Master Lottie Animations in React: A Guide for Real Projects
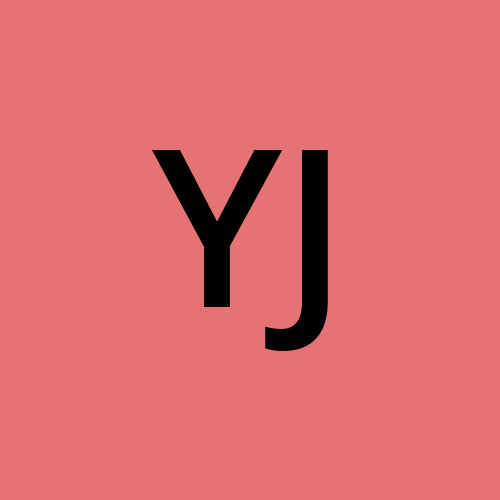

Okay so you’re like me and you’ve seen several cool animations on the web and were like ‘Sick!’ and always wanted to create and use them in personal projects. Well there is a simple way to do it using Lottie (plus lottie-react).
Lottie is a library created by Airbnb built for development across Web, Mobile and Windoes that parses Adobe After Effects animations exported as JSON’. That just means it translates complex animations into simple JSON and renders that animation on the platform you are developing on. It’s great because it’s lightweight and won’t slow down your apps, scales well and is very designer friendly, i’d say it’s even dev- friendly too.
To get started, get the package using yarn or npm
npm i lottie-react
OR
yarn add lottie-react
You can get some free animations from the lottie website or creating one using their custom animation creator here. For this tutorial, I will use one of the free ones, specifially thisone.
After downloading the animation you import it into your react file as shown below.
import Lottie from 'lottie-react'
import animationData from './animation.json'
const MyComponent = () => {
return(
<div>
<Lottie animationData={animationData} />
</div>
)
}
After doing this correctly, when you fire app your project in the browser, you should see your swinging animation in motion! The image below shows what it should look like.
Once you’ve embedded a Lottie animation in your React component, the next thing you'll likely want is fine-grained control: when should the animation start, should it loop, can the user control it, and what if you want it to pause on a certain frame?
Thankfully, the lottie-react
library exposes several props and methods for this.
🧩 Commonly Used Props in <Lottie />
Prop | Type | Description |
animationData | object | The Lottie JSON file. Required. |
loop | boolean | Whether the animation should repeat. Default is true . |
autoplay | boolean | Should the animation play as soon as it mounts? Default is true . |
style | object | Inline styles (e.g., width, height). |
className | string | Optional CSS class for styling. |
lottieRef | React.MutableRefObject | Reference for programmatic control (play , pause , stop , etc). |
🔧 Example: Basic Controlled Animation
import { useRef } from 'react'
import Lottie from 'lottie-react'
import rocket from './rocket.json'
export default function RocketAnimation() {
const lottieRef = useRef()
return (
<div>
<Lottie
animationData={rocket}
loop={false}
autoplay={false}
lottieRef={lottieRef}
style={{ width: 300, height: 300 }}
/>
<div className="controls">
<button onClick={() => lottieRef.current?.play()}>Play</button>
<button onClick={() => lottieRef.current?.pause()}>Pause</button>
<button onClick={() => lottieRef.current?.stop()}>Stop</button>
</div>
</div>
)
}
🛑 stop()
vs pause()
Method | Behavior |
stop() | Resets animation back to frame 0 and halts it |
pause() | Freezes animation at current frame |
play() | Resumes animation from current frame (or from beginning if it was stopped) |
goToAndStop(frame, isFrame)
Use this to jump to a specific point in the animation and freeze there.
// Go to frame 120 and stop
lottieRef.current?.goToAndStop(120, true)
frame
: The target frame numberisFrame
: Iftrue
, interprets120
as a frame number. Iffalse
, it's a time in milliseconds
Want to stop on the last frame? Use:
const lastFrame = lottieRef.current?.animationData.op
lottieRef.current?.goToAndStop(lastFrame, true)
Handling Completion Events
To run logic when the animation ends:
useEffect(() => {
const anim = lottieRef.current
if (!anim) return
const onComplete = () => {
console.log('Animation done!')
