Tuples Demystified: The Power of Immutable Data in Python
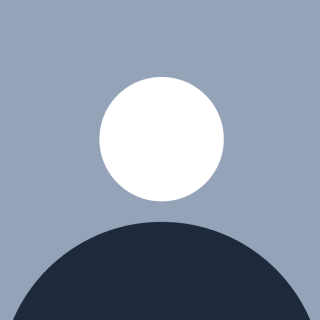

Hey Python folks! 👋
You’ve probably heard of tuples and might’ve wondered—why use them when we already have lists? Well, let’s unravel the mystery together.
By the end of this post, you'll understand:
What tuples are
Why they’re immutable
When to use them (and not use them)
And how they behave in memory
📦 What’s a Tuple?
A tuple is just like a list—but with one big difference:
It cannot be changed.
pythonCopy codecoordinates = (10, 20)
print(coordinates[0]) # 10
Try changing it?
pythonCopy codecoordinates[0] = 15 # ❌ Error: 'tuple' object does not support item assignment
Boom. Python stops you right there. That’s the immutability at work.
🧠 Why Use Tuples?
Here’s why tuples are more than just “frozen lists”:
✅ Safety
You use tuples when your data should not change—like coordinates, fixed settings, or database rows.🚀 Speed
Tuples are slightly faster than lists in Python. Immutability helps with optimization.🔒 Hashable
Tuples can be used as dictionary keys, unlike lists.
pythonCopy codelocation = {(10, 20): "Park"}
print(location[(10, 20)]) # Park
🧮 Tuple vs List (in memory)
Let’s break it down visually:
🧪 Tuple Examples in Real Python Code
✅ Storing coordinates:
pythonCopy codepoint = (5, 8)
✅ Returning multiple values from a function:
pythonCopy codedef get_stats():
return (75, "Pass")
marks, status = get_stats()
✅ Dictionary keys:
pythonCopy codestudent_grades = {("Anu", "Math"): 95}
🎯 When Not to Use Tuples
If your data might need to change
If you’re working with long, dynamic collections
If readability and clarity are better with list-style operations (
.append()
,.remove()
, etc.)
🤔 Can Tuples Hold Mutable Objects?
Yup. The tuple itself is immutable, but it can hold mutable items inside:
pythonCopy codedata = ([1, 2], 3)
data[0].append(4)
print(data) # ([1, 2, 4], 3)
Wild, right?
✅ Final Thoughts
Tuples are like the locked boxes of Python—you store values that shouldn’t change and trust they’ll stay put. Once you know when and how to use them, you’ll write cleaner, safer, and faster code.
Next time you’re reaching for a list, just ask:
“Do I really need to change this data?”
If not, tuple up! 😄
Subscribe to my newsletter
Read articles from abhishek Gaud directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by