Mastering Multi-tab communication - Part 1
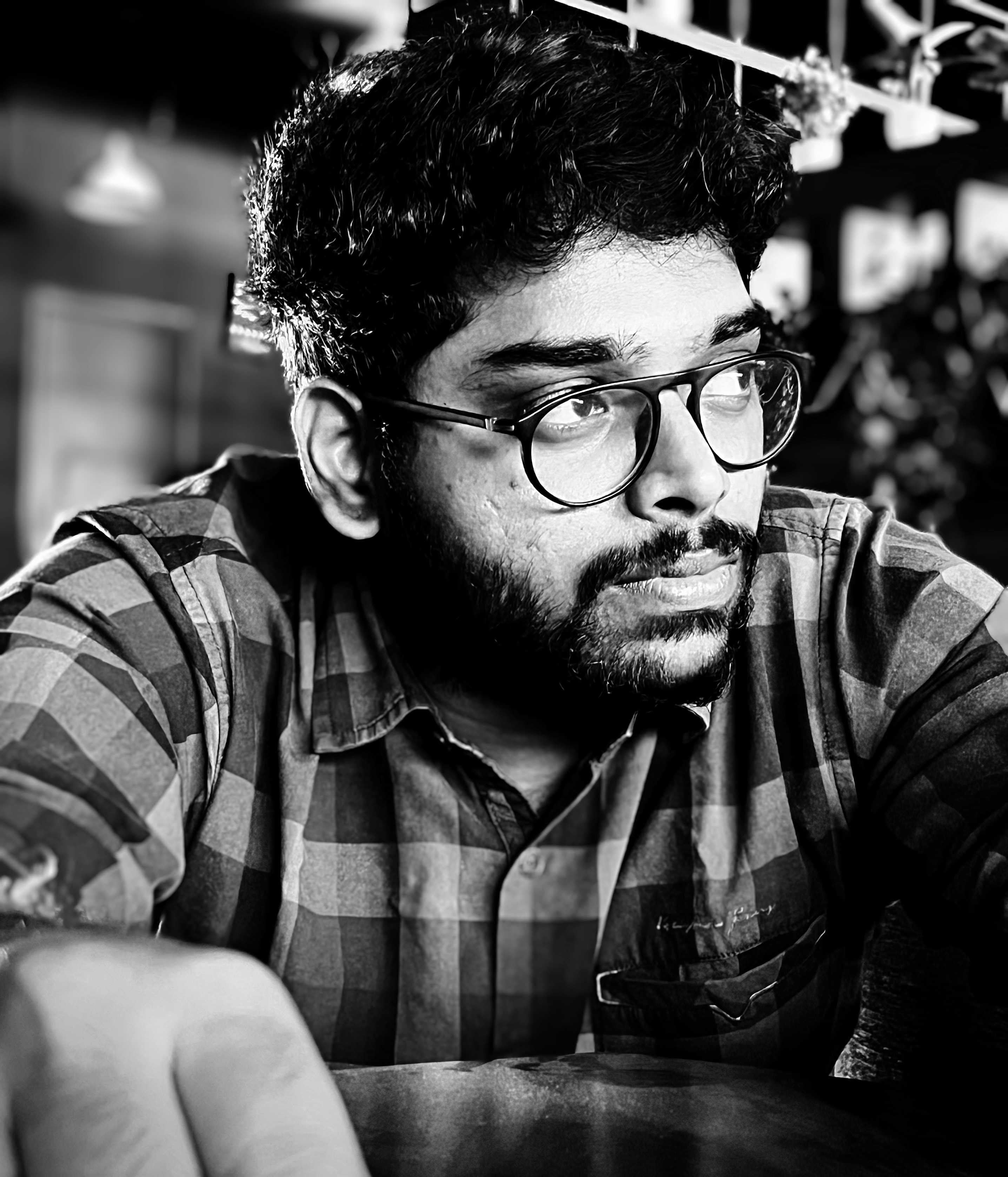
👋 Introduction
Have you ever opened the same app in multiple tabs and wished they could "talk" to each other?
Maybe you logged out in one tab and expected all other tabs to log out too. Or you wanted a single notification alert to appear across tabs.
