How I Made My First GitHub Action Work (With Google’s Help)
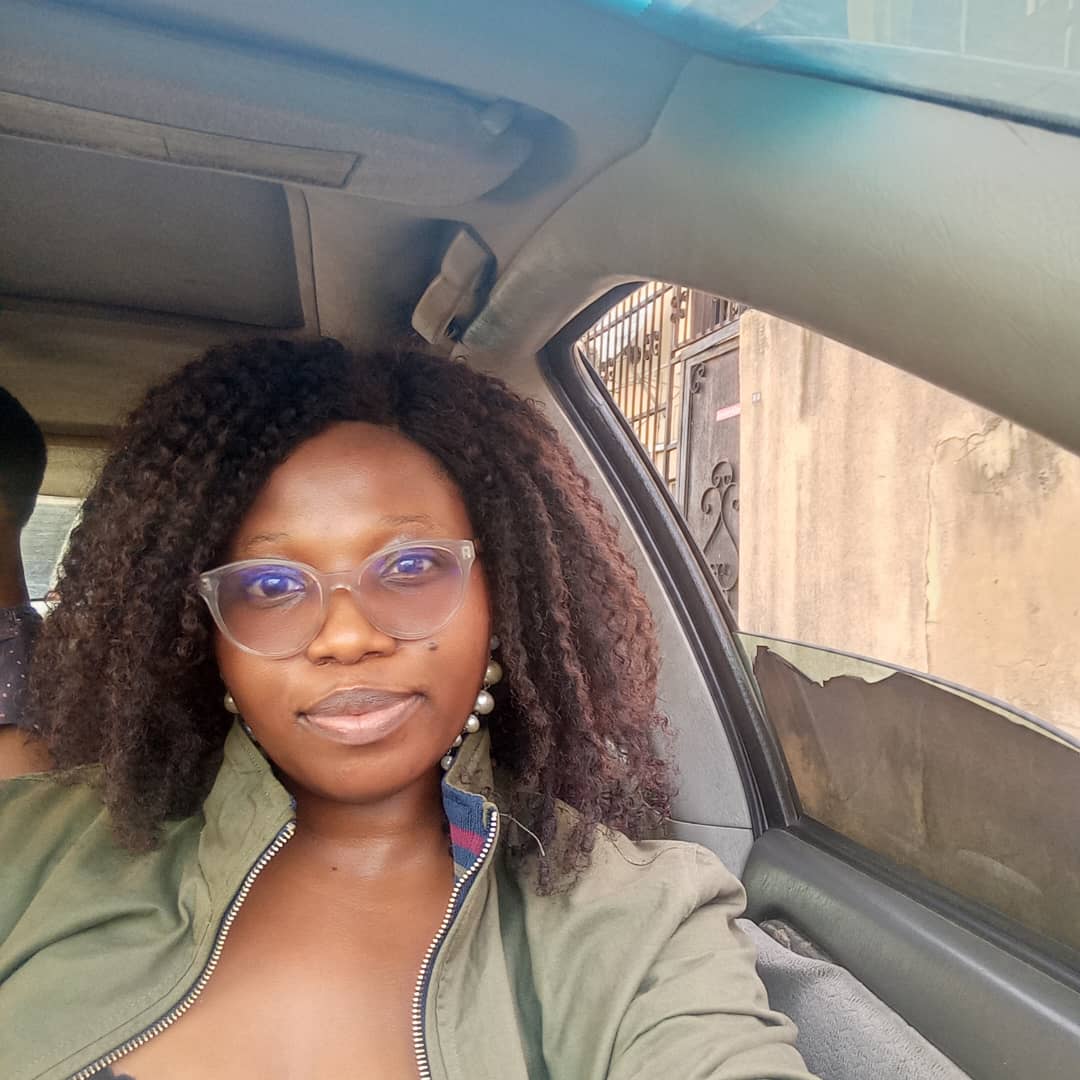

Day 2 of my #90DaysOfBuilding journey
Let me tell you how it actually went the first time I tried setting up a GitHub Action:
Pushed code
Waited anxiously
Saw a scary ❌ error
Googled like my life depended on it
Made it work anyway
The GitHub Actions Promise
GitHub Actions promises to automate your workflows: run tests automatically, deploy your code, and more – all triggered by events in your repository like pushes or pull requests.
It sounds magical... until you try to set it up for the first time.
The Real Story
I was building a community platform prototype (part of my path toward launching a startup), and wanted to automate testing to save time.
The YouTube tutorials made it look so easy:
Create a
.github/workflows
folderAdd a YAML file
Push and you're done!
What they didn't show: my YAML file had more bugs than my app 😅
Common GitHub Actions Errors I Faced
Before we get to the solution, here are some errors that had me pulling my hair out:
Indentation errors
Error: Your workflow file was invalid: The workflow is not valid.
