PostgreSQL CASE: Conditional Logic Made Easy in SQL
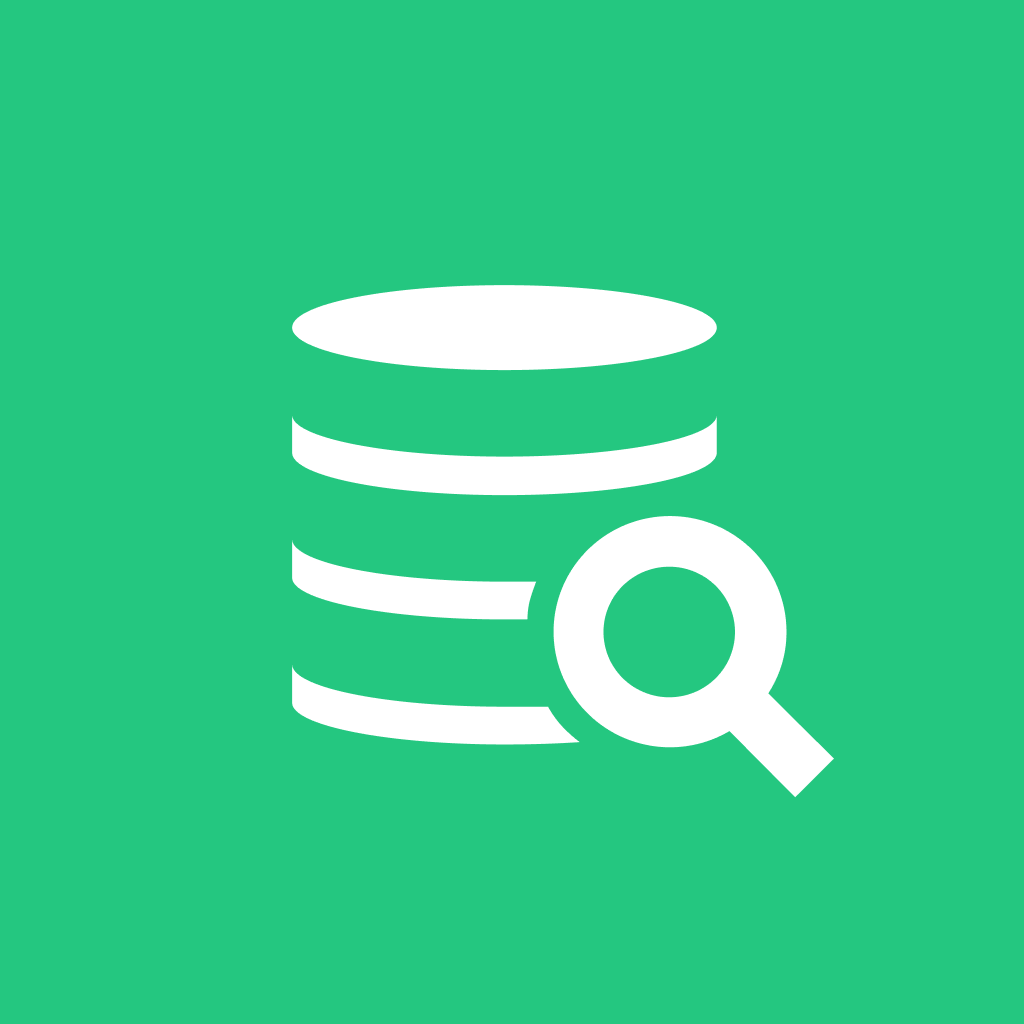

The CASE statement in PostgreSQL allows you to implement conditional logic within SQL queries, returning different results based on defined conditions. It’s a critical feature for developers who need to manipulate data directly in their queries without resorting to complex subqueries or additional logic in their applications.
Key Examples
Simple CASE:
SELECT product_name,
CASE product_category
WHEN 'Electronics' THEN 'High-Tech'
WHEN 'Clothing' THEN 'Fashion'
ELSE 'Other'
END AS category_group
FROM products;
This example checks the product_category
and returns a corresponding group.
Searched CASE:
SELECT order_id, order_quantity,
CASE
WHEN order_quantity > 100 THEN 'Large'
WHEN order_quantity > 50 THEN 'Medium'
ELSE 'Small'
END AS order_size
FROM orders;
The searched CASE provides greater flexibility by evaluating multiple conditions.
Use Cases
Data Categorization
Categorizing customers based on their spending:
SELECT customer_name,
CASE
WHEN total_purchases > 1000 THEN 'VIP'
WHEN total_purchases > 500 THEN 'Preferred'
ELSE 'Regular'
END AS customer_category
FROM customers;
NULL Handling
Replace NULL values with meaningful data:
SELECT product_name, price,
CASE
WHEN price IS NULL THEN '0'
ELSE price
END AS formatted_price
FROM products;
Custom Sorting
Sort employees by specific job titles using CASE:
SELECT employee_name, job_title
FROM employees
ORDER BY
CASE job_title
WHEN 'Manager' THEN 1
WHEN 'Supervisor' THEN 2
ELSE 3
END;
Best Practices
Prioritize Conditions: Ensure that your conditions are ordered logically, with more specific conditions at the top to avoid unintended results.
