Exploring the Power of Ruby on Rails
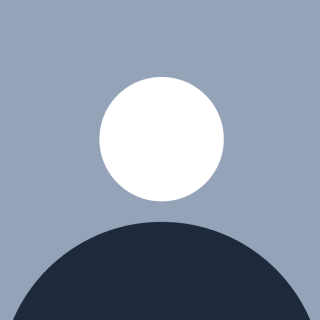

Exploring the Power of Ruby on Rails
Ruby on Rails (often called Rails) is a powerful, developer-friendly web application framework written in Ruby. Since its release in 2004, it has revolutionized web development by emphasizing convention over configuration (CoC) and don’t-repeat-yourself (DRY) principles. In this article, we’ll explore why Rails remains a top choice for developers, its key features, and how it accelerates web development.
Why Ruby on Rails?
Rails is designed for productivity. Its elegant syntax and built-in conventions allow developers to focus on solving business problems rather than configuring files. Here are some reasons why Rails is still relevant in 2024:
Rapid Development – With built-in tools like scaffolding, ActiveRecord, and seamless database integration, Rails enables developers to build applications quickly.
Strong Community Support – The Rails community is active, with numerous gems (libraries) available to extend functionality.
Scalability – Companies like Shopify, GitHub, and Airbnb use Rails at scale, proving its robustness.
Security – Rails includes built-in protections against SQL injection, cross-site scripting (XSS), and CSRF attacks.
Key Features of Ruby on Rails
1. Convention Over Configuration (CoC)
Rails minimizes configuration by assuming default structures. For example, if you have a User
model, Rails automatically links it to a users
table in the database.
ruby
Copy
Download
# app/models/user.rb
class User < ApplicationRecord
end
No need to specify table names or primary keys—Rails handles it.
2. ActiveRecord (ORM)
ActiveRecord simplifies database interactions by allowing developers to use Ruby instead of SQL.
ruby
Copy
Download
# Fetch all users
users = User.all
# Find a user by ID
user = User.find(1)
# Create a new user
User.create(name: "John Doe", email: "john@example.com")
3. RESTful Routing
Rails encourages RESTful architecture, making APIs clean and intuitive.
ruby
Copy
Download
# config/routes.rb
Rails.application.routes.draw do
resources :articles
end
This single line generates CRUD routes (GET /articles
, POST /articles
, etc.).
4. Built-in Testing (MiniTest/RSpec)
Rails promotes test-driven development (TDD) with built-in testing frameworks.
ruby
Copy
Download
# test/models/user_test.rb
require 'test_helper'
class UserTest < ActiveSupport::TestCase
test "should not save user without email" do
user = User.new
assert_not user.save, "Saved user without an email"
end
end
5. Asset Pipeline & Webpacker
Rails efficiently manages JavaScript, CSS, and images via the asset pipeline and Webpacker.
Real-World Applications of Rails
1. Shopify
One of the largest e-commerce platforms, Shopify, runs on Rails, handling millions of transactions daily.
2. GitHub
GitHub’s backend was originally built on Rails, showcasing its ability to manage large-scale applications.
3. Airbnb
Airbnb uses Rails for its core booking and search functionalities.
Getting Started with Rails
To create a new Rails app:
bash
Copy
Download
gem install rails
rails new myapp
cd myapp
rails server
Visit http://localhost:3000
to see your app running.
When Should You Use Rails?
Startups – Fast prototyping and MVP development.
SaaS Applications – Scalable and maintainable architecture.
APIs – Rails works well as a backend for React, Vue, or mobile apps.
Boosting Your Online Presence
If you're a developer looking to grow your YouTube channel or social media presence, consider MediaGeneous, a fantastic platform for social media promotion and marketing. They specialize in helping content creators expand their reach effectively.
Conclusion
Ruby on Rails remains a dominant force in web development due to its simplicity, speed, and scalability. Whether you're building a small project or a large-scale application, Rails provides the tools needed to succeed.
Want to dive deeper? Check out the official Rails guide or explore RubyGems for useful libraries.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from MediaGeneous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by