}
anim.addEventListener('complete', onComplete)
return () => anim.removeEventListener('complete', onComplete)
}, [])
Best Practices
Use
autoplay={false}
+ manualplay()
if you want full controlCombine with
sessionStorage
or React Context to prevent repeated play on navigationAvoid overlapping Lottie animations with multiple running in the DOM they’re lightweight, but not free
❗ Common Issues to Watch Out For
While Lottie is a powerful tool, there are a few things to watch out for when integrating it into React projects especially if you're aiming for polished, production-ready behavior.
Animations replay when revisiting a page
One common frustration is that animations replay every time you navigate back to a page. This happens because the component remounts and resets its state.
A simple fix is to store a flag in sessionStorage
after the animation plays once. On future visits, you can jump to the final frame using .goToAndStop()
:
useEffect(() => {
const anim = lottieRef.current
const alreadyPlayed = sessionStorage.getItem('played') === 'true'
if (alreadyPlayed) {
anim.goToAndStop(anim?.animationData.op, true)
} else {
anim.play()
anim.addEventListener('complete', () => {
sessionStorage.setItem('played', 'true')
})
}
}, [])
This ensures the animation plays once and stays in its final state unless you explicitly reset it.
The lottieRef
prop isn’t full control
While lottie-react
gives you a convenient lottieRef
, it's not the full lottie-web
instance. For more advanced use cases (like timeline scrubbing or syncing with scroll), you may want to use useLottie()
or import lottie-web
directly for complete control.
Animations may not be fully responsive
Depending on how your animation was exported, it might not scale properly across screen sizes. You can control its dimensions using styles or Tailwind classes like:
style={{ width: '100%', height: 'auto' }}
Be sure to test your layout at different breakpoints.
Event listeners can cause issues if not cleaned up
If you're attaching event listeners (like onComplete
), make sure to remove them when the component unmounts. Otherwise, you may end up with duplicate calls or memory issues.
return () => {
animation?.removeEventListener('complete', onComplete)
}
Running too many Lotties at once can slow things down
Even though Lottie animations are vector-based and lightweight, performance can suffer if you have multiple complex animations running simultaneously. If they’re not visible, pause them or remove them from the DOM until needed.
Wrapping Up
Lottie is one of those tools that bridges the gap between design and development in a seamless way. With just a few lines of code, you can bring beautiful, high-quality animations into your React apps no need for heavy video files or complicated keyframes.
Whether you’re building a landing page, onboarding flow, or micro-interaction, Lottie gives you both the flexibility and the polish to do it right.
Here are a few final tips:
Use
autoplay={false}
if you want to control when animations startPrevent unintended replays using
sessionStorage
and.goToAndStop()
For deeper control, explore
useLottie()
orlottie-web
directlyKeep it performant: animate only what’s needed
Animations, when done right, create moments of delight. With Lottie, you get the power to do that without compromising speed or developer sanity.
Subscribe to my newsletter
Read articles from Adeyinka Junaid Hafiz directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
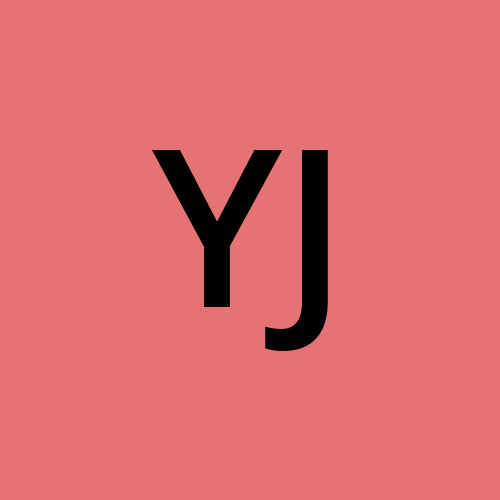