Comment Complex Logic: Add comments when your CASE logic becomes complex. This improves readability for other developers.
Thorough Testing: Test your queries in different scenarios to make sure the CASE statement functions as expected.
FAQ
What’s the difference between a simple and searched CASE?
- A simple CASE compares a single expression to several values, while a searched CASE allows more complex, independent condition checks.
Can CASE be used with WHERE or UPDATE?
- Yes, CASE can be used in SELECT, WHERE, and UPDATE clauses to conditionally alter or filter data based on defined criteria.
Conclusion
The PostgreSQL CASE statement is a versatile and powerful feature that simplifies conditional logic in SQL queries. It’s an essential tool for efficiently handling conditions, categorizing data, and managing NULL values. To explore the full details, check out PostgreSQL CASE: A Comprehensive Guide.
Subscribe to my newsletter
Read articles from DbVisualizer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
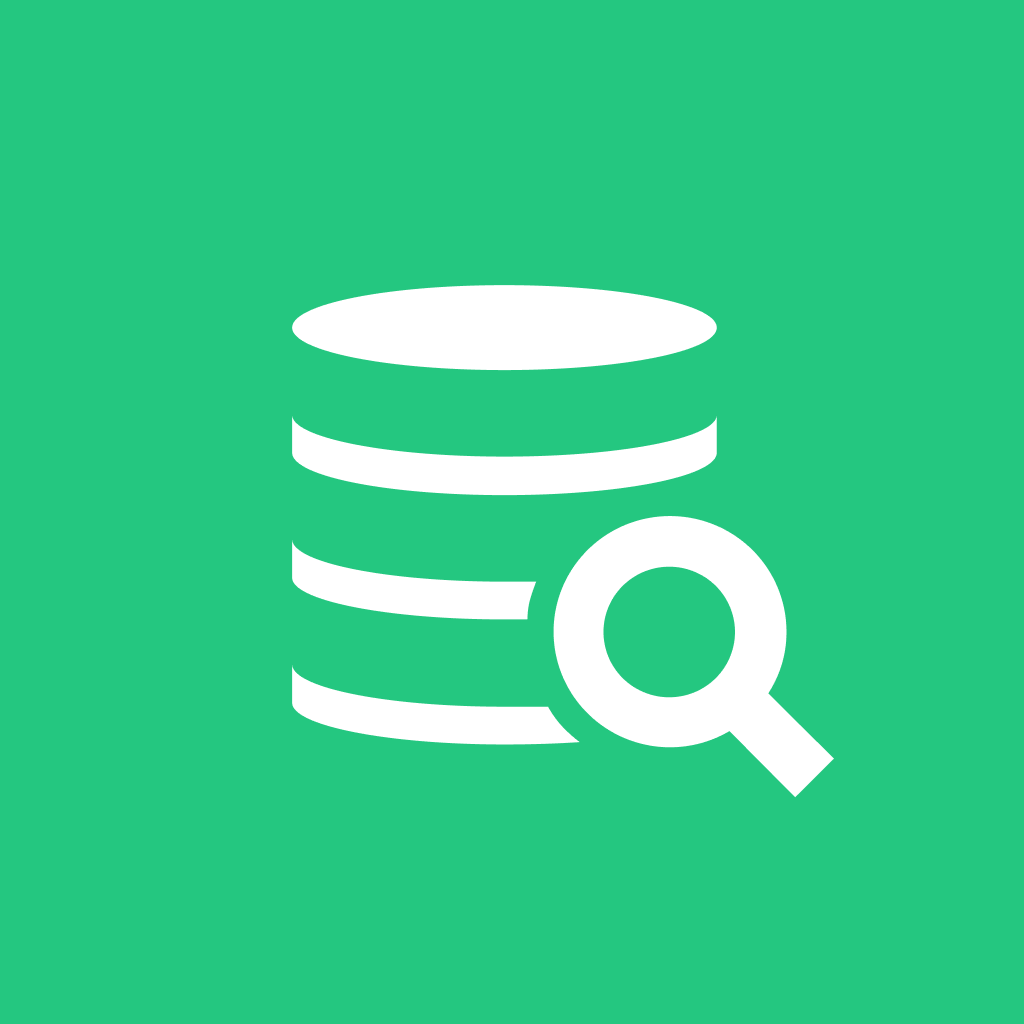
DbVisualizer
DbVisualizer
DbVisualizer is the database client with the highest user satisfaction. It is used for development, analytics, maintenance, and more, by database professionals all over the world. It connects to all popular databases and runs on Win, macOS & Linux.