Mastering Python Lists: From Basics to Common Operations
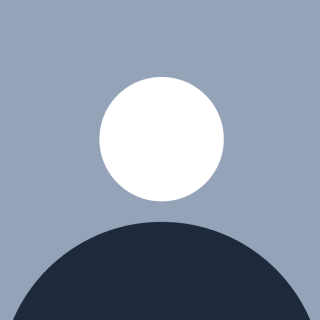

Hey friends! 👋
If you’ve just started learning Python, chances are you've already met lists. And if you haven't, you're about to meet one of the most useful features in the entire language.
In this blog, I'm going to walk you through Python lists in a super simple and beginner-friendly way. No jargon. Just the basics, examples, and common operations you’ll actually use.
🧠 What’s a List in Python?
A list in Python is a collection of items—you can store numbers, strings, or even other lists.
Let me show you a basic one:
pythonCopy codefruits = ["apple", "banana", "cherry"]
Now we’ve got a list named fruits
with 3 elements. You can access them by index.
pythonCopy codeprint(fruits[0]) # apple
print(fruits[2]) # cherry
✅ Key Features of Lists
Ordered 🟰 items stay in the order you add them
Mutable 🔄 you can change them anytime
Allows duplicates 🙃
Can hold mixed data types (yes, even lists inside lists!)
🔧 Common List Operations You’ll Use a Lot
1. Adding Items
Use .append()
to add an item at the end:
pythonCopy codefruits.append("orange")
Or .insert()
to add it at a specific position:
pythonCopy codefruits.insert(1, "grape") # insert at index 1
2. Removing Items
pythonCopy codefruits.remove("banana") # removes by value
fruits.pop(0) # removes by index
To remove all items:
pythonCopy codefruits.clear()
3. Updating Items
pythonCopy codefruits[0] = "mango"
Simple and direct—lists love being changed.
🔁 Looping Through a List
You’ll often want to go through each item. Here’s how:
pythonCopy codefor fruit in fruits:
print(fruit)
Want the index too? Use enumerate()
:
pythonCopy codefor i, fruit in enumerate(fruits):
print(i, fruit)
📐 Slicing and Indexing
Python lists are super flexible when it comes to grabbing parts of the list:
pythonCopy codenumbers = [10, 20, 30, 40, 50]
print(numbers[1:4]) # [20, 30, 40]
print(numbers[-1]) # 50 (last item)
🔄 List Methods You Should Know
Here are some list methods that will make your life easier:
Method | What it does |
append() | Add item at the end |
insert() | Add item at specific index |
remove() | Remove by value |
pop() | Remove by index |
sort() | Sorts the list (default: ascending) |
reverse() | Reverses the list |
count() | Counts occurrences of a value |
🔍 Bonus: List Comprehension
This is one of the coolest Python features. It lets you create a new list in one line:
pythonCopy codesquares = [x**2 for x in range(5)]
print(squares) # [0, 1, 4, 9, 16]
🧼 Final Thoughts
Lists in Python are incredibly powerful. Once you get a good grip on them, you’ll find yourself using them everywhere—from simple data storage to complex algorithms.
So go ahead, create lists, play with them, break them, and rebuild. That's how you master them. 😄
Subscribe to my newsletter
Read articles from abhishek Gaud directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by