🧱Data Structures in python: Built-in and user-defined.
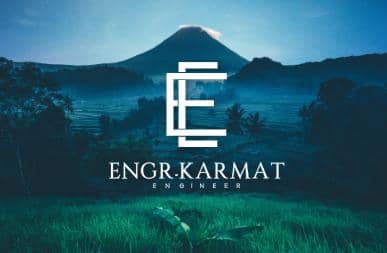
3 min read

🔍 Introduction
A data structure is a way of organizing and storing data so that it can be accessed and modified efficiently. Python provides a rich set of built-in data structures and also allows us to create user-defined ones for more complex operations.
In this guide, you’ll learn:
- The basics of built-in data structures:
lists
,tuples
,sets
, anddictionaries
- How to implement user-defined structures:
stack
,queue
,linked list
,tree
,graph
, andhashmap
✅ 1. Built-in Data Structures
🧾 Lists
- Mutable
- Ordered
- Allows duplicates
fruits = ["apple", "banana", "cherry"]
fruits.append("orange")
print(fruits)
🧊 Tuples
- Immutable
- Ordered
- Allows duplicates
coordinates = (10, 20)
print(coordinates[0])
🔀 Sets
- Mutable
- Unordered
- No duplicates
unique_nums = {1, 2, 2, 3}
unique_nums.add(4)
print(unique_nums)
🧭 Dictionaries
- Mutable
- Key-value pairs
- Unordered (Python 3.6+ keeps insertion order)
person = {"name": "Alice", "age": 25}
print(person["name"])
🛠️ 2. User-defined Data Structures
📚 Stack (LIFO)
stack = []
stack.append(1)
stack.append(2)
stack.pop() # Removes 2
🧾 Queue (FIFO)
from collections import deque
queue = deque()
queue.append(1)
queue.append(2)
queue.popleft() # Removes 1
🔗 Linked List
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
🌳 Tree (Binary Tree)
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
🌐 Graph (Adjacency List)
graph = {
"A": ["B", "C"],
"B": ["A", "D"],
"C": ["A"],
"D": ["B"]
}
🧮 HashMap (Custom Dictionary)
class HashMap:
def __init__(self):
self.map = [None] * 10
def _get_hash(self, key):
return hash(key) % len(self.map)
def add(self, key, value):
key_hash = self._get_hash(key)
self.map[key_hash] = value
📊 Summary Table
Structure | Type | Mutable | Ordered | Unique Items |
List | Built-in | Yes | Yes | No |
Tuple | Built-in | No | Yes | No |
Set | Built-in | Yes | No | Yes |
Dictionary | Built-in | Yes | Yes* | Keys are unique |
Stack | User-defined | Yes | Yes | No |
Queue | User-defined | Yes | Yes | No |
Linked List | User-defined | Yes | Yes | No |
Tree | User-defined | Yes | N/A | N/A |
Graph | User-defined | Yes | N/A | N/A |
HashMap | User-defined | Yes | No | Keys are unique |
🧠 Practice Challenges
- Reverse a list using a stack
- Implement a queue using two stacks
- Traverse a binary tree (in-order, pre-order, post-order)
- Implement Dijkstra's algorithm on a graph
- Create a simple hash map with collision handling
🧩 Conclusion
Choosing the right data structure is key to solving programming problems efficiently. Python gives you both the tools and flexibility to use simple built-ins or build your own from scratch. Practice is the best way to master them!
2
Subscribe to my newsletter
Read articles from Abdulkareem Abdulmateen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
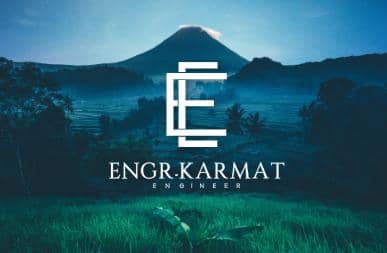