.github/workflows/main.yml (Line: 15, Col: 3): Unexpected value 'with'
Missing required fields
Error: Invalid workflow file: .github/workflows/main.yml#L15
The workflow is not valid. .github/workflows/main.yml (Line: 15, Col: 13):
Required property 'runs-on' not found
Path issues with actions
Error: Could not resolve action, did you mean 'actions/checkout@v2'?
The Breakthrough Solution
After much trial and error, I discovered you only need 3 key parts to get started with a basic CI pipeline for a Node.js project:
name: CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Setup Node
uses: actions/setup-node@v4
with:
node-version: 18
- run: npm install
- run: npm test
Let's break this down:
name: A friendly name for your workflow
on: What triggers this workflow (in this case, any push to the repository)
jobs: The tasks to perform
build: The name of this job
runs-on: The type of machine to run on
steps: The individual actions to take
Check out the code
Set up Node.js
Install dependencies
Run tests
What Actually Helped Me Succeed
After struggling with the syntax, here's what worked for me:
1. Reading Other Developers' YAML Files
GitHub is full of public repositories with working Actions. I found projects similar to mine and analyzed their workflow files.
For example, here's the workflow from a popular Node.js project:
name: Tests
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
test:
runs-on: ubuntu-latest
strategy:
matrix:
node-version: [14.x, 16.x, 18.x]
steps:
- uses: actions/checkout@v3
- name: Use Node.js ${{ matrix.node-version }}
uses: actions/setup-node@v3
with:
node-version: ${{ matrix.node-version }}
cache: 'npm'
- run: npm ci
- run: npm test
This taught me about the matrix strategy for testing across multiple Node versions!
2. Googling Every Line I Didn't Understand
The GitHub Actions documentation is comprehensive, but sometimes overwhelming. I found it more helpful to:
Search for specific error messages
Look up individual actions (like
actions/checkout@v3
)Find beginner-friendly blog posts with examples
3. Testing on a Small Toy Repo First
Instead of trying to set up CI/CD for my main project right away, I created a tiny test repository with:
A simple Node.js app
A basic test suite
A GitHub Actions workflow
This allowed me to experiment without breaking anything important.
Beyond the Basics: What I Added Next
Once I had the basic CI working, I gradually added more features:
1. Environment Variables
jobs:
build:
runs-on: ubuntu-latest
env:
NODE_ENV: test
DB_CONNECTION: mock
steps:
# ... steps here
2. Caching Dependencies
- uses: actions/checkout@v3
- name: Setup Node
uses: actions/setup-node@v4
with:
node-version: 18
cache: 'npm'
- run: npm install
3. Conditional Steps
- name: Deploy to Production
if: github.ref == 'refs/heads/main'
run: npm run deploy
The Impact on My Builder Journey
Now, every time I push code, tests run automatically. This small automation saves me hours each week that I can use to learn more and build faster.
No more "oops I forgot to test." No more "it works on my machine."
Just clean, repeatable CI magic that makes my projects more professional as I work toward building my first startup.
Common Pitfalls to Avoid
YAML indentation: Spaces matter! YAML is very strict about indentation.
Forgetting uses: When referencing external actions, you need the
uses
keyword.Path issues: Make sure your test scripts match what's in your package.json.
Environment secrets: Don't hardcode API keys; use GitHub Secrets instead.
Practical Example: Complete Workflow for a Node.js API
Here's a more complete example of a workflow I use for a Node.js API project:
name: Node.js API CI
on:
push:
branches: [ main, develop ]
pull_request:
branches: [ main, develop ]
jobs:
test:
runs-on: ubuntu-latest
services:
# Mock database for testing
mongodb:
image: mongo:4.4
ports:
- 27017:27017
steps:
- uses: actions/checkout@v3
- name: Use Node.js
uses: actions/setup-node@v4
with:
node-version: '18'
cache: 'npm'
- name: Install dependencies
run: npm ci
- name: Lint code
run: npm run lint
- name: Run tests
run: npm test
env:
NODE_ENV: test
MONGODB_URI: mongodb://localhost:27017/test-db
- name: Build
run: npm run build
- name: Upload coverage reports
uses: codecov/codecov-action@v3
with:
token: ${{ secrets.CODECOV_TOKEN }}
To My Fellow Builders
If you're curious about GitHub Actions, don't wait to feel ready. Break something small, Google a lot, and build from there.
You'll get it. And when you do, share what you've learned.
That's how we all rise together. 🚀
Resources That Helped Me
Shield.io Badges - Add those cool status badges to your README
What's Next?
