Getting Started with AWS CDK: My First Infrastructure as Code Project 🏗️
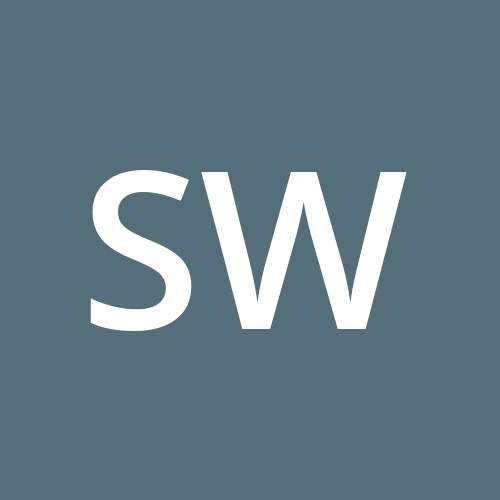

🧠 I built a Lambda, S3 bucket, and SQS queue using AWS CDK—here’s what I learned.
In my recent project, I had the opportunity to work with AWS CDK (Cloud Development Kit) for the first time, and it completely changed the way I think about cloud infrastructure.
Instead of creating AWS resources manually through the console, I learned how to define everything using code. This approach—called Infrastructure as Code (IaC)—is not only faster, but also cleaner, more consistent, and easier to version and share.
In this post, I want to walk you through what I built: a simple AWS stack with:
A Lambda function
An S3 bucket
An SQS queue
These three services formed the foundation of my serverless app, and writing them in code helped me understand AWS better than any tutorial ever did.
🔧 Step 1: Setting Up My CDK Project
I started by installing the AWS CDK and initializing a new TypeScript project:
npm install -g aws-cdk
cdk init app --language typescript
Then I added the libraries for the services I needed:
npm install @aws-cdk/aws-lambda @aws-cdk/aws-s3 @aws-cdk/aws-sqs
At first, it felt a bit overwhelming, but once I saw how things fit together, it really started to click.
🧱 Step 2: Building the Stack
Here’s the core of what I built, inside lib/simple-infra-stack.ts
.
import * as cdk from 'aws-cdk-lib';
import { Construct } from 'constructs';
import * as lambda from 'aws-cdk-lib/aws-lambda';
import * as s3 from 'aws-cdk-lib/aws-s3';
import * as sqs from 'aws-cdk-lib/aws-sqs';
export class SimpleInfraStack extends cdk.Stack {
constructor(scope: Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
🪣 Creating an S3 Bucket
const bucket = new s3.Bucket(this, 'MyBucket', {
bucketName: 'my-simple-iac-bucket',
removalPolicy: cdk.RemovalPolicy.DESTROY,
autoDeleteObjects: true,
});
At first, I didn’t realize how useful removalPolicy
and autoDeleteObjects
were. Since I was experimenting, it saved me a lot of time when I needed to clean up resources.
📨 Setting Up an SQS Queue
const queue = new sqs.Queue(this, 'MyQueue', {
queueName: 'my-iac-queue',
});
I used this queue later to decouple services. Just knowing that it’s easy to drop in an SQS queue for scalability was eye-opening.
⚙️ Writing My First Lambda Function
const lambdaFn = new lambda.Function(this, 'MyLambdaFunction', {
runtime: lambda.Runtime.NODEJS_18_X,
handler: 'index.handler',
code: lambda.Code.fromInline(`
exports.handler = async function(event) {
console.log("Event received:", JSON.stringify(event));
return {
statusCode: 200,
body: "Hello from Lambda!"
};
};
`),
});
This was the first time I saw how simple it could be to get a Lambda up and running with CDK. I used fromInline()
just to test quickly, but now I understand how to point to real source files as well.
🚀 Step 3: Deploying to AWS
After writing my stack, I compiled and deployed it:
npm run build
cdk deploy
It was incredible to see all three resources—bucket, queue, and Lambda—created in just a few seconds. I didn’t touch the AWS Console once.
🧹 Cleaning Up
To avoid charges during testing, I cleaned up with:
cdk destroy
That one command deleted everything cleanly, including the objects in my S3 bucket thanks to the removal policy.
✅ Final Thoughts
This was a real learning moment for me. I realized how powerful Infrastructure as Code is—especially with CDK. Here's a quick recap of which services I used and for what purpose:
AWS Service | Why I Used It |
Lambda | To run serverless backend logic |
SQS | To queue the messages |
S3 Bucket | To store files processed from the Queue |
Subscribe to my newsletter
Read articles from Sudhanshu Wani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
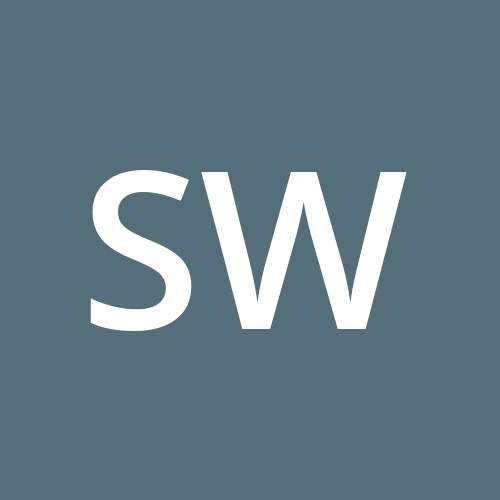