PostgreSQL CASE Explained: Your Guide to Smart SQL Logic
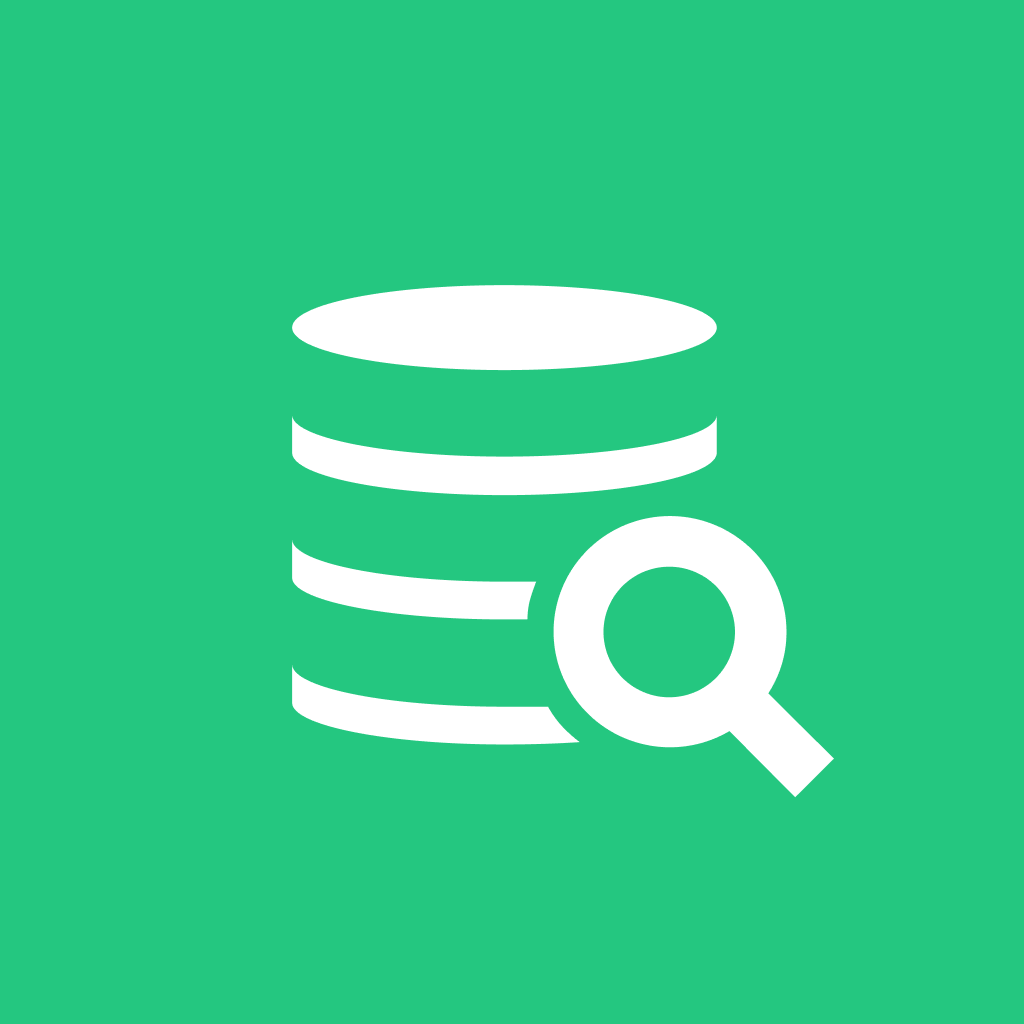

Writing smarter queries in PostgreSQL often means applying conditions directly inside your SQL. That’s where the CASE
statement comes in. This tool allows you to transform data based on rules, making your queries more adaptable.
Syntax and Examples
There are two core types of CASE
statements:
Simple CASE
Used when you're comparing a column to several fixed values:
SELECT product_name,
CASE product_category
WHEN 'Electronics' THEN 'Tech'
WHEN 'Clothing' THEN 'Apparel'
ELSE 'Other'
END AS category_type
FROM products;
Searched CASE
When conditions are more complex:
SELECT order_id, order_quantity,
CASE
WHEN order_quantity > 100 THEN 'Bulk'
WHEN order_quantity > 50 THEN 'Mid-size'
ELSE 'Small'
END AS order_type
FROM orders;
Real-World Use Cases
Grouping Customers
SELECT customer_name,
CASE
WHEN total_purchases > 1000 THEN 'VIP'
WHEN total_purchases > 500 THEN 'Loyal'
ELSE 'New'
END AS customer_status
FROM customers;
Conditional Sorting
SELECT employee_name, job_title
FROM employees
ORDER BY
CASE job_title
WHEN 'CTO' THEN 1
WHEN 'Developer' THEN 2
ELSE 3
END;
Handling Missing Data
SELECT item_name,
CASE
WHEN stock_count IS NULL THEN 0
ELSE stock_count
END AS inventory
FROM stock;
FAQs
Can I use CASE inside WHERE?
