š Getting Started with GraphQL with Spring Boot: A Complete Beginnerās Guide š
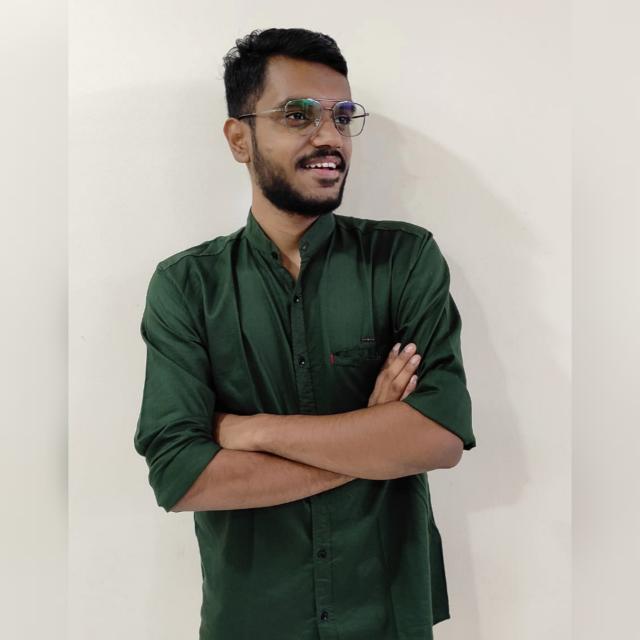

Hi everyone!
In this blog post, weāll explore GraphQL with Spring Boot. Iāll walk you through GraphQL, how it differs from REST, its key features, and how to integrate it with Spring Boot.
By the end, youāll understand how GraphQL works and how to implement it effectively in your projects.
"In the next blog, weāll take it a step further and build a Spring Boot project using GraphQL, step by step."
Letās get started!
š What is an API?
An API, or Application Programming Interface, is a way for two software systems to talk to each other. Think of it as a bridge between the frontend, like a website, and the backend, which handles the data and logic. Instead of directly accessing the database, the frontend sends a request to the API. The API then gets the data and returns it in a structured format. For example, when we use the Google Maps API, we get map data or directions without needing to know how Googleās systems work. APIs are key in modern apps where different services need to talk clearly and securely.
š What is GraphQL?
GraphQL is a query language for APIs, developed by Facebook, that makes data fetching more efficient and flexible than traditional REST APIs.
Unlike REST, which often needs multiple endpoints to get different types of data, GraphQL uses a single endpoint. Clients can request exactly the data they need, avoiding problems like over-fetching (too much data) and under-fetching (too little data).
It uses strong typing, so the structure of the data is clearly defined, and there's no need for versioning. This makes it easier for the client and server to work together smoothly.
In Spring Boot, working with GraphQL is simple: you define a schema, create resolvers, and expose a /graphql
endpoint.
Itās a clean, modern way to build APIs compared to REST.
REST API vs GraphQL
Featureā | RESTā | GraphQLā |
Endpointsā | Multipleā | Singleā |
Data Fetchingā | Fixed, sometimes redundantā | Precise and client-definedā |
Versioningā | Via versioned URLsā | Handled via schema changesā |
Flexibilityā | Limitedā | Very flexibleā |
When comparing REST and GraphQL, one big difference is how they handle endpoints. REST needs many endpointsālike /users
, /orders
, and /products
āto get different types of data. If you need related data, you have to make multiple requests. But GraphQL uses just one endpoint, and you can ask for exactly the data you want in a single request. This makes it faster and easier.
In REST, the data you get back is fixed. Sometimes you get too much data (over-fetching) or not enough (under-fetching), so you need extra calls. GraphQL lets the client choose exactly what data it needs, avoiding both problems. This saves time and improves performance.
When it comes to data fetching, REST APIs often return fixed responses, which can cause problems. Sometimes, you get more data than you need (over-fetching), or you might not get all the data you want, which means you will need to make additional requests (under-fetching). With GraphQL, the client has full control over what data it gets. You can ask for exactly what you needāno extra data, no missing data. This makes the response faster and more efficient because youāre only sending whatās necessary.
Another important difference is versioning. In REST APIs, when you want to make changes, you often have to create new versions of the API.
This can lead to a lot of different versions floating around and can make things harder to maintain. GraphQL doesnāt need versions. Instead, you just update the schema on the server. This means you can add new fields or changes without affecting existing clients, and the client can always request the most up-to-date data without worrying about version numbers.
Flexibility is one of the best things about GraphQL. In REST, the server decides what data to send, so the client might get too much or too little. But with GraphQL, the client chooses exactly what data it needs. This reduces extra data, improves speed, and makes the API work better for the client.
In short: REST uses multiple endpoints, fixed data, and versioning. GraphQL uses one endpoint, gives exact data, and is more flexibleāmaking it a better choice for modern apps.
Architecture of GraphQL šļø
In this architecture, everything starts with the client, whether it's a mobile app or a web application. The client sends a GraphQL request, which could be a query to fetch data, a mutation to modify data, or even a subscription for real-time updates.
When a request reaches the Spring Boot backend, the GraphQL Java engine steps in. It works like the brain of the systemāreading the request, checking if it matches the schema, and figuring out what data is needed. Then, it passes the job tothe resolver functions, which get the actual data from the database.
Once the data is collected, the engine assembles everything into a clean JSON response, perfectly matching the shape of the original query. This response then travels back to the client, completing the cycle. The beauty of this setup is its flexibilityāclients get exactly what they ask for, nothing more, nothing less, all through a single intelligent endpoint.
