Mediator Pattern in Java for Simplified Object Communication
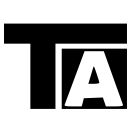
5 min read
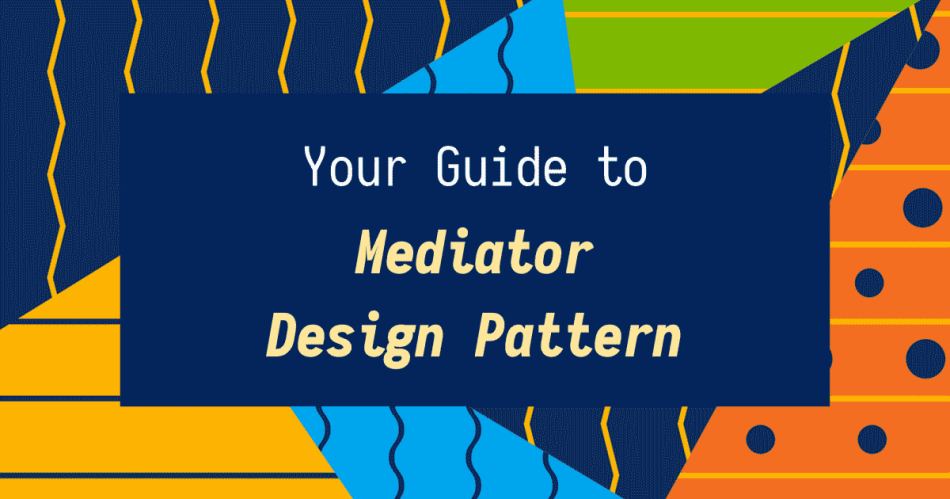
1. What is the Mediator Pattern, and Why Does It Matter?
1.1 Understanding the Core Concept
The Mediator Pattern is a behavioral design pattern that allows objects to communicate through a central mediator rather than directly with each other. This helps to reduce the dependencies among classes and promotes loose coupling. By managing the communication through a mediator, the code becomes less complex, enhancing readability and maintainability.
1.2 The Advantages of Using the Mediator Pattern
When applied correctly, the Mediator Pattern can:
- Simplify Communication Logic: With all interactions routed through a central mediator, modifying how objects communicate becomes simpler.
- Enhance Scalability: By removing direct dependencies between objects, you can easily add or remove components.
- Promote Code Reusability: Decoupled components are more reusable since they don’t rely on specific interactions with other components.
1.3 Use Cases in Java Applications
It’s especially useful in scenarios such as GUI applications, chat applications, and transaction systems where numerous objects interact.
Example: In a chat room, users send messages to a mediator (chat server) that relays messages to the appropriate users. Each user doesn’t need to know the details of the communication process, only how to send messages to the server.
2. Implementing the Mediator Pattern in Java: A Step-by-Step Guide
Let’s build a practical example of a chat room where users can communicate through a central ChatMediator. This example will clarify the roles of different components within the Mediator Pattern and showcase best practices.
2.1 Defining the Core Components
Mediator Interface: Outlines the methods for communication.
Concrete Mediator: Implements the mediator interface and handles communication among User objects.
Colleague Interface: Represents the participants (users).
Concrete Colleagues: Users who communicate through the mediator.
2.2 Building the Mediator Interface and ConcreteMediator Class
interface ChatMediator {
void sendMessage(String message, User user);
void addUser(User user);
}
class ChatMediatorImpl implements ChatMediator {
private List<User> users = new ArrayList<>();
@Override
public void addUser(User user) {
this.users.add(user);
}
@Override
public void sendMessage(String message, User sender) {
for (User user : users) {
if (user != sender) {
user.receive(message);
}
}
}
}
Explanation: Here, ChatMediatorImpl manages a list of users and defines the logic for message passing. The sendMessage method checks that the sender does not receive their own message, which is sent to other users only.
2.3 Implementing the User (Colleague) Class
abstract class User {
protected ChatMediator mediator;
protected String name;
public User(ChatMediator mediator, String name) {
this.mediator = mediator;
this.name = name;
}
public abstract void send(String message);
public abstract void receive(String message);
}
class UserImpl extends User {
public UserImpl(ChatMediator mediator, String name) {
super(mediator, name);
}
@Override
public void send(String message) {
System.out.println(this.name + " sends: " + message);
mediator.sendMessage(message, this);
}
@Override
public void receive(String message) {
System.out.println(this.name + " receives: " + message);
}
}
Explanation: In the UserImpl class, we have the send method, which sends a message to the mediator, and a receive method, which outputs the received message to the console. This setup effectively decouples users from one another; they only interact with the ChatMediator.
2.4 Testing the Mediator Pattern in Action
public class MediatorPatternExample {
public static void main(String[] args) {
ChatMediator chatMediator = new ChatMediatorImpl();
User user1 = new UserImpl(chatMediator, "Alice");
User user2 = new UserImpl(chatMediator, "Bob");
User user3 = new UserImpl(chatMediator, "Charlie");
chatMediator.addUser(user1);
chatMediator.addUser(user2);
chatMediator.addUser(user3);
user1.send("Hello, everyone!");
}
}
Explanation: This main method demonstrates how Alice sends a message that’s broadcast to Bob and Charlie through the mediator. No direct dependencies exist between users, showcasing the simplicity and flexibility introduced by the Mediator Pattern.
3. Best Practices for Using the Mediator Pattern in Java
3.1 Start Simple with a Focused Mediator
It’s best to start with a mediator that only manages the interactions it needs to. Overloading the mediator can turn it into a “god object,” which defeats the pattern’s purpose of reducing complexity.
3.2 Favor Composition over Inheritance
Using interfaces and composition makes the code more flexible and aligns with the principles of clean code design.
4. Potential Challenges and How to Address Them
Performance Overhead
In high-performance systems, using a mediator can add latency due to the extra layer of communication. This is often a trade-off for the improved maintainability and should be carefully evaluated.
Testing Complexity
While the pattern simplifies communication, testing can become challenging as you must ensure all interactions function correctly. Employing unit tests for the mediator itself can help verify correct behavior across all components.
5. Conclusion
The Mediator Pattern can transform how objects interact, leading to cleaner, more modular code. With the right approach, it can simplify your communication flows and enhance your code’s scalability. Are there any particular challenges you’ve faced with implementing design patterns? Feel free to leave a comment below, and let’s discuss!
Read more at : Mediator Pattern in Java for Simplified Object Communication
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
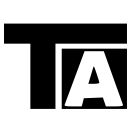
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.