Techniques for Effective Background Task Management
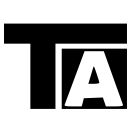
6 min read
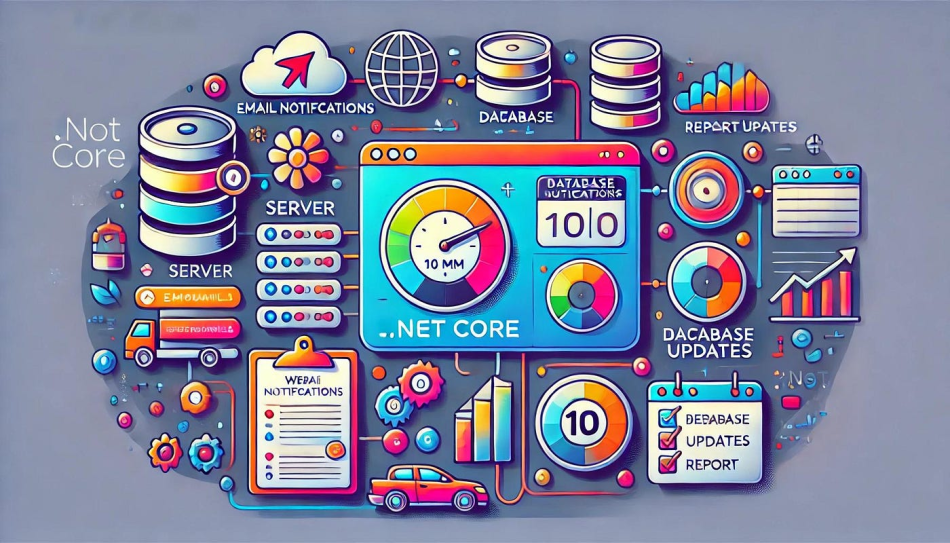
1. Understanding Background Task Management in Spring Boot
1.1 The Purpose of Scheduling in Modern Applications
In microservices and cloud-native environments, many tasks occur periodically or in response to certain triggers. Common tasks include:
- Data Synchronization: Keeping data between services or databases in sync.
- Batch Processing: Running batch jobs, such as end-of-day financial calculations.
- Maintenance Tasks: Cleaning up temporary files, optimizing database tables, or recalculating aggregated metrics.
Without scheduling, developers would need to manually code the timing and execution of these tasks, which can lead to inefficiencies and cluttered codebases. Spring Boot’s built-in scheduling features help keep your code organized and task management simple.
1.2 Key Components of Spring Boot Scheduling
Spring Boot’s scheduling is built upon the @EnableScheduling annotation and the @Scheduled annotation. The first step to harness the power of Spring Boot’s scheduling features is enabling scheduling in your application.
2. Setting Up and Configuring Scheduling
2.1 Enabling Scheduling in Your Application
The @EnableScheduling annotation should be added to the main application class or a configuration class to activate the scheduling framework.
Example Code:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class SchedulingApplication {
public static void main(String[] args) {
SpringApplication.run(SchedulingApplication.class, args);
}
}
With this simple step, Spring Boot now knows it should look for scheduled tasks when initializing the application context.
2.2 Scheduling Tasks with @Scheduled
The @Scheduled annotation provides several ways to define task timing:
- fixedRate: Runs a task at a fixed interval.
- fixedDelay: Runs a task after a specified delay.
- cron: Uses cron expressions for complex scheduling.
Example Code with Explanations:
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class ScheduledTasks {
// Task runs every 5 seconds
@Scheduled(fixedRate = 5000)
public void fixedRateTask() {
System.out.println("Fixed rate task executed");
}
// Task runs 10 seconds after the previous one finishes
@Scheduled(fixedDelay = 10000)
public void fixedDelayTask() {
System.out.println("Fixed delay task executed");
}
// Task runs at the beginning of every hour
@Scheduled(cron = "0 0 * ?")
public void cronTask() {
System.out.println("Cron task executed at the top of every hour");
}
}
Each of these options allows flexibility depending on your requirements. For example, if you need a task to execute at a specific time each day, cron expressions are ideal. The fixedRate approach works well for repetitive tasks that need consistent intervals, such as polling an external service.