Before today, solving this problem meant either complicated setups (like WebSockets) or hacky tricks (like using localStorage
events).
Luckily, browsers gave us a clean, simple, native solution:
✨ The BroadcastChannel API ✨
In this article, we’ll explore what BroadcastChannel is, why it exists, and when to use it — even if you're completely new to this topic!
📚 What is the BroadcastChannel API?
At its core, the BroadcastChannel API is a built-in browser feature that lets different parts of a website —
like different tabs, windows, iframes, or even Web Workers — talk to each other directly without a server.
Think of it as creating a private chat room where any page from your app can join, send messages, and listen to others.
You create a channel by giving it a name:
const channel = new BroadcastChannel('my_channel');
Now, any other tab that creates a BroadcastChannel
with the same name joins the conversation!
🧐 Why Do We Need It?
Let's look at some real-world problems:
Syncing Authentication:
You log out in one tab. Shouldn't all tabs also log out?Managing Notifications:
Receive a message in one tab? Other tabs should show the notification too.Avoiding Duplicate Actions:
You start playing a video in one tab. Shouldn't other tabs know and stop themselves?Single WebSocket Connections:
Instead of each tab opening its own WebSocket (wasting server resources), one tab can open a WebSocket and others get data through BroadcastChannel.
Before BroadcastChannel, developers had only tricky solutions:
localStorage
change events — limited and unreliable.Server-based solutions (sockets, polling) — more complex and costly.
👉 BroadcastChannel solves this cleanly without extra servers and with very little code!
✨ Key Features of BroadcastChannel
Here’s why BroadcastChannel is exciting:
Feature | What It Means |
Native Support | No libraries, no polyfills needed |
Real-Time Messages | Instant message delivery across tabs |
Same-Origin Only | Only pages from the same site can talk |
Supports Web Workers | Great for more complex background tasks |
Simple API | Easy .postMessage() and .onmessage usage |
⚠️ Limitations You Should Know
Like everything, it’s not perfect. Here are some important things to keep in mind:
Same Origin Only:
- Tabs must have the same protocol (http/https), host (domain), and port.
No Message History:
- If a tab joins late, it doesn’t get older messages. Only live messages.
Unreliable Delivery:
- No guarantee of delivery if the browser crashes or the tab is killed.
Limited Browser Support:
Modern browsers like Chrome, Firefox, Edge, Safari ✅
Internet Explorer ❌ (Not supported)
You can check browser support here.
🛠️ Quick Example: Sending and Receiving Messages
Here’s how insanely simple it is:
// In every tab
const channel = new BroadcastChannel('app_channel');
// Listen for incoming messages
channel.onmessage = (event) => {
console.log('Received message:', event.data);
};
// Send a message
channel.postMessage('Hello, other tabs!');
✅ That’s it — real-time communication across all open tabs!
You can send any serializable object — strings, numbers, arrays, plain objects, etc.
🌎 Real-World Use Cases
Let’s make this more practical. Here are real scenarios where BroadcastChannel shines:
User Authentication Sync
- When a user logs out in one tab, all tabs auto-logout.
State Synchronization
- Like playing/pausing videos across tabs.
Single WebSocket Connection Management
- One tab connects to server, others just listen.
Notifications
- Updates (new messages, offers) pushed to all tabs instantly.
Multi-Tab Games or Collaboration
- Simple real-time updates in browser games or collaborative editing.
Tab Control
- Mark one tab as "primary" and others as "secondary".
🧠 Summary
The BroadcastChannel API is like giving your website's tabs superpowers to talk to each other.
It’s:
Simple
Native
No server needed
Extremely useful for real-world apps
🚀 What's Next?
Now that you understand the why and what,
in Part 2, we’ll explore how postMessage
via BroadcastChannel works,
how to structure messages safely,
and common pitfalls you must avoid.
👉 Read Part 2: How to Safely Send Messages Between Browser Tabs Using BroadcastChannel
Stay tuned!
