Master JavaScript by Building a Tic Tac Toe Game: A Hands-On Project Approach
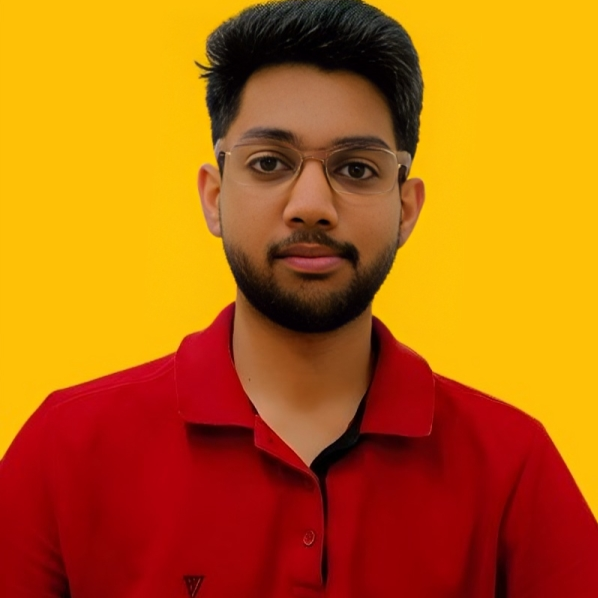

Introduction
Welcome to this JavaScript beginner's guide where we dive deep into building a Tic Tac Toe game! This project will not only help you learn the essentials of JavaScript but also give you a practical example of how to handle game logic, events, and UI manipulation.
In this blog, we’ll walk through the process of creating a fully functional Tic Tac Toe game, detailing the JavaScript methods and functions used to manage game state, player turns, scoring, and the overall user experience.
Why Build a Tic Tac Toe Game?
Building a Tic Tac Toe game using JavaScript allows you to apply fundamental programming concepts in a fun and engaging way. Here’s why this project is beneficial:
Fundamental Concepts: You’ll get hands-on experience with essential JavaScript concepts like variables, functions, loops, conditionals, and event listeners.
Interactive Learning: You’ll see how your code interacts with the game’s user interface (UI) to dynamically update the board and switch between player turns.
Problem Solving: You’ll implement logic to check for winning conditions, detect a draw, and handle user input, giving you a deeper understanding of JavaScript.
Project Setup
Tools and Technologies
HTML/CSS: Used to structure and style the game layout and visual elements.
JavaScript: This is where the core functionality of the game lives, handling player turns, detecting winners, and updating the UI.
Prerequisites
To follow along with this project, you should be familiar with the basics of HTML, CSS, and JavaScript. You should also have a basic understanding of DOM manipulation and event handling.
Building the Game
Step 1: Create the Game Board
In the first step, we create a 3x3 grid for the Tic Tac Toe board using HTML. Here’s a sample code snippet:
<div class="grid-box grid grid-cols-3 grid-rows-3 gap-3 mt-16">
<div class="grid-item bg-grid-box hover:bg-grid-box-hover"></div>
<div class="grid-item bg-grid-box hover:bg-grid-box-hover"></div>
<div class="grid-item bg-grid-box hover:bg-grid-box-hover"></div>
...
</div>
The grid-item
elements represent the individual boxes in the game where players can place their moves (X or O).
Step 2: Implement Game Logic
The core of the game logic is implemented in JavaScript. Let's break it down into key functions:
initGame()
The initGame()
function resets the game board when a new game starts, initializing the player turn to "X" and clearing any previous marks from the grid.
function initGame(){
currentPlayer="X";
gameGrid=["","","","","","","","",""];
boxes.forEach((box) => {
box.innerText = ""; // clear the box content
box.style.pointerEvents = "all"; // re-enable clicking
});
newGameBtn.innerText=`Player - ${currentPlayer} turn`;
}
- Explanation: This function is responsible for setting up the initial game state. It clears the grid, resets the scores, and sets the current player to "X".
swapTurn()
This function switches turns between Player X and Player O:
javascriptCopyEditfunction swapTurn(){
currentPlayer = currentPlayer === "X" ? "O" : "X";
newGameBtn.innerText=`Player - ${currentPlayer} turn`;
}
- Explanation: It checks if the current player is "X" and changes the turn accordingly. It also updates the button that displays the current player's turn.
checkGameOver()
This function checks whether the game has ended, either with a win or a draw:
function checkGameOver(){
let winner = "";
winningPosition.forEach((position) => {
if (gameGrid[position[0]] === gameGrid[position[1]] && gameGrid[position[1]] === gameGrid[position[2]] && gameGrid[position[0]] !== "") {
winner = gameGrid[position[0]];
// Disable further clicks
boxes.forEach((box) => box.style.pointerEvents = "none");
}
});
// Check for a draw
if (gameGrid.every(cell => cell !== "")) {
// Handle draw scenario
}
}
- Explanation: This function checks the 8 winning positions on the grid and determines if a player has won. It also handles the draw condition when all cells are filled but no winner is found.