Absolutely. It helps build flexible filters based on conditions.
What if no WHEN condition matches?
It returns NULL
unless you include an ELSE
.
Are CASE statements performance heavy?
Not usually, but watch complexity in large queries.
Can I use CASE with aggregates like COUNT()?
Yes! It’s great for conditional aggregation.
Conclusion
PostgreSQL's CASE
logic is one of the most underrated SQL features. It gives you full control over how data is presented, sorted, or filtered. Whether you're working on reports or apps, it brings flexibility with minimal overhead.
For deeper examples and tips, visit the full guide: PostgreSQL CASE: A Comprehensive Guide.
Subscribe to my newsletter
Read articles from DbVisualizer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
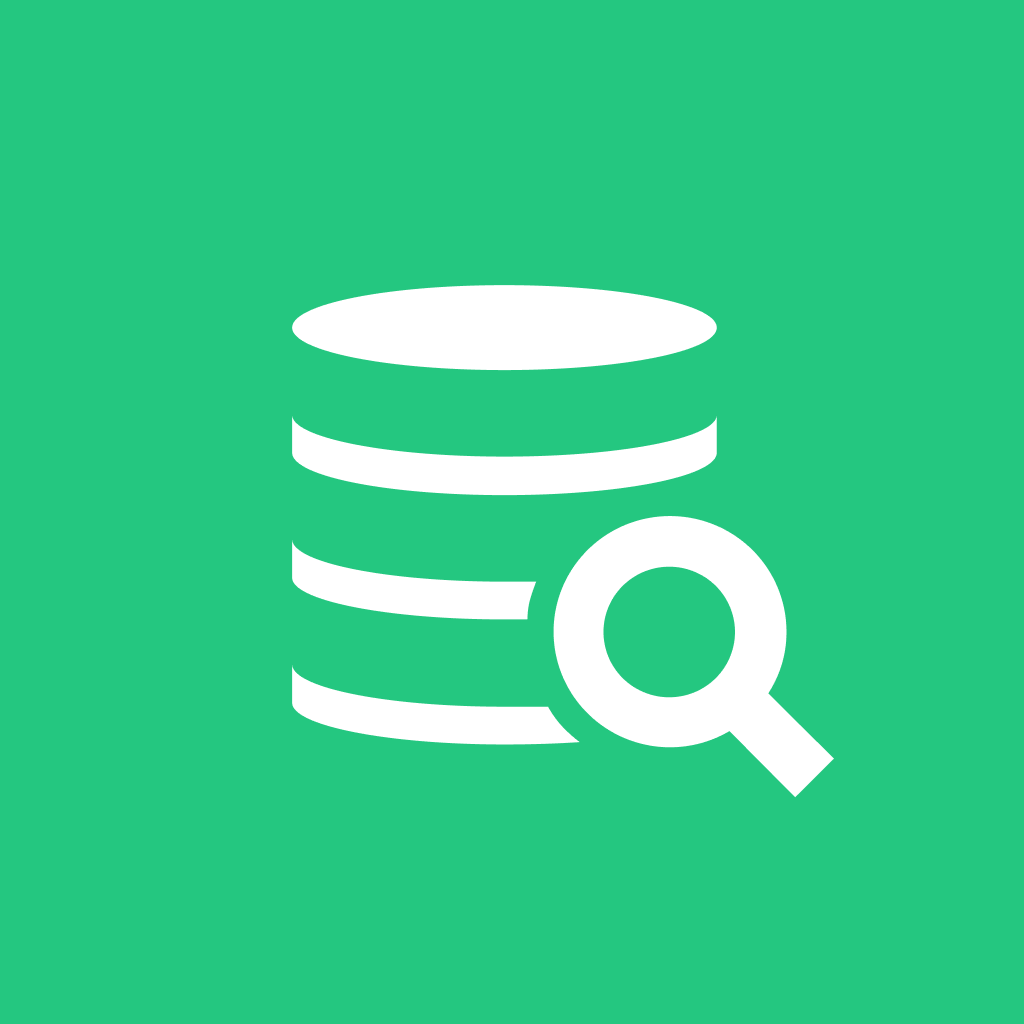
DbVisualizer
DbVisualizer
DbVisualizer is the database client with the highest user satisfaction. It is used for development, analytics, maintenance, and more, by database professionals all over the world. It connects to all popular databases and runs on Win, macOS & Linux.