Subscribe to my newsletter
Read articles from Sridhar Murali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
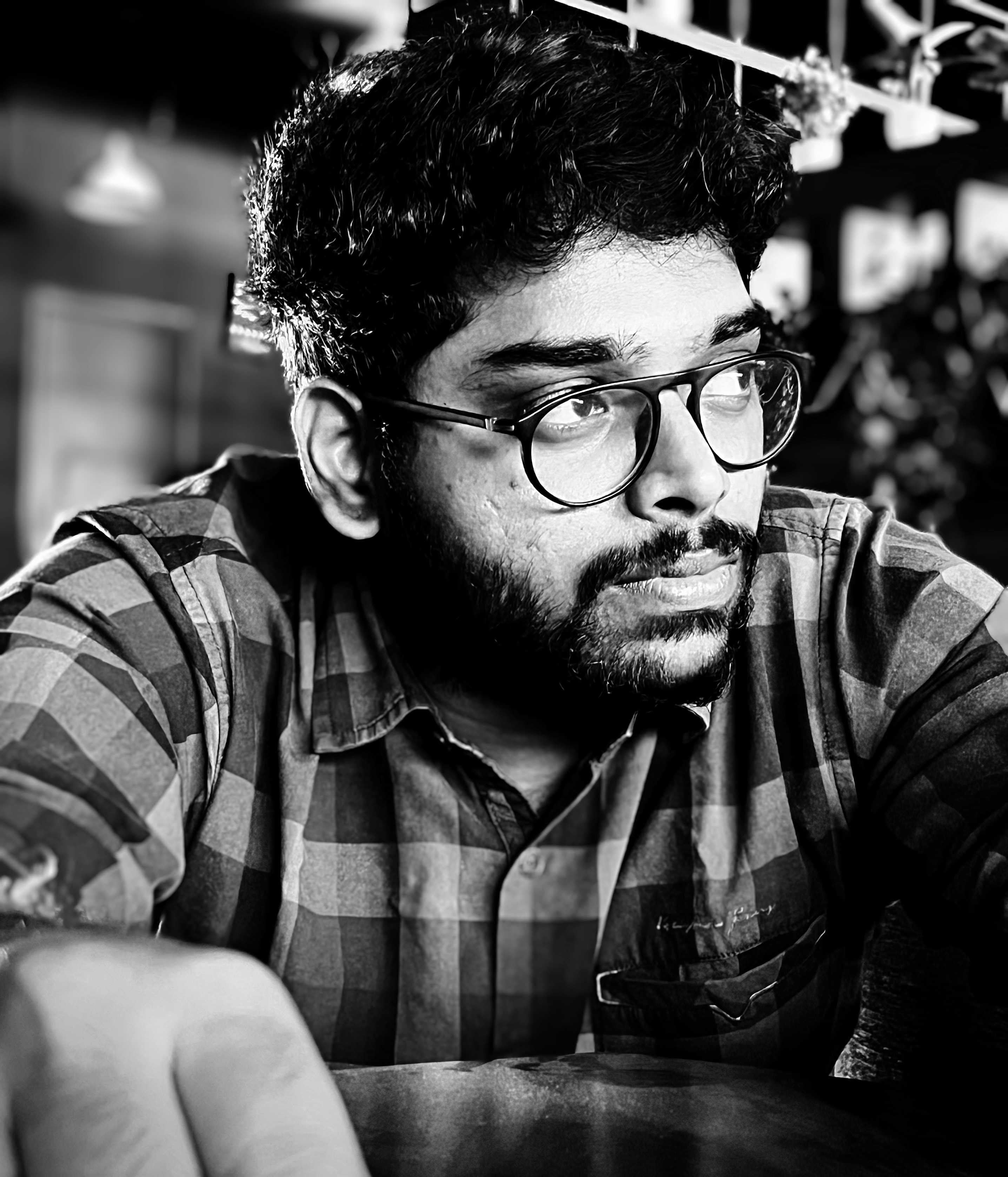
Sridhar Murali
Sridhar Murali
Passionate Frontend Engineer with over 4.5 years of experience in frontend development and a strong previous experience in Quality Assurance of 4 years (2016-2020). Skilled in building responsive, high-quality applications using JavaScript frameworks like Ember.js and React.js, combining meticulous attention to detail from a QA background with a commitment to delivering seamless user experiences. Recognized for writing efficient, accessible code, strong debugging skills