DSA Count Digits Solution in java and Python
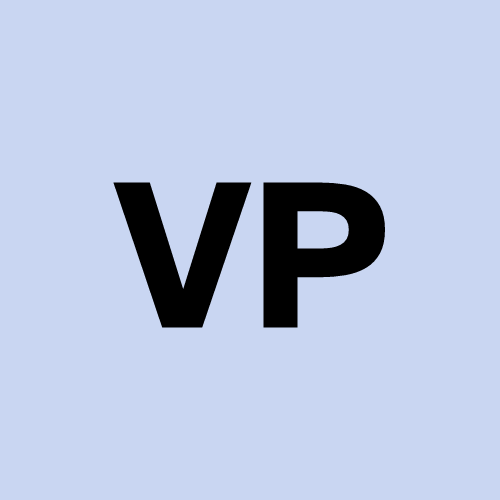
Count Digits in a Number โ Code, Explanation & Real-World Use Case
๐ Introduction
In this blog post, I'll walk you through how I solved the problem of counting digits in a number. This can help developers looking to understand different approaches (recursive and iterative) to solve this fundamental programming problem and see how it translates between Java and Python.
๐ง Problem Statement / Objective
Create a program that counts the number of digits in a given integer, including handling edge cases like zero and negative numbers. Implement solutions using both recursion and iteration, in both Java and Python.
๐งฉ Tools & Technologies Used
Language: Java, Python
IDE: IntelliJ, VSCode
Concepts: Recursion, Iteration, Edge Case Handling
๐ป Java Code
package maths;
public class CountDigits {
private static int solution(long inputNo) {
if(inputNo == 0) return 1;
return countDigitsByRecursion(Math.abs(inputNo));
}
private static int countDigitsByRecursion(long inputNo) {
if(inputNo == 0) return 0;
return 1 + countDigitsByRecursion(inputNo/10);
}
private static int countDigitsByIteration(long inputNo) {
if(inputNo == 0) return 1;
int count = 0;
inputNo = Math.abs(inputNo);
while(inputNo != 0) {
inputNo /= 10;
++count;
}
return count;
}
public static void main(String[] args) {
// Test cases for solution() method (recursion)
long input1 = 0;
if (1 == solution(input1))
System.out.println("Test case 1 (solution) passed");
long input2 = 12345;
if (5 == solution(input2))
System.out.println("Test case 2 (solution) passed");
long input3 = -430;
if (3 == solution(input3))
System.out.println("Test case 3 (solution) passed");
// Test cases for iteration method
long input4 = 0;
if (1 == countDigitsByIteration(input4))
System.out.println("Test case 4 (iteration) passed");
long input5 = 789;
if (3 == countDigitsByIteration(input5))
System.out.println("Test case 5 (iteration) passed");
long input6 = -10000;
if (5 == countDigitsByIteration(input6))
System.out.println("Test case 6 (iteration) passed");
}
}
๐ป Python Code
def count_digits_recursion(number):
number = abs(number)
if number == 0:
return 0
return 1 + count_digits_recursion(number // 10)
def count_digits_iteration(number):
if number == 0:
return 1
count = 0
number = abs(number)
while number != 0:
number = number // 10
count += 1
return count
def solution(number):
if number == 0:
return 1
return count_digits_recursion(number)
# Test cases
if __name__ == "__main__":
# Test recursion solution
assert solution(0) == 1, "Test case 1 (solution) failed"
assert solution(12345) == 5, "Test case 2 (solution) failed"
assert solution(-430) == 3, "Test case 3 (solution) failed"
# Test iteration method
assert count_digits_iteration(0) == 1, "Test case 4 (iteration) failed"
assert count_digits_iteration(789) == 3, "Test case 5 (iteration) failed"
assert count_digits_iteration(-10000) == 5, "Test case 6 (iteration) failed"
print("All test cases passed!")
๐ Explanation
Java Implementation:
solution(): The main method that handles the edge case of 0 (which has 1 digit) and delegates to the recursive method.
countDigitsByRecursion(): Recursively divides the number by 10 until it becomes 0, counting each division.
countDigitsByIteration(): Uses a while loop to count digits by repeatedly dividing the number by 10.
main(): Contains test cases verifying both approaches work correctly, including handling negative numbers.
Python Implementation:
count_digits_recursion(): Similar recursive approach as Java, using integer division (//).
count_digits_iteration(): Iterative approach with while loop.
solution(): Same edge case handling as Java version.
Test cases: Uses assertions to verify correctness.
Key Differences:
Python uses
//
for integer division while Java uses/
Python has simpler syntax without type declarations
Java requires class and method definitions
Python uses assertions for testing while Java uses print statements
๐ ๏ธ Output / Result
For both implementations, running the code will show that all test cases pass, demonstrating correct handling of:
Zero (returns 1)
Positive numbers
Negative numbers
Both recursive and iterative approaches
๐ Real-World Use Case / Application
Counting digits is fundamental in:
Data Validation: Ensuring phone numbers or IDs have correct length
Banking Systems: Validating credit card numbers or account numbers
Data Processing: Analyzing number distributions in datasets
Cryptography: Working with large prime numbers
Competitive Programming: Common basic problem in coding challenges
๐งต Conclusion
Through this exercise, I learned:
How to approach a problem with both recursive and iterative solutions
The importance of handling edge cases (like zero)
How similar logic translates between Java and Python
The tradeoffs between recursion (elegant but potential stack overflow) and iteration (more verbose but generally safer)
The recursive solution is more elegant but might hit stack limits for very large numbers, while the iterative solution is more robust for production systems.
Subscribe to my newsletter
Read articles from Vikash Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
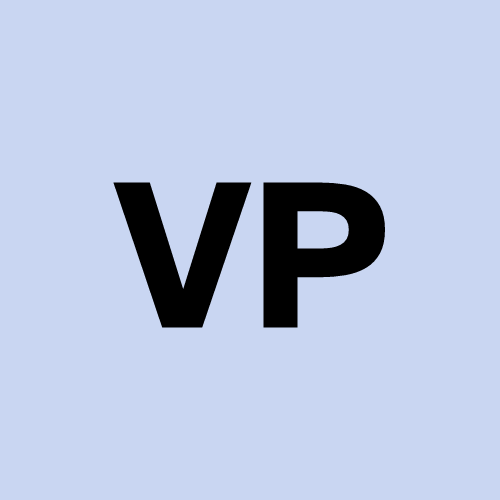