I'm planning to launch a weekly coding session soon where we'll go through DevOps basics for beginners. If you found this helpful, follow me here or connect on Twitter/LinkedIn to stay updated!
What was your first GitHub Actions experience like? Let me know in the comments!
Happy automating! 🤖
#githubactions #github #programming #beginners #automation #devops #developer #webdev
Subscribe to my newsletter
Read articles from Abigeal Afolabi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
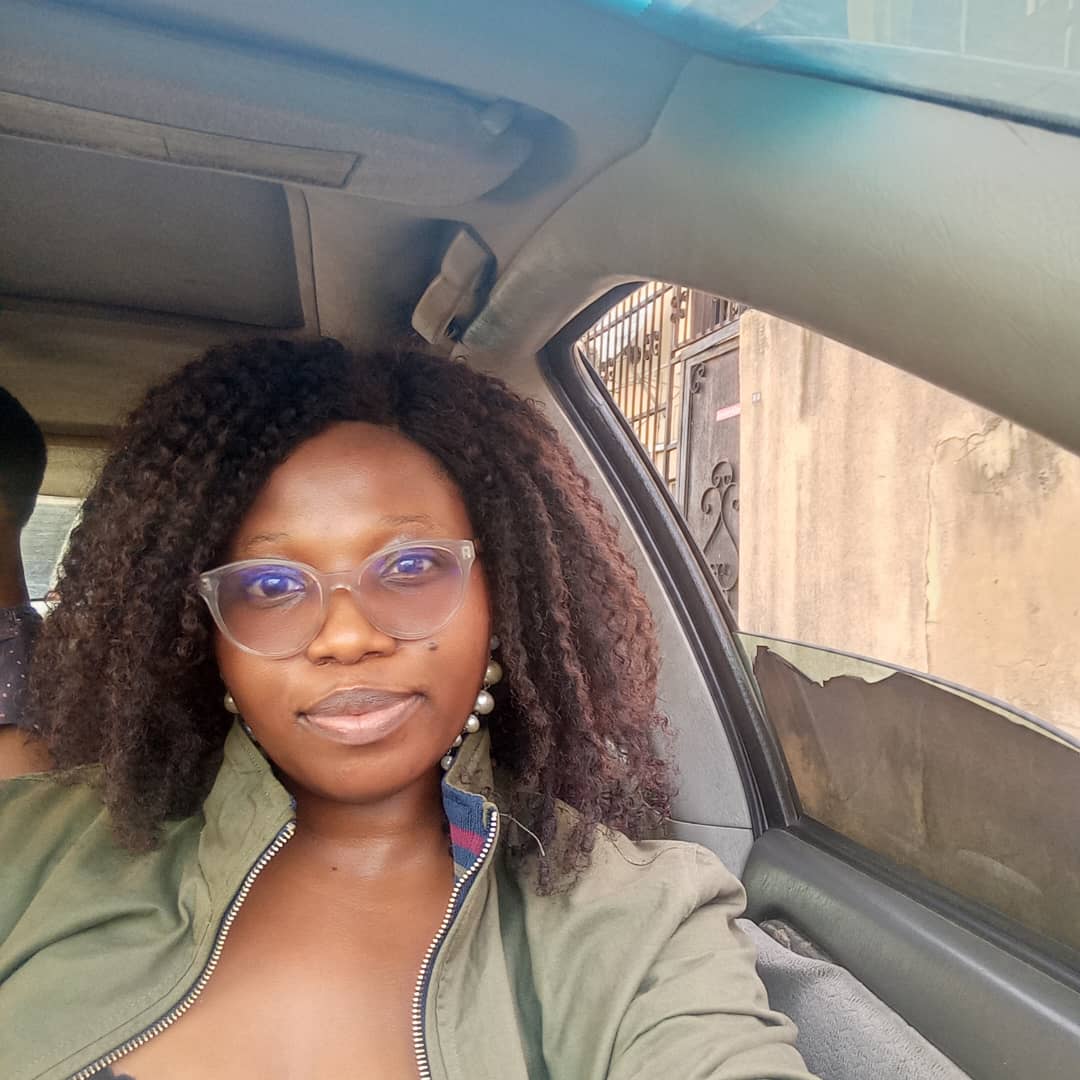
Abigeal Afolabi
Abigeal Afolabi
🚀 Software Engineer by day, SRE magician by night! ✨ Tech enthusiast with an insatiable curiosity for data. 📝 Harvard CS50 Undergrad igniting my passion for code. Currently delving into the MERN stack – because who doesn't love crafting seamless experiences from front to back? Join me on this exhilarating journey of embracing technology, penning insightful tech chronicles, and unraveling the mysteries of data! 🔍🔧 Let's build, let's write, let's explore – all aboard the tech express! 🚂🌟 #CodeAndCuriosity