š Key Features of GraphQL š”
GraphQL offers powerful improvements over traditional REST APIs, boosting flexibility, efficiency, and performance.
Precise Data Fetching: Clients get exactly the data they request, avoiding over-fetching or under-fetching and improving speed and efficiency.
Single Endpoint: Instead of multiple REST endpoints, GraphQL uses one endpoint for all operations like fetching, updating, and subscribing to data.
Strong Typing: GraphQL enforces a strict schema, helping catch errors early, improving documentation, and ensuring smooth collaboration between frontend and backend teams.
Real-Time Updates: With subscriptions, GraphQL can push live updates to clients over WebSockets, perfect for apps like chats, notifications, and dashboards.
GraphQLās precision, simplicity, strong typing, and real-time capabilities make it an ideal choice for modern scalable apps.
ā” Why Should We Use GraphQL with Spring Boot?
Easy Integration: Add spring-boot-starter-graphql and you're ready to go.
Quick Setup: Built-in server and auto-configuration support.
Developer Tools: Use GraphiQL for testing and visualization. Strong Typing: Schema-driven APIs reduce bugs and improve clarity
š§ Core Concepts of GraphQL
Schema:
At the center of GraphQL is the schema. You can think of it as a blueprint or map for your API. It shows how the data is structured and how the client and server will talk to each other. The schema defines what types of data are availableālike text, numbers, or objectsāand what kind of queries clients are allowed to make. Itās like a contract that keeps everything clear and organized.
Schema {
query : Query
mutation : Mutation
}
Type:
At the core of GraphQL is the concept of types. GraphQL is a strongly typed language, which means every field and object in the schema is defined with a specific type. Types ensure that the data is predictable, consistent, and well-structured, which helps developers avoid errors and makes the API easier to work with.
type User {
name : String!
email : String!
}
Field:
In GraphQL, a field is a specific piece of data that is part of a type. Every type in a GraphQL schema can have multiple fields, each representing a different aspect of the data. For example, in the User type, you might have fields like name, email, and profilePicture. These fields represent pieces of information that a client might want to access when querying the API.
Each field in a type has a name and a type, and can also have additional metadata, like whether itās required or whether it can have a default value. Fields are critical because they define the granularity of the data a client can request. Instead of receiving a whole object with unnecessary data, the client can specify exactly which fields it needs, making data fetching more efficient.
Query:
A query is the most common operation in GraphQL and is used to read data from the API. Think of a query as a way to ask the server for information, like requesting a specific set of data from a database. When a client sends a query, they specify which fields of which types they want to retrieve. The server then returns the requested data in a structured format, usually as a JSON object.
type Query {
findAllUser : [User]
findUserById(id:ID):User
}
Mutation:
While queries are used for fetching data, mutations are used for making changes to dataāwhether itās creating new records, updating existing records, or deleting records. Mutations are like the "write" operations in a database; they allow clients to modify data. A mutation might be used to create a new user, update a post, or delete a comment. Mutations can accept parameters (such as the data to be inserted or updated) and return a result, typically the updated object or an acknowledgment of the change.
type Mutation {
createUser(user:User):Void
updateUser(user:User):User
}
š Integration with Spring Boot š»
Dependencies and Tools
ā¢spring-boot-starter-graphql
ā¢Postman for sending GraphQL queries
Project setup
ā¢Use Spring Initializr to generate the project
ā¢Add GraphQL starter and web dependency
ā¢Typical folders: controller, service, model, and resources/graphql/
How It Works Together
Client ā Sends GraphQL query ā /graphql endpoint ā Resolver ā Service ā Data ā Back to Client
Now that we've seen how GraphQL works, let's explore a Spring Boot project using GraphQL.
šÆConclusion:
To conclude, combining GraphQL with Spring Boot offers a modern and efficient way to build APIs that are both powerful and flexible. Unlike traditional REST, GraphQL enables clients to fetch exactly the data they need through a single endpoint, improving performance and reducing unnecessary data transfer. This approach leads to faster, more scalable, and more maintainable applications, making it an excellent choice for modern development.
What's Next: š
In the next blog, weāll explore how to practically integrate GraphQL with Spring Boot by building a real-world project step by step. Stay tuned!
About Me:
LinkedIn | GitHub
Subscribe to my newsletter
Read articles from Sumeet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
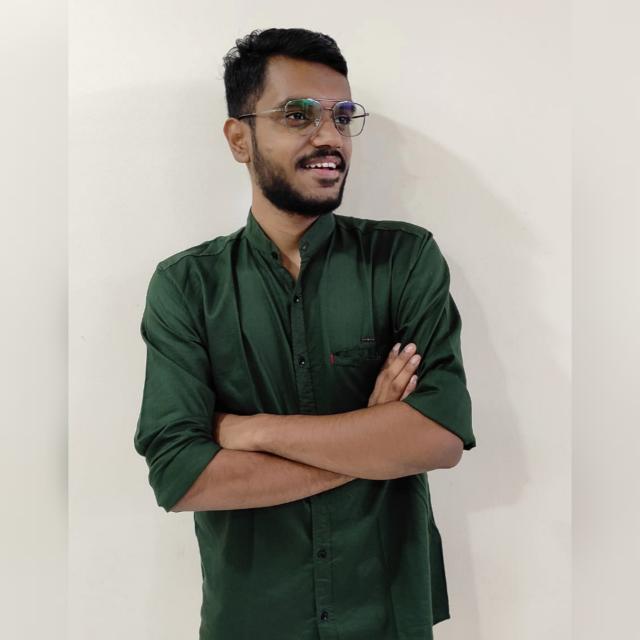