A Developer's Guide to Scalable API Requests with Params in JavaScript and TypeScript
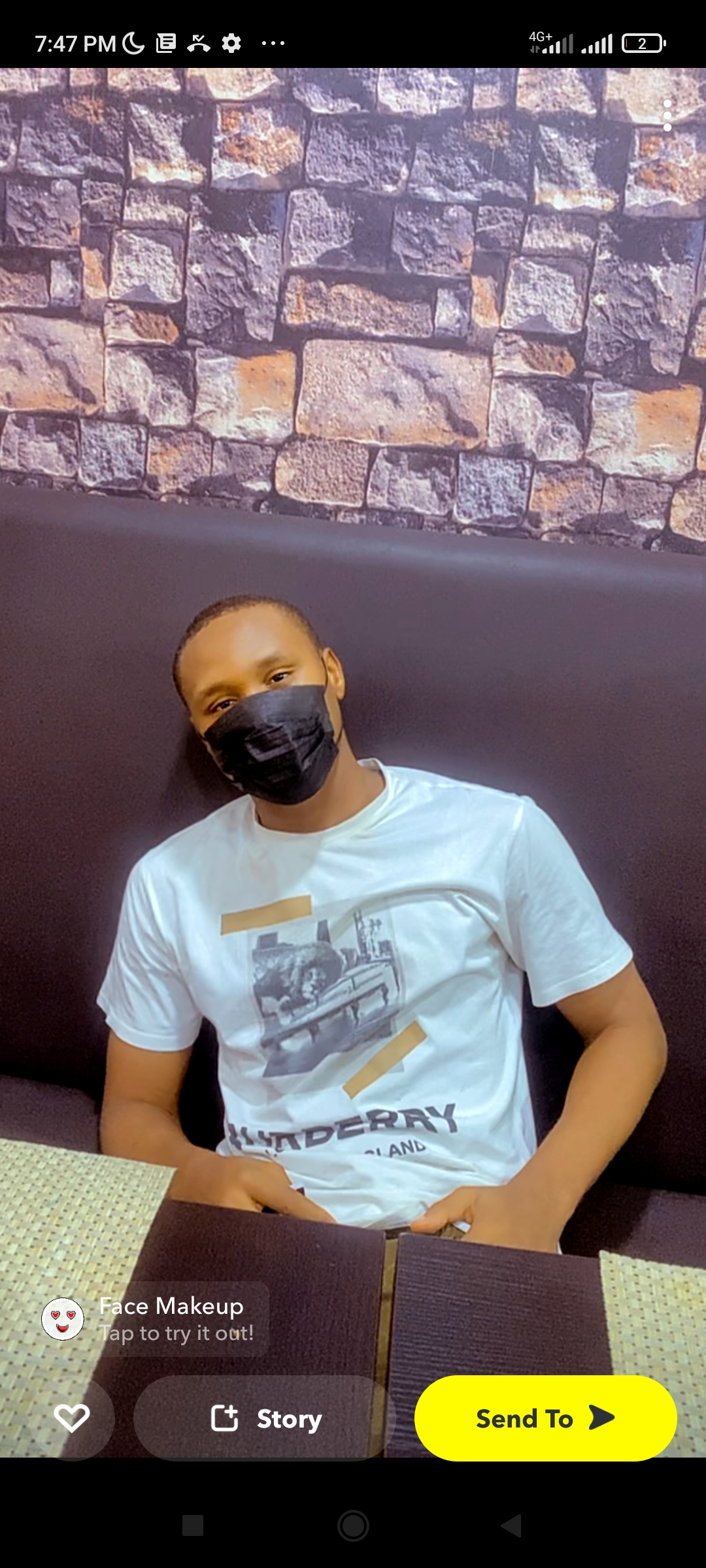

Welcome back to my article. Today, we will be discussing how we can make a scalable and dynamic API call using parameters.
In the world of software engineering, it’s likely for every developer to make an API call, whether a POST or a GET API request, so it is very important for developers to understand how to make this call dynamically.
However, to make an API call, we need to organise how our data should be received and sent. To do this, we need a parameter for dynamic API calls, a schema to store data in our database, and an interface to keep our data organized. First, let's look at how we can make an API call in JavaScript and TypeScript.
