Mastering Data Flow and State Management in Flutter: A Practical Guide for React Developers
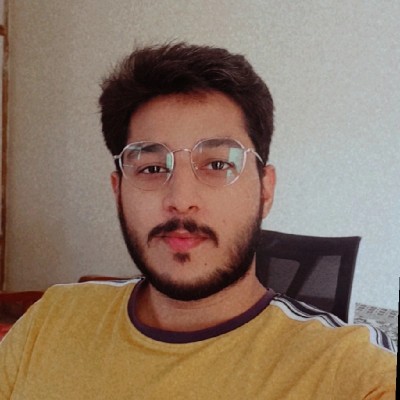

Introduction
Flutter has rapidly become one of the most popular frameworks for building cross-platform mobile applications. If you’re coming from a React or Angular background, many of its concepts—widgets, immutable state, and one-way data flow—will feel familiar. Yet Flutter introduces its own idioms, particularly around state management and widget lifecycles. In this post, we’ll explore how to:
Pass data down from parent to child widgets
Send data up from child to parent
Manage local widget state with
StatefulWidget
andsetState
Handle app-wide state using the
Provider
package
Throughout, you’ll find concise code snippets and React-to-Flutter analogies that make the transition smooth.
Passing Data from Parent to Child in Flutter
In React you pass props; in Flutter you pass constructor parameters. Every widget is just a class, so to hand immutable data to a child you:
Declare a
final
field on the child.Require it via the constructor.
Supply the value when instantiating.
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
//Parent
class MyApp extends StatelessWidget {
const MyApp();
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: HomeScreen(title: 'Welcome to Flutter!'), // child 1 constructor call
);
}
}
// Child 1
class HomeScreen extends StatelessWidget {
final String title;
const HomeScreen({required this.title});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text(title)),
body: const Center(
child: Greeting(message: 'Hello from the child!'), // child 2 Constructor call
),
);
}
}
//child 2
class Greeting extends StatelessWidget {
final String message;
const Greeting({required this.message});
@override
Widget build(BuildContext context) {
return Text(
message,
style: const TextStyle(fontSize: 24),
);
}
}
Analogy:
Greeting
’smessage
field is like a React prop—immutable, passed in by its parent.Tip: Always mark these fields
final
to enforce immutability and optimize rebuilds.
Passing Data Up (Child → Parent)
In React you’d hand a function down via props so the child can “call back.” Flutter does the same with callback types:
Parent defines a function that takes the data it needs.
Parent passes that function to the child.
Child invokes the callback when appropriate.
class HomeScreen extends StatefulWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
State<HomeScreen> createState() => _HomeScreenState(); // create the state to later update
}
class _HomeScreenState extends State<HomeScreen> {
String _feedback = 'No feedback yet'; // state variable value
void _handleFeedback(String text) { // define the function that is to be passed
setState(() {
_feedback = text;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Child → Parent Demo')),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
FeedbackInput(onSubmit: _handleFeedback), // call child and pass the function reference
const SizedBox(height: 20),
Text(_feedback, style: const TextStyle(fontSize: 18)),
],
),
);
}
}
class FeedbackInput extends StatelessWidget {
final void Function(String) onSubmit; //explaination ahead
final TextEditingController _controller = TextEditingController();
FeedbackInput({required this.onSubmit, Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
SizedBox(
width: 200,
child: TextField(controller: _controller),
),
IconButton(
icon: const Icon(Icons.send),
onPressed: () {
onSubmit(_controller.text);
_controller.clear();
},
),
],
);
}
}
Key points:
The parent holds the mutable state (
_feedback
).It passes
_handleFeedback
down asonSubmit
.The child simply calls
onSubmit(...)
when the user acts.
Lets take a moment to understand — “ void Function(String) onSubmit(); ”
let's break it down carefully.
final void Function(String) onSubmit;
This line declares a field inside a Dart class (most likely a StatelessWidget
or StatefulWidget
), and it means:
Part | Meaning |
final | This variable must be assigned exactly once. (immutable) |
void Function(String) | A type definition: it’s a function that takes a String and returns nothing (void ). |
onSubmit | The name of the variable — you can call this function later. |
In simple words:
onSubmit
is a callback function.It expects a
String
as its input.It returns nothing (
void
).final
means you can assign it once (usually via constructor), and then just use it.
