Sorting Algorithm 1 (Selection Sort)
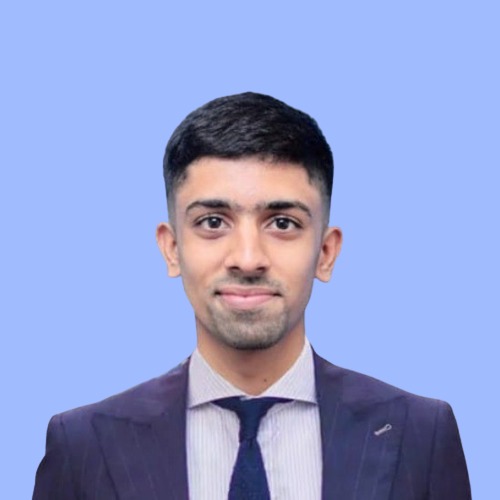

- The selection sort algorithm is a straightforward sorting technique that operates by repeatedly identifying the smallest element from the unsorted portion of a list and moving it to the beginning of the sorted section.
This in-place sorting method is memory-efficient, as it does not require additional storage beyond the input data itself, making it suitable for large datasets. However, its time complexity is O(n²), which limits its efficiency for larger inputs compared to more advanced algorithms. Notably, selection sort is a stable sorting algorithm, meaning that it maintains the relative order of equal elements, which can be crucial in certain applications. Overall, while selection sort exemplifies basic algorithm design principles, its practical use is often overshadowed by more efficient sorting methods in performance-critical scenarios.
Basic Algorithm Idea
Selection sort operates in two primary phases:
Selection Phase: Scans the unsorted portion of the array to find the minimum element
Swapping Phase: Exchanges this minimum element with the first element of the unsorted section
The algorithm progresses by maintaining two subarrays:
The sorted subarray (initially empty)
The unsorted subarray (initially full)
With each iteration, the sorted subarray grows by one element while the unsorted subarray shrinks by one.
Step-by-Step Example: Original array: [64, 25, 12, 22, 11]
Find minimum (11) in entire array, swap with first element →
[11, 25, 12, 22, 64]
Find minimum (12) in remaining
[25,12,22,64]
, swap with second element →[11,12,25,22,64]
Find minimum (22) in
[25,22,64]
, swap with third element →[11,12,22,25,64]
Remaining elements are already sorted
Pseudocode
selection_sort(A)
n = length(A)
for i from 0 to n-1
min_index = i
for j from i+1 to n
if A[j] < A[min_index]
min_index = j
swap A[i] with A[min_index]
Implementation:
Java Implementation
public class SelectionSort {
public static void sort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
}
}
#include <vector>
using namespace std;
void selectionSort(vector<int>& arr) {
int n = arr.size();
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
swap(arr[i], arr[minIndex]);
}
}
function selectionSort(arr) {
const n = arr.length;
for (let i = 0; i < n - 1; i++) {
let minIndex = i;
for (let j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
[arr[i], arr[minIndex]] = [arr[minIndex], arr[i]];
}
return arr;
}
Complexity Analysis
Time Complexity:
Best Case: O(n²) (even if array is already sorted)
Average Case: O(n²)
Worst Case: O(n²)
The algorithm always performs (n² - n)/2 comparisons and O(n) swaps
Space Complexity: O(1) (in-place sorting)
Adaptive?: No (doesn't adapt to partially sorted data)
Stable?: No (swapping can change order of equal elements)
Stability Analysis
Selection sort is not stable because it swaps non-adjacent elements. Consider this example:
Original: [3a, 2, 3b]
(where 3a and 3b are equal elements) After sorting: [2, 3b, 3a]
(original order of equal elements is lost)
When to Use Selection Sort
Small datasets: When n is small (typically <10)
Memory constraints: When you need minimal memory overhead
Educational purposes: To demonstrate fundamental sorting concepts
Embedded systems: With limited memory but small data sizes
When to Avoid Selection Sort
Large datasets: Performance degrades quadratically with dataset size
Performance-critical applications: O(n²) complexity makes it unsuitable
Datasets with many duplicates: Instability could alter data relationships
Adaptive sorting needs: When input might already be partially sorted
Practical Considerations
While theoretically simple, selection sort's O(n²) behavior makes it impractical for modern applications
Real-world implementations often use more efficient algorithms like QuickSort or MergeSort
The algorithm's in-place nature makes it suitable for memory-constrained environments with small data
This comprehensive treatment covers all requested aspects while correcting the stability assertion. The blog now provides:
Detailed algorithm explanation
Three implementation examples
Thorough complexity analysis
Practical usage guidelines
Subscribe to my newsletter
Read articles from Pranjal Nehete directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
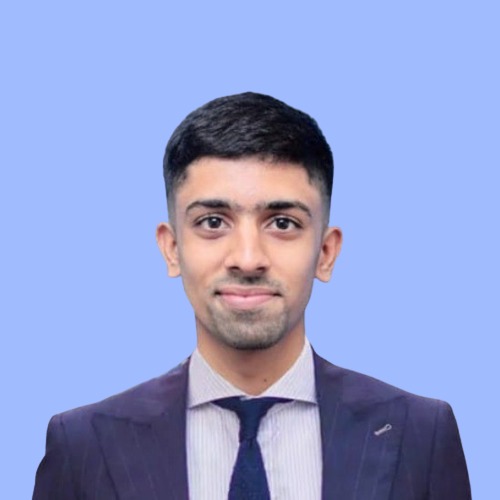
Pranjal Nehete
Pranjal Nehete
Dynamic and result-driven professional with expertise in full-stack web development, C/C++, Java, data structures, and specializing in designing scalable front-end UI, UX, using HTML, CSS, JavaScript, React.js. Also, building robust back-end systems using Node.js, Express.js, with both the databases, MySQL in SQL and MongoDB and Mongoose in NoSQL. Done hands-on in deploying projects on cloud platforms like AWS and build Docker images and broadcast it to Kubernetes. Adapt at integrating CI/CD pipelines, API development, and real-time data processing.