🧰 Functions and Modules in Python: A Practical Guide
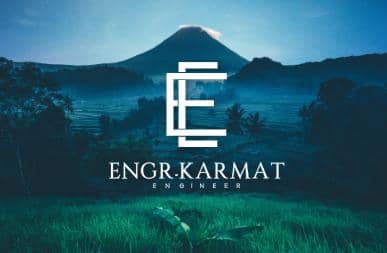
3 min read

🔍 Introduction
In Python, functions and modules are essential tools for writing clean, reusable, and maintainable code. Functions allow you to encapsulate logic for repeated use, while modules let you organize related code across multiple files or packages.
In this article, you'll learn:
- How to define and call functions
- The importance of parameters and return values
- Scope and lifetime of variables
- How to import and use modules
- Creating your own modules
🧠 Why Use Functions and Modules?
- Avoid code duplication
- Improve readability
- Enable testing and debugging
- Facilitate collaboration and modular programming
✅ 1. Functions in Python
➤ Defining a Function
def greet(name):
print(f"Hello, {name}!")
➤ Calling a Function
greet("Alice")
📥 Parameters and 📤 Return Values
def add(a, b):
return a + b
result = add(5, 3)
print(result) # Output: 8
🎯 Default Parameters
def greet(name="Guest"):
print(f"Hello, {name}!")
🔄 Variable-Length Arguments
def sum_all(*args):
return sum(args)
print(sum_all(1, 2, 3, 4)) # Output: 10
🌐 Variable Scope
- Local Scope: Defined inside the function
- Global Scope: Defined outside any function
x = 5
def func():
x = 10 # local
print(x)
func() # 10
print(x) # 5
📦 2. Modules in Python
Modules are simply Python files (.py) containing functions, variables, and classes.
➤ Importing a Module
import math
print(math.sqrt(16)) # Output: 4.0
➤ Importing Specific Elements
from math import pi, sin
print(pi) # Output: 3.14159...
print(sin(pi / 2)) # Output: 1.0
➤ Creating Your Own Module
- Create a file
mymath.py
:
def square(x):
return x * x
- Use it in another file:
import mymath
print(mymath.square(5)) # Output: 25
📁 Built-in vs Third-Party Modules
- Built-in:
math
,os
,random
,datetime
, etc. - Third-party: Install using
pip
, e.g.,requests
,numpy
,pandas
.
pip install requests
🧪 Practice Example: Temperature Converter
# temp_converter.py
def celsius_to_fahrenheit(c):
return (c * 9/5) + 32
def fahrenheit_to_celsius(f):
return (f - 32) * 5/9
# main.py
import temp_converter
print(temp_converter.celsius_to_fahrenheit(100)) # 212.0
🔁 Summary
Concept | Description |
Function | Block of reusable code |
Parameters | Inputs to a function |
Return | Outputs from a function |
Module | Python file with reusable code |
Import | Load modules into your script |
🧩 Conclusion
Functions and modules are fundamental to writing organized Python code. They help you structure your code better, promote reusability, and make debugging easier. Start using functions to break problems into pieces and modules to share logic across files.
🧠 Practice Ideas
- Create a module for basic arithmetic operations.
- Write a function that returns the factorial of a number.
- Create a module that formats dates in different styles.
3
Subscribe to my newsletter
Read articles from Abdulkareem Abdulmateen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
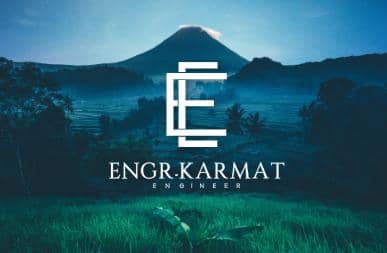