Template Method Pattern in Java: Creating a Blueprint for Subclasses
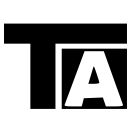
5 min read
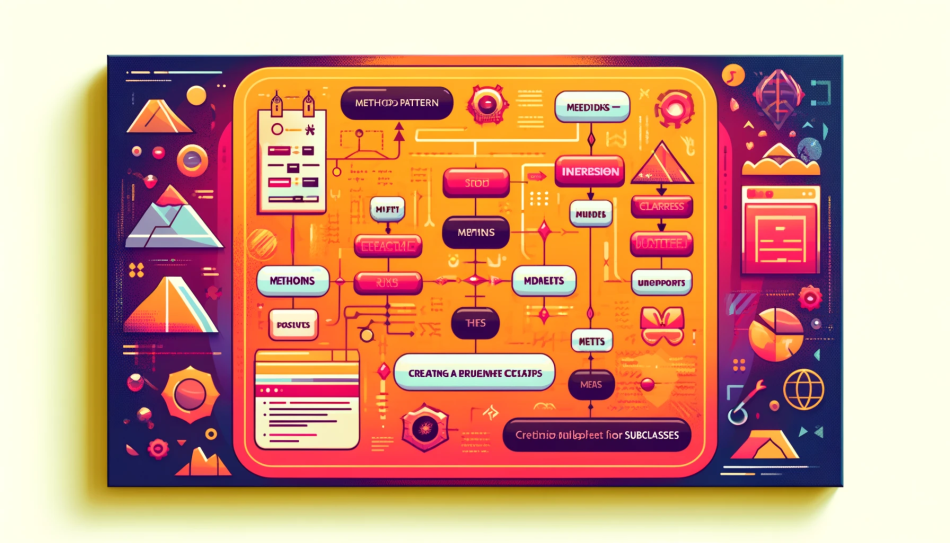
1. What is the Template Method Pattern?
At its core, the Template Method Pattern defines an algorithm's steps in a base class, deferring the implementation of some steps to subclasses. This allows you to enforce a consistent structure across subclasses while giving them freedom to customize specific behaviors.
Key Components:
- Abstract Class: Contains the template method that outlines the algorithm structure.
- Concrete Methods: Common methods that can be reused across subclasses.
- Abstract Methods: Methods that must be implemented by subclasses to provide specific behavior.
- Hook Methods: Optional methods that subclasses can override.
2. Template Method Pattern Example in Java
Let’s look at a practical example where we have a class that processes different types of documents—PDF and Word. We will use the Template Method Pattern to ensure the processing steps remain the same while allowing different formats to define how content is handled.
abstract class DocumentProcessor {
// Template method
public final void processDocument() {
openDocument();
parseContent();
closeDocument();
}
// Steps of the algorithm
protected abstract void openDocument();
protected abstract void parseContent();
// Hook method
protected void closeDocument() {
System.out.println("Closing document.");
}
}
class PdfDocument extends DocumentProcessor {
@Override
protected void openDocument() {
System.out.println("Opening PDF document.");
}
@Override
protected void parseContent() {
System.out.println("Parsing PDF content.");
}
}
class WordDocument extends DocumentProcessor {
@Override
protected void openDocument() {
System.out.println("Opening Word document.");
}
@Override
protected void parseContent() {
System.out.println("Parsing Word content.");
}
}
In this example, the DocumentProcessor class outlines the template method processDocument(), which dictates the steps to open, parse, and close a document. The openDocument() and parseContent() methods are abstract, meaning that each subclass (PDF and Word) must provide its own implementation. Meanwhile, the closeDocument() method is a hook that can optionally be overridden.
The primary reason for using the Template Method Pattern is to enforce a structure across related objects while allowing flexibility in specific implementations. Without this pattern, subclasses would have to replicate code for common steps, leading to unnecessary duplication and higher maintenance costs.
3. Best Practices for Implementing the Template Method Pattern
While the Template Method Pattern is powerful, it’s essential to follow some best practices to maximize its benefits.
Keep the Template Method Final
The template method should be marked as final to prevent subclasses from overriding it and breaking the algorithm’s structure. This enforces a consistent flow of operations across all subclasses.
Use Hook Methods for Customization
If you anticipate that some steps might not always be needed, consider defining them as optional (hook methods). Subclasses can choose to override them or use the default implementation.
Keep Subclass Responsibilities Minimal
Subclasses should only focus on implementing the specific steps they need to customize. Avoid placing too much logic in subclasses, as this can lead to increased complexity and make the code harder to maintain.
4. Common Issues with the Template Method Pattern
Although the Template Method Pattern offers many advantages, there are also potential pitfalls if not used correctly.
Over-Engineering
A common issue developers face with the Template Method Pattern is over-engineering. Avoid adding too many abstract or hook methods, as it could make the base class overly complicated and difficult to maintain.
Violation of the Liskov Substitution Principle
Be cautious when designing subclasses. If a subclass changes the fundamental behavior of the base class's template method, it can break the Liskov Substitution Principle, which states that objects of a subclass should be able to replace objects of the parent class without altering the correctness of the program.
Difficulties with Testing
Since the Template Method Pattern relies heavily on inheritance, unit testing can become tricky, especially when mocking or stubbing specific steps in the process. It’s important to structure tests carefully to ensure each part of the process is tested individually.
5. Real-World Applications of the Template Method Pattern
Building Frameworks
The Template Method Pattern is often used in frameworks where the skeleton of an operation is defined, but developers are allowed to inject their logic at specific points. For example, Spring’s AbstractApplicationContext uses this pattern to outline the lifecycle of a Spring application, but leaves the initialization of beans to the subclass.
Games Development
In game development, the pattern is used to define the general steps of a game loop (like initializing, updating, and rendering) while allowing different games to provide their unique implementations for these steps.
6. Conclusion
The Template Method Pattern is a highly effective tool for structuring complex workflows and promoting code reuse. By following best practices and avoiding common pitfalls, you can create scalable and maintainable systems that are easy to extend.
Have you encountered challenges implementing the Template Method Pattern? Let us know your questions or experiences in the comments below!
Read more at : Template Method Pattern in Java: Creating a Blueprint for Subclasses
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
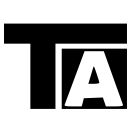
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.