Step 3: Add Interactivity
Event listeners are used to make the game interactive. The handleClick()
function is called when a player clicks on a grid box:
function handleClick(index){
if (gameGrid[index] === "") {
boxes[index].innerText = currentPlayer;
gameGrid[index] = currentPlayer;
boxes[index].style.pointerEvents = "none";
swapTurn();
checkGameOver();
}
}
boxes.forEach((box, index) => {
box.addEventListener("click", () => handleClick(index));
});
- Explanation: This function updates the game grid based on the player's move, disables the clicked box, and then checks if the game is over.
Testing and Debugging
After implementing the game logic, it’s essential to test your game thoroughly:
Check Player Turns: Ensure the turns alternate between Player X and Player O.
Winning Logic: Verify that the winning conditions are correctly handled, and the winner is displayed.
Draw Detection: Test if the game correctly detects a draw.
UI Updates: Ensure that the game UI reflects changes, like player moves, turn switching, and score updates.
Project Links
You can explore the full source code and try out the live version of the Tic Tac Toe game here:
💻 GitHub Repository: View Code on GitHub
🚀 Live Demo: Play the Game Live
Feel free to fork the repo, experiment with the code, or even contribute improvements!
Learn More About JavaScript
If you’re interested in deepening your JavaScript knowledge beyond this project, here are some excellent resources to help you continue learning:
📘 MDN Web Docs - JavaScript Guide: The official and comprehensive guide to JavaScript.
🎓 JavaScript Tutorial by W3Schools: Beginner-friendly tutorials with interactive code snippets.
📺 freeCodeCamp JavaScript Course (YouTube): A complete beginner-friendly JavaScript course on YouTube.
These resources will help you master JavaScript and prepare you for more advanced projects like building web apps using frameworks like React or working with APIs.
Conclusion
By completing this Tic Tac Toe project, you have gained a solid understanding of JavaScript programming concepts. You’ve learned how to manage game logic, update the UI dynamically, and handle user interactions using JavaScript.
What You Learned:
How to manipulate the DOM using JavaScript.
How to manage game state and switching between players.
How to detect game outcomes (win or draw).
Event handling and dynamically updating the UI based on player actions.
Call to Action
Now that you’ve built the Tic Tac Toe game, it’s time to challenge your friends to a match! Continue to explore more advanced JavaScript concepts and expand your skills by building more complex games or web applications. The more you practice, the better you'll get at coding and problem-solving.
Share your project on GitHub or Vercel and showcase your work to the world. Happy coding!
Contact Information
Want to connect or contact me? Feel free to hit me up on the following:
Twitter / X: @I_am_Chirag28
LinkedIn: @Chiragtyagi01
Subscribe to my newsletter
Read articles from Chirag Tyagi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
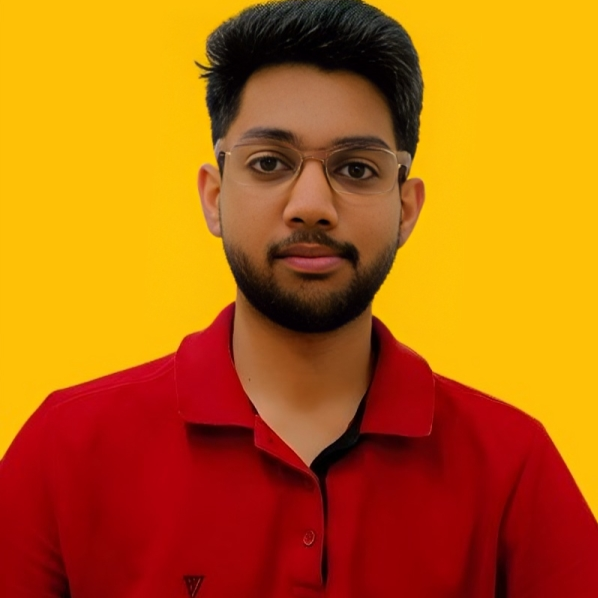
Chirag Tyagi
Chirag Tyagi
Profile Bio I’m currently pursuing B.Tech in Computer Science and Engineering at Roorkee Institute of Technology, where I am deeply engaged in learning full-stack development with the MERN stack. My passion for technology drives me to explore and stay updated with the latest advancements in the field. Eager to apply and expand my skills, I am committed to continuous learning and growth in the ever-evolving tech landscape.