Bridge Pattern in Java for Separating Abstraction from Implementation
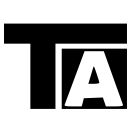
5 min read
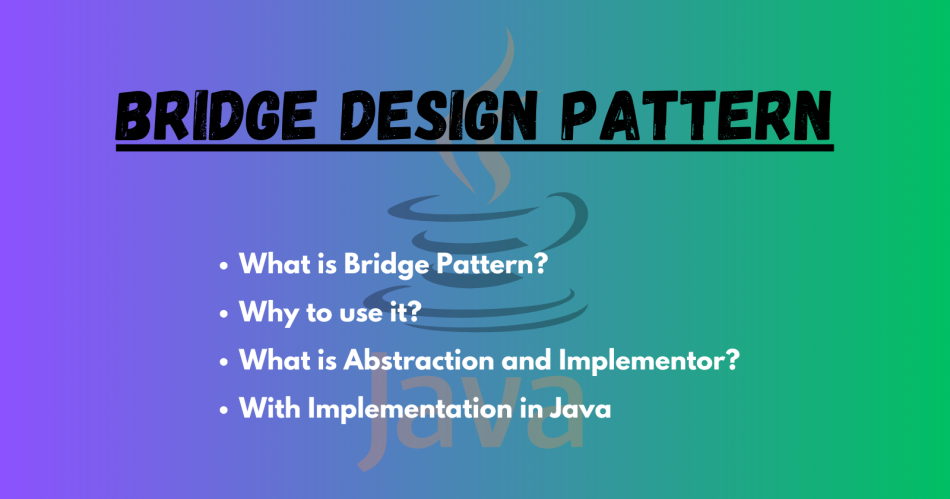
1. Introduction to the Bridge Pattern
1.1 What is the Bridge Pattern?
The Bridge Pattern is a structural design pattern that divides abstraction from implementation so that they can vary independently. In simpler terms, it allows you to create different versions of a class and its behavior, which is particularly useful when you need to switch between multiple implementations or extend functionality without modifying existing code.
1.2 Why Choose the Bridge Pattern?
Imagine you’re building a drawing application that needs to support different types of shapes (like circles, rectangles, etc.) and various drawing APIs. You don’t want each shape to depend on a specific API implementation directly. Using the Bridge Pattern helps you decouple these concerns, which enhances the code’s flexibility and maintainability.
Without this pattern, creating separate classes for each shape and drawing API combination can lead to class explosion, where an overwhelming number of classes are needed to handle every scenario. The Bridge Pattern solves this by providing a clear separation of abstraction and implementation.
2. Implementing the Bridge Pattern in Java
2.1 Key Components of the Bridge Pattern
In the Bridge Pattern, two major components are involved:
- Abstraction: Defines a high-level control mechanism or class.
- Implementor: Specifies the interface for implementation classes. The implementation classes (concrete implementors) carry out the actual operation specifics.
Each side of the Bridge Pattern in Java can be represented by either abstract classes or interfaces.
// Implementor interface - specifies the interface for drawing APIs
interface DrawingAPI {
void drawCircle(double x, double y, double radius);
}
// Concrete Implementors - Different drawing implementations
class DrawingAPI1 implements DrawingAPI {
public void drawCircle(double x, double y, double radius) {
System.out.println("Drawing API 1: circle at (" + x + ", " + y + ") with radius " + radius);
}
}
class DrawingAPI2 implements DrawingAPI {
public void drawCircle(double x, double y, double radius) {
System.out.println("Drawing API 2: circle at (" + x + ", " + y + ") with radius " + radius);
}
}
2.2 Creating the Abstraction
The Abstraction class will hold a reference to the implementation class and pass requests to it. This decouples the functionality of shapes from their rendering implementations.
// Abstract class that uses an instance of the implementor
abstract class Shape {
protected DrawingAPI drawingAPI;
protected Shape(DrawingAPI drawingAPI) {
this.drawingAPI = drawingAPI;
}
public abstract void draw(); // High-level control mechanism
public abstract void resizeByPercentage(double pct); // Example operation for shapes
}
// Concrete Abstraction
class CircleShape extends Shape {
private double x, y, radius;
public CircleShape(double x, double y, double radius, DrawingAPI drawingAPI) {
super(drawingAPI);
this.x = x;
this.y = y;
this.radius = radius;
}
// Forward request to implementor
public void draw() {
drawingAPI.drawCircle(x, y, radius);
}
public void resizeByPercentage(double pct) {
radius *= (1.0 + pct / 100.0);
}
}
By this structure, CircleShape is free to change the implementation it uses at runtime. You could dynamically decide to use DrawingAPI1 or DrawingAPI2 based on your needs.
2.3 Using the Bridge Pattern
Here’s how you can instantiate different CircleShape objects with different drawing APIs:
public class BridgePatternDemo {
public static void main(String[] args) {
Shape circle1 = new CircleShape(1, 2, 3, new DrawingAPI1());
Shape circle2 = new CircleShape(5, 7, 11, new DrawingAPI2());
circle1.draw();
circle2.draw();
circle1.resizeByPercentage(10);
circle1.draw();
}
}
3. Best Practices for Using the Bridge Pattern
Choose the Bridge Pattern for the Right Reasons
The Bridge Pattern is powerful, but it’s not always necessary. Consider it for situations where you have a high number of combinations of abstractions and implementations. This pattern is also valuable in cases where both sides (abstraction and implementation) might change over time.
Avoid Unnecessary Complexity
Overusing the Bridge Pattern can make your code overly complex. If you have simple cases with only one or two implementations, the added complexity might outweigh the benefits. Always weigh the complexity of the pattern against the flexibility it provides.
Design with Extension in Mind
When designing with the Bridge Pattern, always think about future extensions. For example, if you plan to add more drawing APIs or shapes in the future, ensure that your current implementation can easily accommodate these changes.
4. Potential Issues and Troubleshooting
Misplacing Responsibilities
One common issue with the Bridge Pattern is not clearly distinguishing between the Abstraction and Implementor classes. This can lead to tightly coupled code, which defeats the purpose of using this pattern. Ensure that abstraction classes are focused on high-level operations, while implementation classes handle specifics.
Refactoring Legacy Code
Incorporating the Bridge Pattern into existing code that doesn’t separate abstraction from implementation can be challenging. It may involve significant refactoring, especially if there is a lot of dependence on a specific implementation. Consider breaking down the refactor into stages and thoroughly test each stage to avoid regressions.
5. Conclusion
The Bridge Pattern is a valuable tool for separating abstraction from implementation, making Java code more flexible and maintainable. By carefully considering when to use this pattern and following best practices, you can avoid common pitfalls and create scalable, clean code.
Feel free to leave a comment below if you have questions about using the Bridge Pattern or need help with your Java code!
Read more at : Bridge Pattern in Java for Separating Abstraction from Implementation
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
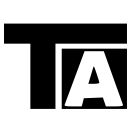
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.