Week
1. Setting Up Hibernate with Spring
The first thing I learned was how to configure Hibernate with Spring:
Adding Hibernate + MySQL dependencies.
Configuring
SessionFactory
bean.Setting up
hibernate.cfg.xml
or Java-based Hibernate configurations.Creating an Entity class (POJO) mapped to a database table.
Key takeaway: Spring makes Hibernate integration so much smoother compared to doing it manually!
2. Entity Classes and @Entity Annotation
In Hibernate, classes are mapped to database tables using annotations like:
@Entity
@Table(name = "student")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String name;
}
No more writing SQL to create or map tables manually (at least for simple use cases)!
3. Basic CRUD with Hibernate + Spring ORM
Of course, just setting things up isnβt enough.
So, I practiced full CRUD operations:
Create β Saving new students into the database.
Read β Fetching student records.
Update β Updating details like name, age, etc.
Delete β Removing student records.
The cool thing? All done through Hibernate's Session API, but managed via Spring's dependency injection. π€―
4. Session and Transaction Management
One thing that confused me initially was how Hibernate sessions and transactions are managed.
After working on a few examples, I understood:
Open a
Session
fromSessionFactory
.Begin a
Transaction
.Perform operations (save, update, delete).
Commit or rollback if something goes wrong.
Spring takes care of a lot of boilerplate here, especially if we use declarative transaction management (@Transactional
).
5. Hands-on Mini Project: Student-Book Management Console App π
Instead of just writing random CRUD methods, I decided to build something simple to put everything I learned into practice:
β‘οΈ Console-based Student Record Management System
Add new students.
Update student details.
Delete student records.
View all students.
Nothing fancy β just a pure console app β but it helped me connect all the dots and realize where Hibernate ORM truly shines! π
π Code Repositories (All Hands-On Practice)
All the codes I practiced this week are available publicly on GitHub:
πΈ Spring ORM Practice Repo
(Where I practiced configurations, CRUD, and Hibernate basics)
π https://github.com/Arkadipta-Kundu/springORMusingHibernate
πΈ Student-Book Management Console Project
(Where I applied ORM concepts in a mini real-world style project)
π https://github.com/Arkadipta-Kundu/student_book
If youβre learning Spring ORM too, feel free to check them out!
I kept them clean and beginner-friendly. π¬
π Whatβs Next for Me?
Now that I have a basic understanding of how Spring integrates with ORM and Hibernate, itβs time to move up the ladder!
π Next Target:
Learning Spring MVC (Model-View-Controller)
β Building web apps with Spring.
β Understanding Controllers, Views, Models in-depth.
Super excited because now I'll finally start working on web-based backend development in Spring! π―
π₯ In a Nutshell (TL;DR)
Learned about Spring ORM and Hibernate for database operations.
Understood Entity mapping, Session management, and Transaction handling.
Practiced full CRUD operations using Hibernate + Spring.
Built a mini console project to apply learnings practically.
All code is available on GitHub (links above!).
Next goal β Spring MVC and web application development! π
Thanks a lot for reading my learning journey so far! π
If youβve got any tips, resources, or projects related to Spring MVC β feel free to share them with me! Would love to hear from you and learn more! π―
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
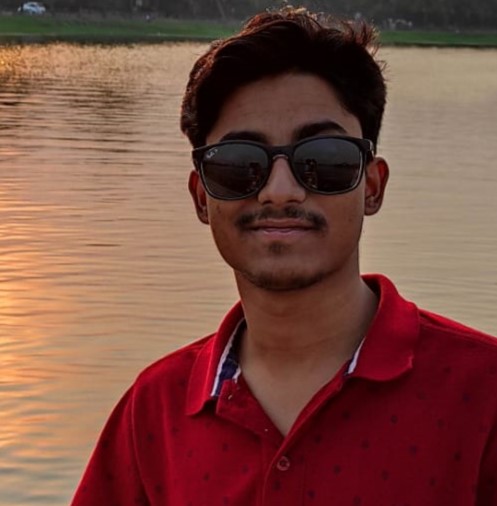
Arkadipta Kundu
Arkadipta Kundu
Iβm a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, Iβm focused on sharpening my DSA skills and expanding my expertise in Java development.