Week 1: Deploying a Minimal Go App on Kubernetes: My Local Setup Journey
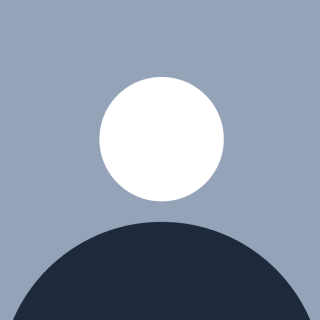
2 min read

After a long break, I'm diving back into Kubernetes โ this time by deploying a tiny Go app locally. If you're learning Kubernetes or want a minimal setup to play with, this post walks you through deploying a Go app using either Docker Desktop or Minikube.
1. Step 1: Write a Simple Go App
// main.go
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello from Go on Kubernetes!")
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
2. Step 2: Multi-stage Dockerfile
Make sure you have a Go mode file. Build and push the Docker image to Docker Hub.
FROM golang:1.24 AS builder
#maximum version supported by tidy (should match with go.mod file or less)
WORKDIR /app
COPY go.mod ./
RUN go mod tidy
COPY . .
RUN CGO_ENABLED=0 GOOS=linux go build -o server .
# Final image
FROM alpine:latest
WORKDIR /root/
COPY --from=builder /app/server /
EXPOSE 8080
CMD ["/server"]
3. Step 3: Kubernetes YAML files
Use the same name as the Docker repo name in Container/image.
deployment.yaml
:
apiVersion: apps/v1
kind: Deployment
metadata:
name: go-hello-app
spec:
replicas: 2
selector:
matchLabels:
app: go-hello
template:
metadata:
labels:
app: go-hello
spec:
containers:
- name: go-app
image: <Username>/<repo-name>:<tag>
imagePullPolicy: Always
ports:
- containerPort: 8080
service.yaml
:
apiVersion: v1
kind: Service
metadata:
name: go-service
spec:
selector:
app: go-app
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: ClusterIP
4. Step 4: Apply the YAML files
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
kubectl get pods
kubectl get svc
Then, port-forward the service:
kubectl port-forward service/go-service 8080:80
Access your app at: http://localhost:8080
Conclusion
This is just the beginning โ my goal is to keep iterating weekly and explore AWS EKS, ConfigMaps, Secrets, scaling, Helm, and more.
If you're starting, too, follow along and feel free to share your tips!
Follow me on LinkedIn for weekly updates and technical breakdowns.
More posts coming soon! ๐
0
Subscribe to my newsletter
Read articles from Shorya Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Kubernetescontainer orchestrationcontainersmulti stage docker fileStarting Pointdiscrete Docker image
Written by
Shorya Dwivedi
Shorya Dwivedi
๐ Exploring Cloud, DevOps, and all things Kubernetes. ๐ Learning in public, one command at a time. ๐ Currently revisiting Kubernetes & sharing weekly updates. ๐ก Building, breaking, fixing โ and documenting the process.