🚀 How to Use SVG Images in Flutter? | Complete Guide 🖼️
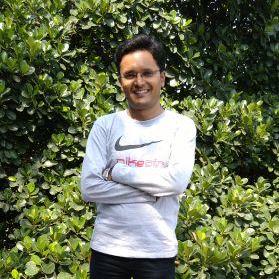

Introduction:
In this article, we will explore the process of incorporating SVG (Scalable Vector Graphics) images into a Flutter application.
Installation:
By utilizing, flutter_svg package, developers can effortlessly integrate SVG images in Flutter application. To add flutter_svg package to our Flutter application, open up pubspec.yaml
file and add below code.
dependencies:
flutter_svg: ^2.0.17
Save the changes and execute below command in terminal to install the package in our Flutter application.
flutter pub get
How To Use SVG Image From An Asset Folder?
Now the package is installed, we can start using SVG images in our Flutter application.
Create a folder called asset in project’s root directory and add SVG images to that folder.
Now open up
pubspec.yaml
file, add the path of our SVG images under assets section as shown below.flutter: assets: - assets/<filename-with-extension>
If you have multiple images, then just specify the directory name as shown below and save the changes.
flutter: assets: - assets/
Open up main.dart file and use SvgPicture.asset widget to display the SVG image from asset folder as shown below.
class MyHomePage extends StatelessWidget { const MyHomePage({super.key, required this.title}); final String title; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( backgroundColor: Theme.of(context).colorScheme.inversePrimary, title: Text(title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[SvgPicture.asset('assets/<filename-with-extension>')], ), ), ); } }
Save the changes and let’s run the application.
Image will get displayed as shown below.
How To Use SVG Image From Internet?
Displaying SVG image from internet is super easy. Instead of SvgPicture.asset widget, we use SvgPicture.network widget and instead of image path, we use image URL as shown below.
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SvgPicture.network('<image-url>')
],
),
),
);
}
}
Save the changes and run the application. While using SVG image from internet, just make sure your internet connection is active.
How To Add Filtering Effect To SVG Image?
The SvgPicture widget has various properties that you can use to customize the look of SVG image. For instance, you can change the width and height of the image, as well as apply a filtering effect.
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SvgPicture.network(
"<image-url>",
width: 200,
height: 200,
colorFilter: ColorFilter.mode(Colors.amber, BlendMode.darken),
)
],
),
),
);
}
}
Save the changes and let’s run the application. The image will look like this:
I have also created a YouTube video regarding this topic, so if you would like to watch a video tutorial, then please go to https://youtu.be/1ujNbxNbZEc.
Conclusion:
In conclusion, incorporating SVG images into a Flutter application is a straightforward process with the help of the flutter_svg package. Whether you're using SVG images from an asset folder or directly from the internet, SvgPicture widget provides flexible options to display and customize SVG images. By following the steps outlined in this guide, you can enhance your application's visual appeal with scalable and high-quality graphics. I hope this article helped!
Happy coding!
Subscribe to my newsletter
Read articles from Manoj Kulkarni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
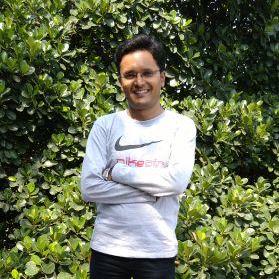
Manoj Kulkarni
Manoj Kulkarni
I am programmer, blogger and foodie. I just love computers, coding and technical things. I spend most of my free time working on building my skill set by reading technical articles about new technologies or books on software development and try to keep my self updated as much as I can.