My First Attempt at Vibe Coding
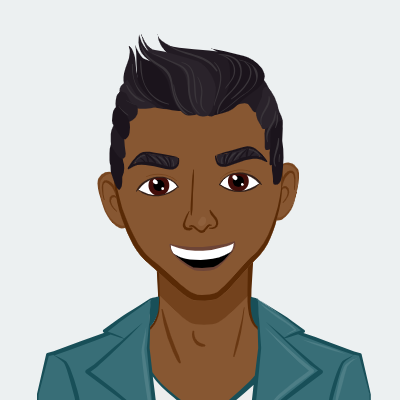

Introduction
If you’ve been on LinkedIn or Reddit there has been a huge push for vibe coding. It talks about people building entire applications without any programming expertise, solving problems quickly, rapid iteration, and so on and so forth. It sounds awesome, I am not a professional coder by any means, but I work in the tech industry and I have a lot of ideas. So it sounded perfect.
What I wanted to Build
What I wanted to build was simple, I just wanted something that would pull all urls that where loaded on a page, whether it was initial load or by js in the background. Now for this to happen I needed to run in the browser but outside the webpage. The best option is something Google hates, Extensions. I also have zero experience developing extensions, but I do know enough JS to be dangerous.
This was the opportunity I had been waiting for. Now I’m not going to pay for anything, so no integrated IDE environments, meaning no cursor or copilot. I read through all the AI rankings, (I didn’t really) I just remembered that everyone was hyping Claude, so thats what I tried with.
The first prompt I used was:
how do I detect a url call on a page
Its a pretty poor prompt, but interestingly Claude actually understood me intent (Yes using “understood” is wrong when speaking about LLMs but it highlights how deterministic language is). The output had 4 different options:
Using the Fetch API or XMLHttpRequest Event Listeners
Using the Performance API
Using Browser DevTools Network Monitor
Using Service Workers
All of these are good ideas, and I would not have thought of options 2 and 3. However none of these work for me because I can’t load JS on the page as I want to monitor pages I don’t own. So I reframed my question.
how about using an extension
I will skip all of the ai fluff agreeing with the suggestion. It again gave me three options.
Use an existing module - for my purposes useless.
Extension using a service worker
Extension using a content injection model.
So whats the difference between a service worker extension and a content injection extension? I didn’t know at the time. To be honest I am not clear on it now either. It is something that I need to look into when I further develop this extension that I am building. So going with the theme of this I went with the vibe and I chose option 2. To see where it would take me. This is the code it gave me.
{
"name": "URL Call Detector",
"version": "1.0",
"manifest_version": 3,
"permissions": ["webRequest", "webRequestBlocking", "<all_urls>"],
"background": {
"service_worker": "background.js"
}
}
// background.js
chrome.webRequest.onBeforeRequest.addListener(
function(details) {
console.log("URL call detected:", details.url);
// You can also log method, type, timestamp, etc.
return {cancel: false}; // Don't block the request
},
{urls: ["<all_urls>"]},
["blocking"]
);
This code is remarkably close to what I wanted to achieve, in fact it looked perfect. I pulled it altogether into chrome and tried to run it but it failed with errors. Now I have some background context on why it was failing. Google had recently (read June 2024) deprecated Manifest v2. Now I wouldn’t really know much about this except for the fact that this broke every adblocker out there. The replacement module Manifest v3 is a poor replacement as it doesn’t allow the same level of access to block ads.
Why did I suspect that this was the issue, well, the manifest version is set to 3, and we have the “blocking tag.” I ended up going to the docs and yep that was the issue. In a blue box at the top of the page with a star
So I went back to Claude because this is an experiment in vibe coding.
request blocking does not work with manifest3
It decided to start from scratch and decided to give me this code:
// background.js
// For monitoring requests without blocking them
chrome.declarativeNetRequest.onRuleMatchedDebug.addListener(
(info) => {
console.log("Matched URL:", info.request.url);
// Store the request info if needed
chrome.storage.local.get(['requests'], (result) => {
const requests = result.requests || [];
requests.push({
url: info.request.url,
method: info.request.method,
timestamp: Date.now()
});
chrome.storage.local.set({requests: requests});
});
}
);
// Set up rules to match all requests
chrome.declarativeNetRequest.updateSessionRules({
addRules: [{
id: 1,
priority: 1,
action: { type: "allow" },
condition: {
urlFilter: "*",
resourceTypes: ["main_frame", "sub_frame", "stylesheet", "script", "image", "font", "object", "xmlhttprequest", "ping", "other"]
}
}]
});
This did not work either, I went back to Claude with the error that I found.
Trying to use option 1 leads to the error
Uncaught TypeError: Cannot read properties of undefined (reading 'addListener')
In response Clade decided to start again. It generated new code that did not work either. To cut a long story short I kept going back with errors and it kept coming back with solutions that did not work. After a an hour or two of this bullshit (Note my frustration at this point). What was worse was that it was generating even more complex code, rather than continuing to solve my problem. It generated a popup.htm file, and even more things.
In the end out of sheer frustration I deleted everything and went back to the original prompt response and went to the original docs. This is what I ended up with.
chrome.webRequest.onBeforeRequest.addListener(
function(details) {
console.log(details.urls);
},
{urls: ["<all_urls>"]},
);
It worked!
So what where the fundamental errors? There were actually 2, the first was Claude generating more and more complex code without understanding what I was trying to do. Also I was feeding it bad info because of the second issue. When you run a background service the errors or the log output does not appear in the default console. Instead there is a seperate console that you have to open a seperate console. This means that there was a really good chance (read definite) that I was feeding Claude the wrong error messages as well.
Conclusion
You have to remember there are claims that people are deploying entire applications after building it with vibe coding with no programming experience. My experience demonstrates that these claims are highly suspect. A person with no programming experience is not going to be able to utilise AI in a productive manner. We need to have a basic understanding and also need to read docs to be able to utilise this correctly. What I did find is that if I need to generate basic code quickly, this is super useful. I generated a website and it looked great. After I understood how to test properly the generated code actually makes sense.
Subscribe to my newsletter
Read articles from Vishnu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
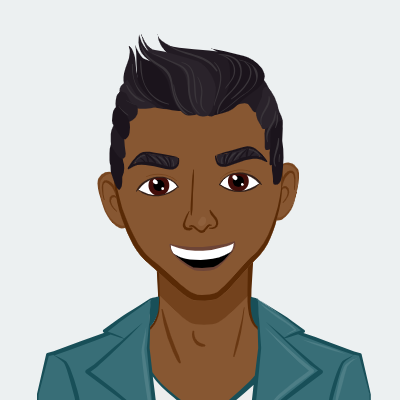
Vishnu
Vishnu
A science and tech enthusiast with opinions on a variety of topics.