Secrets to Extending Class Functionality Without Modification Using the Visitor Pattern in Java
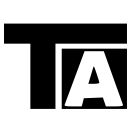
5 min read
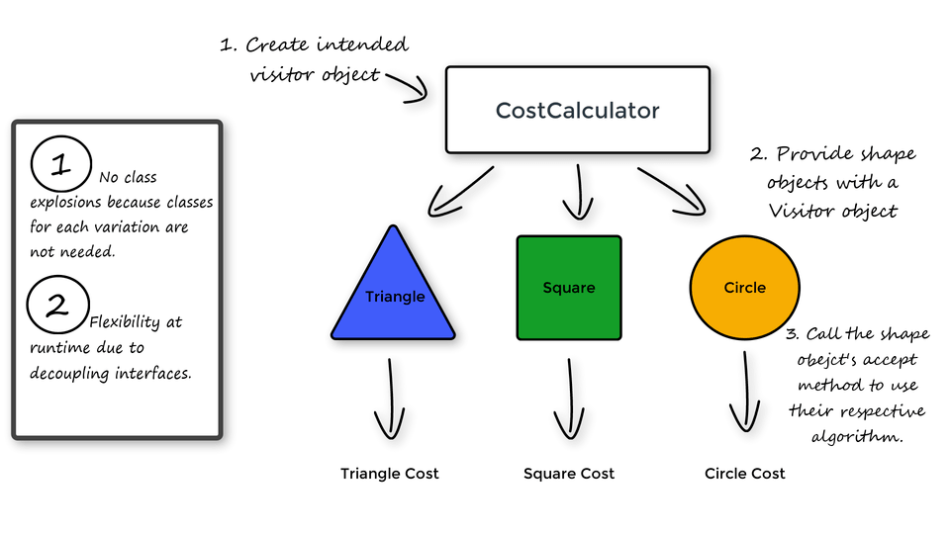
Source: Secrets to Extending Class Functionality Without Modification Using the Visitor Pattern in Java
1. Introduction: What is the Visitor Pattern?
1.1 Overview of the Visitor Pattern
Introduce the Visitor Pattern as one of the Behavioral Design Patterns. Explain that it allows adding new functionality to a set of classes without modifying their structure, promoting code that is closed for modification but open for extension.
Highlight that the Visitor Pattern adheres to the Open/Closed Principle (OCP) in SOLID, making systems more maintainable and extensible. For example, when dealing with a Shape object, instead of adding a new method to Shape, we create a Visitor that can perform a new operation, such as drawing or calculating the area, without altering Shape.
1.2 Real-World Analogy
Use the analogy of a museum guide. Artworks (Elements) remain unchanged, while the guide (Visitor) can provide various types of information about each piece, like historical background, style, or artist details, without modifying the artworks themselves.
This comparison shows how the Visitor Pattern adds functionality similarly, by allowing different operations to be performed on an object without modifying the object’s structure.
1.3 Purpose and Benefits
- Core Purpose: Explain why you would want to use the Visitor Pattern: it enables the addition of new behaviors to existing classes without altering their internal structure. This pattern is particularly useful when the set of operations on an object structure is subject to change more often than the object structure itself.
- Detailed Benefits: List specific benefits like maintaining stability in existing code, extending functionality without modifying the original class, and ease of use when dealing with multiple types of objects and varied operations. Additionally, it helps separate operations from the objects on which they operate, increasing modularity and clarity.
2. Implementing the Visitor Pattern in Java
2.1 Core Components of the Visitor Pattern
Key Components: Explain the four main components of the Visitor Pattern:
- Visitor Interface: Declares methods for visiting each type of Element (e.g., visit(Circle
- ConcreteVisitor: Implements the Visitor interface, defining the operations that will be performed on each Element.
- Element Interface: Declares the accept method, which takes a Visitor object as an argument.
- ConcreteElement: Implements the Element interface and calls the accept method on the Visitor, passing itself as an argument.
2.2 Setting Up the Example Code
Create a Basic Structure: Start with an example to illustrate the Visitor Pattern in action. Introduce a Shape class as an Element interface, with Circle and Rectangle as concrete implementations. Each class implements an accept method to accept a Visitor.
interface Shape {
void accept(Visitor visitor);
}
class Circle implements Shape {
public void accept(Visitor visitor) {
visitor.visit(this);
}
}
class Rectangle implements Shape {
public void accept(Visitor visitor) {
visitor.visit(this);
}
}
2.3 Implementing the Visitor Interface
Define Visitor Interface: Add a Visitor interface that contains a visit method for each Element type. Each visit method takes an Element parameter. This allows the Visitor to perform different operations on each Element.
interface Visitor {
void visit(Circle circle);
void visit(Rectangle rectangle);
}
2.4 ConcreteVisitor Implementations
Examples of Visitors: Create specific Visitor implementations, such as DrawVisitor to render shapes, and AreaCalculatorVisitor to calculate areas. Show each Visitor performing its operation on the Circle and Rectangle elements.
class DrawVisitor implements Visitor {
public void visit(Circle circle) {
System.out.println("Drawing a circle");
}
public void visit(Rectangle rectangle) {
System.out.println("Drawing a rectangle");
}
}
class AreaCalculatorVisitor implements Visitor {
public void visit(Circle circle) {
System.out.println("Calculating area of the circle");
}
public void visit(Rectangle rectangle) {
System.out.println("Calculating area of the rectangle");
}
}
3. Best Practices for Using the Visitor Pattern
When to Use the Visitor Pattern
Discuss when it’s best to use the Visitor Pattern, like when a system contains a group of objects with a stable structure but dynamic operations. It’s especially useful if the operations on the object structure frequently change.
Avoiding Common Pitfalls
Acknowledge the added complexity with the Visitor Pattern, which can be overkill for simpler tasks. Recommend it mainly for scenarios where the benefit of extensibility outweighs this complexity.
Extending Visitor Pattern Capabilities
Offer insights on extending the pattern, like adding more Visitors for different operations or modifying the Visitor interface to accommodate new Element types.
4. Advanced Topics and Related Issues
4.1 Double Dispatch and the Visitor Pattern
Provide a thorough look at how the Visitor Pattern enables double dispatch in Java, allowing a method to act on different object types based on runtime information.
4.2 Visitor Pattern vs. Other Patterns
Compare the Visitor Pattern with similar patterns, such as Strategy and Composite, explaining how they differ in purpose and usage, and why the Visitor Pattern might be the best choice in certain cases.
5. Conclusion
Summarize the main points discussed and emphasize the value of using the Visitor Pattern to add functionality to a class structure without modifying it. Encourage readers to comment with any questions or share their experiences.
Read more at : Secrets to Extending Class Functionality Without Modification Using the Visitor Pattern in Java
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
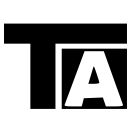
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.