API REQUEST
Assuming we want to make an API call from the Unsplash URL, we would need an API key to access the data from Unsplash. Once we have an API key, we can make this API call. Here’s a quick way to make this call using JavaScript and TypeScript.
// Create a global variable for our API key env file, which we will be using to access unsplash endpoint in our code
const Api_key = process.env.API_KEY ;
const url = 'https://unsplash_api';
async function fetchApi(url, method = 'GET', body = null, headers = {}) {
const defaultHeaders = {
'Content-type': 'application/json',
'Authorization': `Bearer ${Api_Key}`,
...headers
};
const response = await fetch(url, {
method,
headers: defaultHeaders,
body: body ? JSON.stringify(body) : null
});
let data;
try {
data = await response.json();
if (!response.ok) {
return 'Data failed to retrieve';
}
} catch (error) {
console.error('API call failed to fetch data', error);
return 'Error trying to fetch data';
}
return data;
}
In TypeScript, this is how we make the same call.
const api_key = 'unspalsh00992';
async function fetchapi(url: string, method: string = 'GET', body: any = null, header: Record<string, string> = {}): Promise<string> {
// Try to get the response
try {
// Create a default header to dynamically access headers in the function
const defaultHeader = {
'Content-type': 'application/json',
'Authorization': `Bearer ${process.env.api_key}`,
...header
};
// Create a variable that defines our response data
const response = await fetch(url, {
method,
headers: defaultHeader,
body: body ? JSON.stringify(body) : null
});
// Create a variable to hold our response
let data = await response.json();
return data;
} catch (error) {
console.error('API call failed', error);
return 'An error occurred while fetching data';
}
}
Now that we have finally learned how to make our first API call, let's look at the issue at hand. Imagine we want to make an API call for specific images of a certain kind of location, e.g., "beach in Spain." When we make the api call like the previous one, it wont give us full control of the kind of data we want to use, and it isn't scalable or ideal to allow our API to keep fetching irrelevant data. This is where parameters come into play.
What is a Parameter
A parameter is what we feed into our API call to get a specific kind of data. This is useful when we don't want to get irrelevant data. Here is a detailed example of how we can make an API call using a parameter.
Firstly, we need to set our parameter dynamically, and to do this, we can use a plain Object-based parameter structure. This can be done directly in JavaScript or TypeScript. While libraries like Zod can assist with table validation, this article focuses on using pure JavaScript and TypeScript.
async function fetchApi(
url: string,
params: { country: string; type: string; location: string },
method: string = 'GET',
body: any = null,
headers: Record<string, string>
): Promise<string> {
try {
const { country, type, location } = params;
const unsplashParam = `${country}, ${location}, ${type}`;
const apiUrl = `https://unsplashapi/search/photos?query=${encodeURIComponent(unsplashParam)}&per_page=40`;
const defaultHeaders = {
'Content-Type': 'application/json',
Authorization: `Bearer ${process.env.API_KEY}`,
...headers
};
const response = await fetch(apiUrl, {
method,
headers: defaultHeaders,
body: body ? JSON.stringify(body) : null
});
const data = await response.json();
return data;
} catch (error) {
console.error('Failed to retrieve API response', error);
return 'API retrieval failed';
}
}
Then we can dynamically call our function this way.
await fetchApi('https://unsplashapi...',{ type: 'beach', country: 'Spain', location: 'Ibiza' });
await fetchApi('https://usplashapi',{ type: 'temple', country: 'India', location: 'Dubai' });
await fetchApi('https://unspalashapi',{ type: 'temple', country: 'Nigeria', location: 'Akure' });
You can call multiple APIs dynamically without hardcoding values. This approach offers a more scalable and efficient way to make API requests to any endpoint of your choice.
Now that we know how to make an API call dynamically, we need to decide if we want to save data or send it directly to our client, if we don't want to set up a backend database for this.
My advice is to create a database backend system to have more control over how we use our data.
What is a database
A database is a backend system where useful data can be stored. Think of it as the brain of an application, where the key information ,logic and useful data are saved and used throughout the application. To organise data effectively , we use what we call a schema
In JavaScript development, PostgreSQL is highly recommended. Although PostgreSQL isn't written in JavaScript, we can interact with it using JavaScript libraries like pg or Prisma.
What is a schema?
A schema is a structure or blueprint that helps us organize data in a database or system. Think of it as a data organizer. For instance, imagine we don't have a schema and we want to make an API call. Without a schema, our server wouldn't be able to recognize what data the user is asking for. But with a schema, the system understands what kind of data we are dealing with.
