How to Easily Configure CUDA with PyTorch
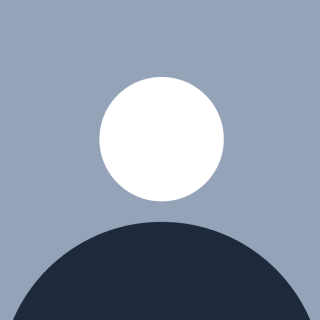

Running machine learning models on cpu could be a big hassle, especial when the model is large or the number of training epochs is high. For this you might be thinking is there any way this process could get faster. One crucial aspect of achieving optimal performance is configuring the CUDA environment properly. In this blog post, we'll delve into the world of CUDA and discuss the best practices for configuring CUDA for PyTorch.
Understanding GPU architecture
Before diving into configuration, it's important to understand CUDA's core components. CUDA (Compute Unified Device Architecture) is a parallel computing platform developed by NVIDIA, enabling developers to leverage GPUs for general-purpose computation.
Key Components:
CUDA Cores: Processing units within the GPU that execute parallel operations.
Memory Bandwidth: The rate at which data transfers between the GPU and system memory.
Clock Speeds: Determines how fast CUDA cores execute instructions.
Different NVIDIA GPUs have varying numbers of CUDA cores, memory bandwidth, and clock speeds. For example:
GPU Model | CUDA Cores | Memory Bandwidth | Clock Speed |
GTX 1080 Ti | 3584 | 768 GB/s | 1.5 GHz |
When choosing a GPU for deep learning applications, it's crucial to consider the performance characteristics of each model.
Setting up CUDA Environment
Now that we've covered the key components of a GPU, let's look at how to set up your environment to start using CUDA with PyTorch. To use PyTorch with CUDA, you'll need to install the necessary drivers and libraries for your operating system (Windows, Linux, or macOS). Here are the steps:
1. Install CUDA Drivers: Download and install the latest CUDA driver version from the NVIDIA website.
https://developer.nvidia.com/cuda-downloads
2. Verify Installation: Run nvcc --version
in the terminal to verify that CUDA is properly installed.
Configuring pytorch CUDA settings
import torch
print(torch.cuda.is_available())
num_gpus = torch.cuda.device_count()
print(num_gpus)
This code will print the number of available GPUs on your system.
The output should be:
Error handling:
If getting KMP duplicate error
Add this line before importing torch:
import os
os.environ["KMP_DUPLICATE_LIB_OK"]="TRUE"
OR
set KMP_DUPLICATE_LIB_OK = TRUE in system environment variables
Optimizing Memory Allocation
Memory allocation is a critical aspect of optimizing CUDA performance. PyTorch manages memory allocation for tensors and modules using a complex algorithm that takes into account various factors such as tensor size, data type, and GPU memory availability.
To optimize memory allocation, you can use the torch.cuda.memory_allocated
to manually manage memory. This allows you to:
Pre-allocate memory: Reserve memory in advance to ensure sufficient space for your tensors.
Reduce tensor size: Minimize tensor sizes to reduce memory usage.
Use mixed precision: Switch between float32 and float64 data types to balance performance and memory efficiency.
Debugging and troubleshooting
When optimizing CUDA configuration for PyTorch, it's essential to debug and troubleshoot common issues. Here are some tips:
Use nvidia-smi: Monitor GPU usage and temperature with the nvidia-smi
command.
Check torch.cuda.get_device_properties(): Verify that your model is being executed on the correct device.
Inspect torch.cuda.memory_allocated(): Verify that memory allocation is working correctly.
Conclusion
Configuring CUDA environment is a critical aspect of achieving optimal performance in deep learning applications. By understanding CUDA architecture, setting up the right drivers and libraries, optimizing memory allocation, utilizing GPU acceleration, and debugging and troubleshooting common issues, you'll be well on your way to unlocking the full potential of your GPUs. Remember, PyTorch provides an extensive API for configuring CUDA settings, so don't hesitate to explore its features!
Subscribe to my newsletter
Read articles from Divij Pirankar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by