What are React Bootstrap Components
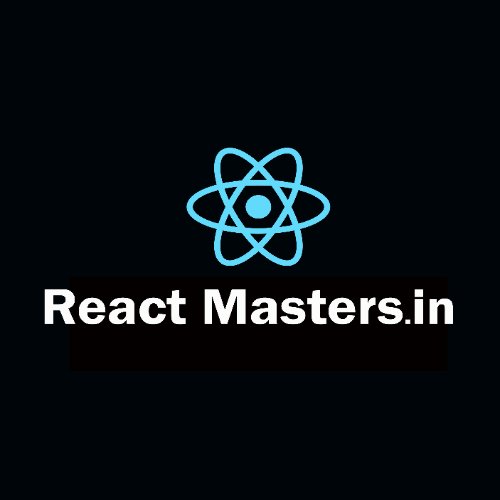
In modern web development, combining powerful frameworks can lead to highly responsive, user-friendly, and visually appealing applications.
React Bootstrap is one such combination that brings together the best of both worlds React JS and Bootstrap.
This article will explore what React Bootstrap is, its components, benefits, use cases, and more.
📘 What is React Bootstrap?
React Bootstrap is a library of reusable React components built using the Bootstrap framework. Instead of using Bootstrap’s JavaScript plugins (which rely on jQuery), React Bootstrap re-implements those plugins as native React components.
This means you get the familiar styling and responsive grid system of Bootstrap, while still taking full advantage of React’s component-based architecture, virtual DOM, and state management.
🔍 Example:
Instead of using HTML like this:
htmlCopyEdit<button class="btn btn-primary">Click Me</button>
With React Bootstrap, you use:
jsxCopyEditimport Button from 'react-bootstrap/Button';
<Button variant="primary">Click Me</Button>
This is cleaner, reusable, and much easier to manage in large-scale applications.
✅ Benefits of Using React Bootstrap
Using React Bootstrap offers a long list of advantages for developers and project teams. Here are the key benefits:
1. 🔄 Seamless React Integration
React Bootstrap is built for React—no jQuery or DOM manipulation. This means better compatibility, cleaner code, and faster performance.
2. ⚙️ Pre-Styled Components
Every component comes pre-styled using Bootstrap’s classes. You don’t need to manually add CSS for buttons, forms, modals, or cards.
3. 💡 Easy to Use and Customize
Each component can be modified using props. Want a small button? Just pass the size="sm"
prop. Need a different color? Use variant="success"
.
4. 🧩 Modular and Reusable
You can build UI faster by reusing components across your app. React encourages breaking down UIs into small, manageable parts.
5. 📱 Mobile-Responsive by Default
Built on Bootstrap’s grid system, components automatically adjust to screen sizes—no extra media queries needed.
6. 🌍 Strong Community and Documentation
React Bootstrap has extensive documentation and a large user base. You’ll always find support, examples, and troubleshooting help.
🧱 What are React Bootstrap Components?
React Bootstrap offers a full suite of UI components that match the functionality of standard Bootstrap but are written entirely in React. These include:
Buttons
Forms
Cards
Modals
Alerts
Navbars
Tabs
Accordions
Spinners
Carousels
Dropdowns
Tables
Tooltips
Popovers
Toasts
Pagination
Grids and more
Each of these components can be easily imported and used in your React application with minimal setup.
📝 React Bootstrap Forms – A Closer Look
Forms are one of the most used elements in any web application. React Bootstrap makes it easy to build accessible and attractive forms.
🚀 Features of React Bootstrap Forms:
Clean structure using
<Form>
and<
Form.Group
>
componentsForm controls for input, email, password, text area, checkbox, radio, select dropdown
Built-in validation support
Ability to work smoothly with libraries like React Hook Form or Formik
📦 Example:
jsxCopyEdit<Form>
<Form.Group controlId="formName">
<Form.Label>Your Name</Form.Label>
<Form.Control type="text" placeholder="Enter your name" />
</Form.Group>
<Form.Group controlId="formEmail">
<Form.Label>Email address</Form.Label>
<Form.Control type="email" placeholder="Enter email" />
</Form.Group>
<Button variant="primary" type="submit">Submit</Button>
</Form>
This creates a clean, responsive form without writing a single line of CSS!