This article does not focus on saving dynamic API calls data. To learn more about SQL databases and how to store data using sql , I will cover this topic in my next article.
Thank you for taking the time to read this. I hope it helps you understand how you can effectively use an API parameters, when making an api call to get a specific kind data.
Until the next article!
Subscribe to my newsletter
Read articles from Abanobi Emmanuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
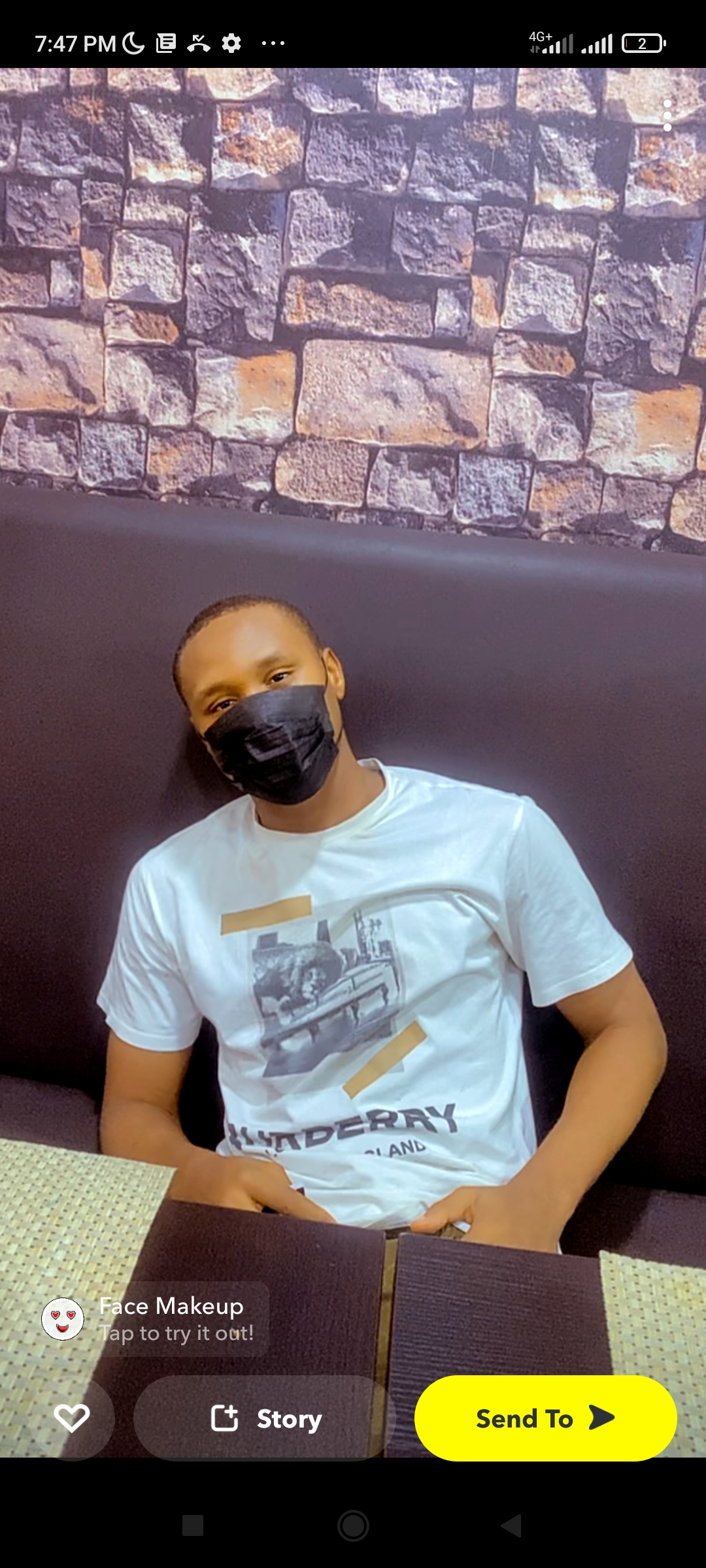
Abanobi Emmanuel
Abanobi Emmanuel
I am a skilled software engineer and technical writer with extensive experience in the field of computer science. With a passion for technology and a drive to stay up-to-date with the latest trend.