Nomba React SDK: A Quick Setup Guide
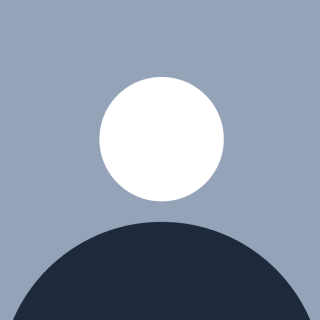

If you're building a React-based application and want to integrate seamless payments, Nomba offers a powerful SDK to help you do just that—without writing low-level payment logic. In this quick guide, you'll learn how to set up and use the Nomba React SDK to accept payments in a few simple steps.
Prerequisites
Before jumping into the code, make sure you have the following ready:
- A Nomba Business Account
- Your
accountId
andclientId
from the Nomba dashboard - A React project set up with Vite or Create React App
Installing the SDK
npm install react-nomba-checkout-sdk
# or
yarn add react-nomba-checkout-sdk
Once installed, you’ll need to initialize the SDK at the top level of your app. This ensures Nomba’s checkout modal and required scripts are ready when you need them.
import { StrictMode } from 'react'
import { createRoot } from 'react-dom/client'
import './index.css'
import App from './App.tsx'
import { InitializeNombaCheckout} from 'react-nomba-checkout-sdk';
InitializeNombaCheckout();
createRoot(document.getElementById('root')!).render(
<StrictMode>
<App />
</StrictMode>,
)
Here’s a minimal example of how to trigger a payment using the Nomba SDK
Basic Setup with Nomba SDK
import { useState } from "react";
import { useNombaCheckout } from 'react-nomba-checkout-sdk';
const Pay = () => {
const [amount, setAmount] = useState('');
const [isLoading, setIsLoading] = useState(false);
const handleCheckout = async () => {
if (!amount) return alert('Please enter an amount.');
setIsLoading(true);
await useNombaCheckout({
accountId: import.meta.env.VITE_ACCOUNT_ID,
clientId: import.meta.env.VITE_CLIENT_ID,
order: {
orderReference: `order-${Math.floor(Math.random() * 1000000)}`,
callbackUrl: '<callbackURL>',
customerEmail: 'jane@example.com',
customerId: 'user-123',
amount: parseFloat(amount).toFixed(2),
currency: 'NGN',
accountId: import.meta.env.VITE_ACCOUNT_ID,
splitRequest: {
splitType: 'PERCENTAGE',
splitList: [
{
accountId: 'your-account-id',
value: '65.45',
},
],
},
tokenizeCard: false,
onCreateOrder: (ref) => {
console.log('Order created:', ref);
setIsLoading(false);
},
onFailure: (err) => {
console.error('Checkout failed:', err);
setIsLoading(false);
},
onClose: () => {
console.log('Checkout modal closed');
setIsLoading(false);
},
onPaymentSuccess: (res) => {
console.log('Payment successful:', res);
},
});
};
return (
<div>
<h2>Pay with Nomba</h2>
<input
type="text"
placeholder="Enter amount (e.g., 3000)"
value={amount}
onChange={(e) => setAmount(e.target.value)}
/>
<button onClick={handleCheckout} disabled={isLoading}>
{isLoading ? 'Processing...' : 'Pay Now'}
</button>
</div>
);
};
export default Pay;
Tip: Always use environment variables (VITE_ACCOUNT_ID
, etc) to keep your credentials secure.
Making Sense of Nomba SDK Callbacks
One of the most powerful features of the Nomba SDK is its callbacks, which help you stay in control of the user's journey. Here’s a breakdown of what each callback does—and how to use them effectively:
onCreateOrder
: When Things Kick Off
This callback is triggered right after an order reference is created—before the actual payment begins.
onCreateOrder: (orderRef) => {
console.log('Order created:', orderRef);
}
onFailure
: When Something Goes Wrong
If the checkout fails (maybe due to network issues, invalid input, or user cancellation before payment begins), this callback gets triggered. You render an appropriate message to notify the user about what happened.
onFailure: (error) => {
console.error('Checkout failed:', error);
}
onClose
: When the User Walks Away
Sometimes users just close the payment modal. Maybe they had second thoughts or got distracted. This callback handles that. You can clear modals or overlays, restore the UI to it's pre-payment state.
onClose: () => {
console.log('User closed the checkout modal.');
}
onPaymentSuccess
: When the Payment Goes Through
This is the one we all love—the payment was completed, and money is on the move! This callback fires when the transaction is successful.
onPaymentSuccess: (response) => {
console.log('Payment successful:', response);
}
conclusion
The Nomba React SDK offers a fast and reliable way to accept payments without worrying about complex APIs. Once you're familiar with the basic setup, you can explore advanced features like card tokenization or webhook validation.
Subscribe to my newsletter
Read articles from Makanju Oluwafemi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Makanju Oluwafemi
Makanju Oluwafemi
My name is Makanju Oluwafemi, and I'm passionate about the dynamic relationship between developers and API tools. As a DevRel and Frontend Engineer, I’m dedicated to building a strong, engaged community and contributing to the growth of the African tech ecosystem. I aim to leverage my skills to drive innovation, share knowledge, and support the development of technology across the continent.