DevOps Mini Project: CI/CD Pipeline for a Node.js App Using Jenkins and Docker
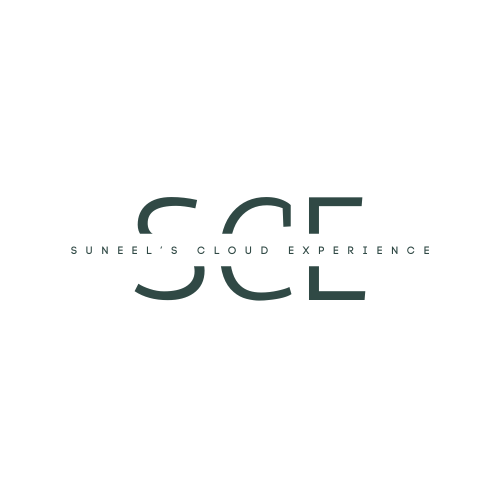
Table of contents
- ๐ ๏ธ Prerequisites:
- ๐ 1. Create a Simple Node.js App
- ๐ณ 2. Create Dockerfile
- ๐ค 3. Add Jenkinsfile for Pipeline
- ๐ Note: In Jenkins > Credentials, add your DockerHub credentials with ID dockerhub-creds.
- โ๏ธ 5. Set Up Jenkins Pipeline Job
- ๐ 6. Setup Webhook (Optional)
- โ 7. Final Output

In this blog, weโll walk through setting up a CI/CD pipeline for a simple Node.js application using Jenkins, Docker, and GitHub.
๐ ๏ธ Prerequisites:
Jenkins installed (locally or on an EC2 instance)
Docker installed and running
DockerHub account
GitHub account
๐ 1. Create a Simple Node.js App
Folder Structure:
nodejs-app/
โโโ Dockerfile
โโโ Jenkinsfile
โโโ package.json
โโโ index.js
๐ index.js
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.get('/', (req, res) => {
res.send('Hello from DevOps CI/CD Pipeline!');
});
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
๐ package.json
{
"name": "nodejs-app",
"version": "1.0.0",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"dependencies": {
"express": "^4.17.1"
}
}
๐ณ 2. Create Dockerfile
๐ Dockerfile
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
๐ค 3. Add Jenkinsfile for Pipeline
๐ Jenkinsfile
pipeline {
agent any
environment {
DOCKER_IMAGE = 'yourdockerhubusername/nodejs-app'
}
stages {
stage('Clone Repo') {
steps {
git 'https://github.com/yourusername/nodejs-app.git'
}
}
stage('Build Docker Image') {
steps {
script {
sh 'docker build -t $DOCKER_IMAGE .'
}
}
}
stage('Push to DockerHub') {
steps {
withCredentials([usernamePassword(credentialsId: 'dockerhub-creds', usernameVariable: 'USERNAME', passwordVariable: 'PASSWORD')]) {
sh """
echo "$PASSWORD" | docker login -u "$USERNAME" --password-stdin
docker push $DOCKER_IMAGE
"""
}
}
}
stage('Deploy Container') {
steps {
sh "docker run -d -p 3000:3000 $DOCKER_IMAGE"
}
}
}
}
๐ Note: In Jenkins > Credentials, add your DockerHub credentials with ID dockerhub-creds
.
โ๏ธ 4. Push Code to GitHub
Create a GitHub repo and push all your code:
git init
git remote add origin https://github.com/yourusername/nodejs-app.git
git add .
git commit -m "Initial commit"
git push -u origin main
โ๏ธ 5. Set Up Jenkins Pipeline Job
Open Jenkins dashboard.
Click New Item โ Pipeline โ Give a name.
In the pipeline configuration:
Choose Pipeline script from SCM
SCM: Git
Repository URL:
https://github.com/yourusername/nodejs-app.git
Branch:
main
Script Path:
Jenkinsfile
Click Save and then Build Now.
๐ 6. Setup Webhook (Optional)
To trigger builds automatically:
Go to your GitHub repo โ Settings โ Webhooks โ Add webhook.
Payload URL:
http://<jenkins-server>:8080/github-webhook/
Content type:
application/json
Events: Just the push event
โ 7. Final Output
After successful build:
Jenkins pulls code โ builds Docker image โ pushes to DockerHub โ runs container.
Access your app at
http://<server-ip>:3000
.
Subscribe to my newsletter
Read articles from Suneel Kumar Kola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
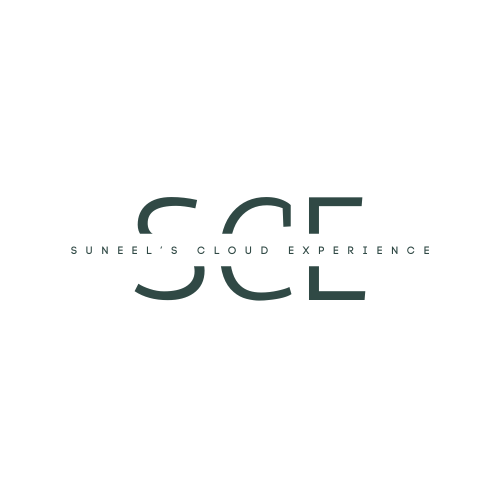