🌀 Control Flow in Python: A Complete Guide for Beginners
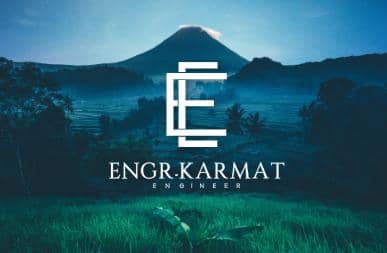

🔍 Introduction
Control flow is the backbone of any programming language, determining the order in which code executes. In Python, control flow lets you make decisions, repeat tasks, and manage complex logical sequences. Understanding it is essential whether you're building a calculator, a web app, or solving coding challenges.
In this article, you’ll learn how Python handles:
- Conditional statements
- Loops
- Loop control statements (
break
,continue
,pass
) - Nested control structures
📘 What is Control Flow?
Control flow refers to the order in which individual statements, instructions, or function calls are executed or evaluated. Python provides several structures to control this flow:
- Conditional Statements
- Loops
- Control Flow Tools
✅ 1. Conditional Statements (Decision-Making)
Python uses if
, elif
, and else
to perform decision-making:
Syntax:
if condition:
# do something
elif another_condition:
# do something else
else:
# fallback
Example:
x = 10
if x > 0:
print("Positive")
elif x == 0:
print("Zero")
else:
print("Negative")
🧠 Tip: Indentation is crucial in Python. Always indent blocks under if
, else
, etc.
🔁 2. Loops (Iteration)
Python supports two main types of loops:
➤ for
Loop
Iterates over a sequence (list, string, tuple, etc.)
for i in range(5):
print(i)
➤ while
Loop
Repeats as long as a condition is true
count = 0
while count < 5:
print(count)
count += 1
🛑 3. Loop Control Statements
These help you manage how and when a loop exits or continues.
🔹 break
Exits the loop early
for i in range(10):
if i == 5:
break
print(i)
🔹 continue
Skips the current iteration
for i in range(5):
if i == 2:
continue
print(i)
🔹 pass
Placeholder — does nothing, used to define empty blocks
for i in range(3):
pass # maybe add logic later
🔄 4. Nested Control Flow
You can nest if
statements and loops within each other.
for i in range(3):
if i % 2 == 0:
print(f"{i} is even")
else:
print(f"{i} is odd")
💡 Real-world Example: FizzBuzz Problem
Problem:
Print numbers from 1 to 15. For multiples of 3, print "Fizz"; for 5, print "Buzz"; for both, print "FizzBuzz".
Solution:
for i in range(1, 16):
if i % 3 == 0 and i % 5 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
⏱️ Time & Space Complexity
- Control structures themselves don't have a "complexity" per se — it depends on the number of operations inside.
- Loops:
O(n)
if loopingn
times. - Nested loops:
O(n²)
if both aren
.
⚠️ Common Mistakes to Avoid
- Misusing indentation (will raise
IndentationError
) - Forgetting to update conditions in
while
loops (can lead to infinite loops) - Using
=
instead of==
in conditions
🧩 Conclusion
Control flow is essential in writing meaningful, logical programs. With a solid understanding of conditionals, loops, and flow tools like break
and continue
, you’ll be able to tackle a wide range of coding problems efficiently.
🧠 Bonus Challenges to Practice
- Write a program to check if a number is prime.
- Create a pattern using nested loops.
- Simulate a login system using
while
with max 3 attempts.
Subscribe to my newsletter
Read articles from Abdulkareem Abdulmateen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
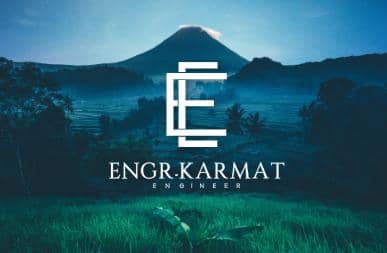