2.3 Understanding and Creating Cron Expressions
What is a Cron Expression? A cron expression is a string of parameters that define when a job should run, down to the minute or even the second. The format of a cron expression in Spring Boot is "seconds minutes hours day-of-month month day-of-week year".
- "0 0 ?" – runs at the top of every hour.
- "0 15 10 * ?" – runs every day at 10:15 AM.
By using cron expressions, you can create highly customized schedules, ideal for tasks like daily reports, weekly maintenance, or monthly cleanups.
3. Best Practices for Effective Scheduling
3.1 Avoiding Task Overlaps
Task overlaps can occur when a scheduled task hasn’t finished executing before the next one starts. This can lead to resource contention and even application crashes. To handle this:
- Option 1: Use @Async with @Scheduled to enable asynchronous task execution.
- Option 2: Consider using a distributed lock (e.g., Redisson) if running in a clustered environment.
Example with Asynchronous Scheduling:
import org.springframework.scheduling.annotation.Async;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class AsyncTasks {
@Async
@Scheduled(fixedRate = 10000)
public void asyncScheduledTask() {
System.out.println("Asynchronous task executed with no overlaps");
}
}
This allows the task to run in a separate thread, avoiding any overlap with the main thread, and allowing the main thread to process other requests.
3.2 Managing Thread Pools for Efficient Task Execution
By default, Spring Boot uses a single-threaded task scheduler. In high-traffic or concurrent task environments, you can configure a thread pool to allow multiple tasks to run concurrently, avoiding bottlenecks.
Example Configuring a Custom ThreadPoolTaskScheduler:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.scheduling.concurrent.ThreadPoolTaskScheduler;
@Configuration
public class SchedulerConfig {
@Bean
public ThreadPoolTaskScheduler taskScheduler() {
ThreadPoolTaskScheduler scheduler = new ThreadPoolTaskScheduler();
scheduler.setPoolSize(10);
scheduler.setThreadNamePrefix("MyScheduler-");
return scheduler;
}
}
By setting a pool size, you ensure that a set number of threads are available for scheduling tasks, reducing the risk of blocking.
3.3 Handling Errors and Exceptions Gracefully
Scheduled tasks can fail due to exceptions, which can stop the scheduler entirely. To prevent this, wrap your scheduled tasks in try-catch blocks and handle exceptions properly.
Example with Exception Handling:
@Component
public class ErrorHandledTasks {
@Scheduled(fixedRate = 15000)
public void safeTask() {
try {
System.out.println("Executing safely...");
// Simulate task code that may throw an exception
} catch (Exception e) {
System.err.println("Exception caught during scheduled task: " + e.getMessage());
}
}
}
This ensures that your application continues running even if one of the tasks encounters an issue.
4. Common Issues and Troubleshooting
Start-up Dependencies
Tasks may start running before all beans are initialized. Consider using @DependsOn to specify dependencies, especially if the task relies on a service or another bean that might not be available at start-up.
Performance Implications of Long-Running Tasks
When a task takes longer than expected, it can delay subsequent tasks. For such tasks, consider breaking them into smaller parts, or moving them to a dedicated background worker service using Spring Boot Batch or external task queues like Kafka.
Monitoring and Logging Scheduled Tasks
Logging is crucial for monitoring scheduled tasks. Consider using an external logging system or Spring Boot’s Actuator to monitor task health and execution times.
5. Conclusion
Effective background task management in Spring Boot helps ensure your application remains responsive and reliable. By implementing the best practices above, you’ll be well on your way to creating a robust and maintainable scheduling system. If you have questions or specific challenges you’d like to discuss, drop a comment below!
Read more at : Techniques for Effective Background Task Management
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
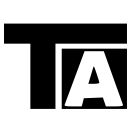
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.