🧭 Key React Bootstrap Components – Expanded
Let’s explore some of the most widely used React Bootstrap components in more detail:
🟢 1. Button
A simple yet powerful component used for user actions.
jsxCopyEdit<Button variant="warning" size="lg">Download</Button>
🟣 2. Card
Used to display content in a structured and elegant way.
jsxCopyEdit<Card style={{ width: '18rem' }}>
<Card.Img variant="top" src="image.jpg" />
<Card.Body>
<Card.Title>Card Title</Card.Title>
<Card.Text>This is a card with some text.</Card.Text>
<Button variant="primary">Go Somewhere</Button>
</Card.Body>
</Card>
🔵 3. Modal
Perfect for alerts, confirmation messages, or forms in a popup.
jsxCopyEdit<Modal show={true} onHide={handleClose}>
<Modal.Header closeButton>
<Modal.Title>Welcome</Modal.Title>
</Modal.Header>
<Modal.Body>Thanks for visiting!</Modal.Body>
<Modal.Footer>
<Button variant="secondary" onClick={handleClose}>Close</Button>
</Modal.Footer>
</Modal>
🟠 4. Navbar
Use for headers, menus, and navigation links.
jsxCopyEdit<Navbar bg="dark" variant="dark">
<Navbar.Brand href="#home">MyApp</Navbar.Brand>
<Nav className="me-auto">
<Nav.Link href="#features">Features</Nav.Link>
<Nav.Link href="#pricing">Pricing</Nav.Link>
</Nav>
</Navbar>
🔁 React Bootstrap vs Traditional Bootstrap
Feature | Bootstrap (with HTML/JS) | React Bootstrap |
Integration | HTML + jQuery | Native React components |
Reusability | Limited | Highly reusable |
DOM Control | Manual via jQuery | Virtual DOM |
State Management | External JS | React state/props |
Performance | Slower for dynamic UIs | Optimized for re-rendering |
Customization | CSS classes | Props & JSX |
🔧 How to Install React Bootstrap
To start using React Bootstrap, follow these steps:
1. Install via npm:
bashCopyEditnpm install react-bootstrap bootstrap
2. Import Bootstrap CSS:
In your index.js
or App.js
:
javascriptCopyEditimport 'bootstrap/dist/css/bootstrap.min.css';
3. Import Components as Needed:
javascriptCopyEditimport Button from 'react-bootstrap/Button';
import Form from 'react-bootstrap/Form';
🧠 When Should You Use React Bootstrap?
React Bootstrap is a perfect choice when:
You’re working on a React-based project
You want a mobile-friendly, responsive UI
You don’t want to write custom CSS for everything
You want faster development time
You need a maintainable, scalable frontend structure
You’re building forms, dashboards, landing pages, or admin panels
🔚 Conclusion
In 2025, as the demand for clean, fast, and responsive web applications continues to rise, React Bootstrap stands out as a go-to solution for frontend developers.
It allows you to build attractive, functional UIs without the hassle of managing complex styles or DOM manipulation.
With its large component library, flexibility, and React-native integration, React Bootstrap offers everything you need to create modern web apps quickly, reliably, and professionally.
If you’re learning React or planning to build production-grade apps, don’t overlook the power of React Bootstrap components.
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
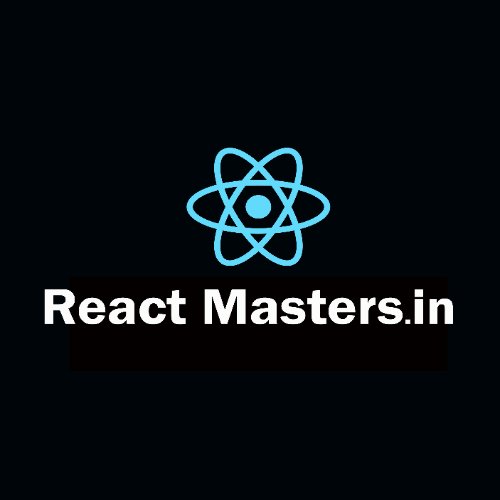
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.