Example to visualize it:
Suppose you have this widget:
class FeedbackInput extends StatelessWidget {
final void Function(String) onSubmit; // <-- THIS
FeedbackInput({required this.onSubmit});
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: () {
onSubmit("Some user feedback"); // <-- Call the callback
},
child: const Text('Submit Feedback'),
);
}
}
And in the parent widget, you use it like this:
FeedbackInput(
onSubmit: (String feedbackText) {
print('User submitted: $feedbackText');
},
)
When the button is pressed,
onSubmit
will be called with a String.That String (e.g., user feedback) is passed up to the parent.
Quick React analogy:
If you come from a React background, void Function(String) onSubmit
is conceptually exactly like:
function FeedbackInput({ onSubmit }) {
return <button onClick={ () => onSubmit("some feedback") }>Submit</button>;
}
Why Flutter likes it this way:
Type safety: Dart knows exactly what kind of function
onSubmit
must be. (takes aString
, returnsvoid
).Cleaner error checking during compile time.
Summary:
➡️ final void Function(String) onSubmit;
defines a final, strongly-typed function pointer that expects a String
argument and returns nothing.
Now lets move on!
Local State Management with setState
Whenever a widget needs to manage its own transient state—like toggling a button or tracking input focus—wrap it in a StatefulWidget
and call setState
to trigger a rebuild.
class CounterButton extends StatefulWidget {
const CounterButton({Key? key}) : super(key: key);
@override
State<CounterButton> createState() => _CounterButtonState();
}
class _CounterButtonState extends State<CounterButton> {
int _count = 0;
void _increment() {
setState(() {
_count += 1;
});
}
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: _increment,
child: Text('Clicked $_count times'),
);
}
}
setState
takes a closure in which you mutate your state; Flutter then efficiently re-runsbuild
.Performance tip: Keep the
setState
scope as narrow as possible to avoid unnecessary rebuilds.
Handling App-Wide State with Provider
For larger apps, passing props and callbacks through many widget levels becomes cumbersome. Enter Provider, Flutter’s lightweight dependency-injection and state-notification solution.
Define a model by extending
ChangeNotifier
:// lib/models/counter_model.dart import 'package:flutter/foundation.dart'; class CounterModel extends ChangeNotifier { int _count = 0; int get count => _count; void increment() { _count++; notifyListeners(); } }
Provide it at the top of your app:
void main() => runApp( ChangeNotifierProvider( create: (_) => CounterModel(), child: const MyApp(), ), );
Consume it anywhere below:
class CounterScreen extends StatelessWidget { const CounterScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { final count = context.watch<CounterModel>().count; return Scaffold( appBar: AppBar(title: const Text('Provider Example')), body: Center( child: Text('Count: $count', style: const TextStyle(fontSize: 32)), ), floatingActionButton: FloatingActionButton( onPressed: () => context.read<CounterModel>().increment(), child: const Icon(Icons.add), ), ); } }
.watch<T>()
rebuilds whennotifyListeners()
fires..read<T>()
fetches without subscribing.This pattern decouples state from UI and avoids “prop drilling” through deep trees.
Conclusion & Next Steps
You’ve seen the core patterns of Flutter’s data flow:
Downward via constructor parameters
Upward via callback functions
Local via
StatefulWidget
+setState
Global via
ChangeNotifier
+Provider
To continue leveling up:
Explore selectors and Consumer widgets for more granular rebuilds.
Experiment with multi-provider setups for complex apps (e.g., auth + theming + domain data).
Compare Riverpod, BLoC, or Redux if your app demands advanced state orchestration.
Integrate Futures and Streams to manage asynchronous data (API calls, WebSockets).
Feel free to fork these snippets into a sample Flutter project. As you build, refer back to React analogies when in doubt—Flutter’s composable widgets and unidirectional data flow will start to feel second nature. Happy coding!
Subscribe to my newsletter
Read articles from Waleed Javed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
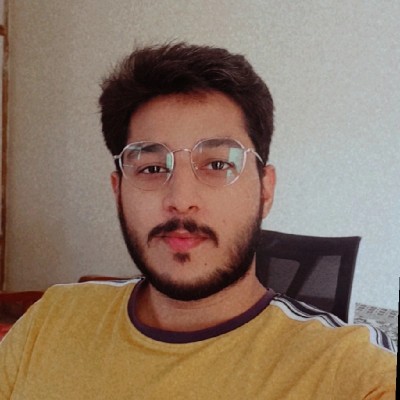
Waleed Javed
Waleed Javed
Building Enterprise Softwares🚀 | Sharing insights on Linkedin/in/Waleed-javed